FastJson简介:
fastJson是阿里巴巴旗下的一个开源项目之一,顾名思义它专门用来做快速操作Json的序列化与反序列化的组件。它是目前json解析最快的开源组件没有之一!在这之前jaskJson是命名为快速操作json的工具,而当阿里巴巴的fastJson诞生后jaskjson就消声匿迹了,不过目前很多项目还在使用。
将fastJson加入到SpringBoot项目内,配置json返回视图使用fastJson解析。
一、项目搭建
项目搭建目录及数据库
