定义的抽象类进行计算
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace 老陈小石头 { public abstract class Strategy { public abstract int calculate(int a, int b); } public class AddStr : Strategy //加法 { public override int calculate(int a, int b) { return a + b; } } public class SubStr : Strategy //减法 { public override int calculate(int a, int b) { return a - b; } } public class MultiplyStr : Strategy //乘法 { public override int calculate(int a, int b) { return a * b; } } public class Environment //调用抽象类的方法 { private Strategy strategy; public Environment(Strategy str) { this.strategy = str; } public int calculate(int a, int b) { return this.strategy.calculate(a, b); } } }
Form1窗体的代码
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.IO; namespace 老陈小石头 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } string path = "I:\大二上\软件工程"; public static int count=0; public static int t = 0; public static int right = 0; public static int sum; int j = 0; int i = 0; //"*" private void cheng_Click(object sender, EventArgs e) { ysf.Text = "*"; count++; number2.Focus(); } //"+" private void jia_Click(object sender, EventArgs e) { ysf.Text = "+"; count++; number2.Focus(); } //"-" private void jian_Click(object sender, EventArgs e) { ysf.Text = "-"; count++; number2.Focus(); } //出题 private void submitan_Click(object sender, EventArgs e) { StreamWriter n1 = File.AppendText("n1.txt"); n1.WriteLine(number1.Text); n1.Close(); StreamWriter fu = File.AppendText("fu.txt"); fu.WriteLine(ysf.Text); fu.Close(); StreamWriter n2 = File.AppendText("n2.txt"); n2.WriteLine(number2.Text); n2.Close(); tiku.Text += number1.Text + ysf.Text + number2.Text + deng.Text + " "; j++; number1.Text = ""; number2.Text = ""; ysf.Text = " "; txtsave.Enabled = true; txtopen.Enabled = true; number1.Focus(); } private void timer1_Tick(object sender, EventArgs e) { t = t + 1; jitime.Text = t.ToString(); } //开始答题 private void start_Click(object sender, EventArgs e) { jitime.Text = t.ToString(); timer1.Enabled = true; timer1.Interval = 1000; timer1.Start(); string[] m1 = new string[100]; m1 = File.ReadAllLines("n1.txt"); string[] m2 = new string[100]; m2 = File.ReadAllLines("fu.txt"); string[] m3 = new string[100]; m3 = File.ReadAllLines("n2.txt"); textBox1.Text = m1[0]; textBox2.Text = m2[0]; textBox3.Text = m3[0]; answer.Focus(); } //提交答案 private void mit_Click(object sender, EventArgs e) {
int a=int.Parse(textBox1.Text); int b=int.Parse(textBox3.Text); string m = textBox2.Text; Environment envir; switch (m) { case "+": envir = new Environment(new AddStr()); sum = envir.calculate(a, b); break; case "-": envir = new Environment(new SubStr()); sum = envir.calculate(a, b); break; case "*": envir = new Environment(new MultiplyStr()); sum = envir.calculate(a, b); break; default: break; } if (answer.Text == sum.ToString()) right++; answer.Text = "";
i++; if (i < j) { string[] m1 = new string[100]; m1 = File.ReadAllLines("n1.txt"); string[] m2 = new string[100]; m2 = File.ReadAllLines("fu.txt"); string[] m3 = new string[100]; m3 = File.ReadAllLines("n2.txt"); textBox1.Text = m1[i]; textBox2.Text = m2[i]; textBox3.Text = m3[i]; } else { textBox1.Text = ""; textBox2.Text = ""; textBox3.Text = ""; } answer.Focus(); } //查看做题情况 private void button1_Click(object sender, EventArgs e) { timer1.Enabled = false; Form2 frm = new Form2(); frm.Show(); } //保存 private void txtsave_Click(object sender, EventArgs e) { SaveFileDialog TxtSaveDialog = new SaveFileDialog(); TxtSaveDialog.Filter = "RTF文件(*.RTF)|*.RTF"; if (File.Exists(path)) { this.tiku.SaveFile(path, RichTextBoxStreamType.RichText); MessageBox.Show("保存成功", "提示信息", MessageBoxButtons.OK, MessageBoxIcon.Asterisk); this.tiku.Clear(); txtsave.Enabled = false; } else { if (TxtSaveDialog.ShowDialog() == DialogResult.OK) { this.tiku.SaveFile(TxtSaveDialog.FileName, RichTextBoxStreamType.RichText); MessageBox.Show("保存成功", "提示信息", MessageBoxButtons.OK, MessageBoxIcon.Asterisk); this.tiku.Clear(); txtsave.Enabled = false; } } } //查看 private void txtopen_Click(object sender, EventArgs e) { OpenFileDialog TxTOpenDialog = new OpenFileDialog(); TxTOpenDialog.Filter = "RTF文件(*.RTF)|*.RTF"; if (TxTOpenDialog.ShowDialog() == DialogResult.OK) { path = TxTOpenDialog.FileName; this.tiku.LoadFile(TxTOpenDialog.FileName, RichTextBoxStreamType.RichText); txtsave.Enabled = false; txtopen.Enabled = false; MessageBox.Show("打开成功", "提示信息", MessageBoxButtons.OK, MessageBoxIcon.Asterisk); } } private void Form1_Load(object sender, EventArgs e) { txt tt = new txt(); tt.text1(); } } }
Form2的窗体代码
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace 老陈小石头 { public partial class Form2 : Form { public Form2() { InitializeComponent(); } private void Form2_Load(object sender, EventArgs e) {
textcount.Text = Form1.count.ToString(); Ttishu.Text = Form1.right.ToString(); Tlv.Text = ((Form1.right / (double)(Form1.count)) * 100).ToString()+"%"; yunss.Text=(Form1.t/(double)(Form1.count)).ToString()+"/t"; } } }
类txt的代码
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.IO; namespace 老陈小石头 { class txt {
//生成三个文本存放数据 public void text1() { File.WriteAllText("n1.txt", string.Empty); File.WriteAllText("fu.txt", string.Empty); File.WriteAllText("n2.txt", string.Empty); } } }
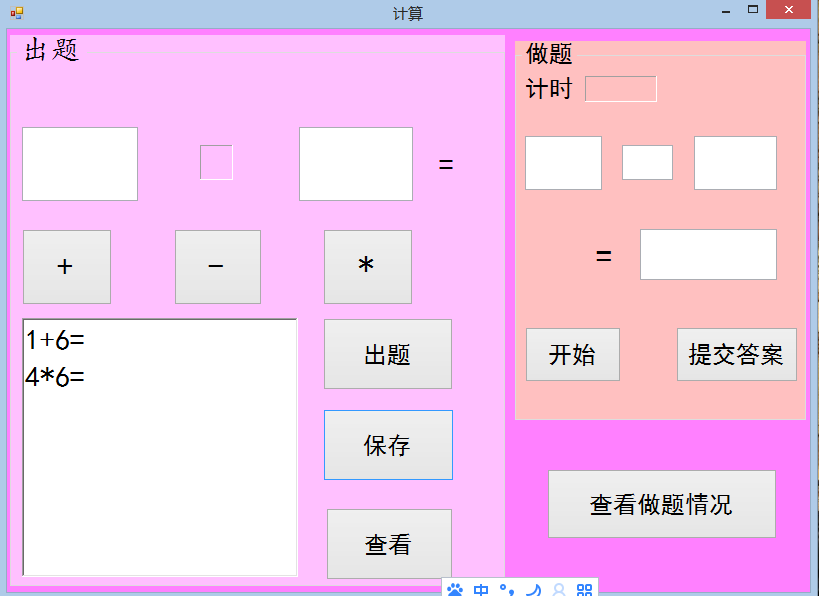

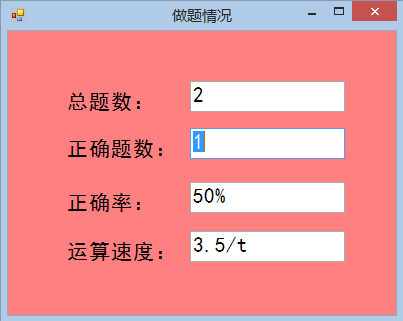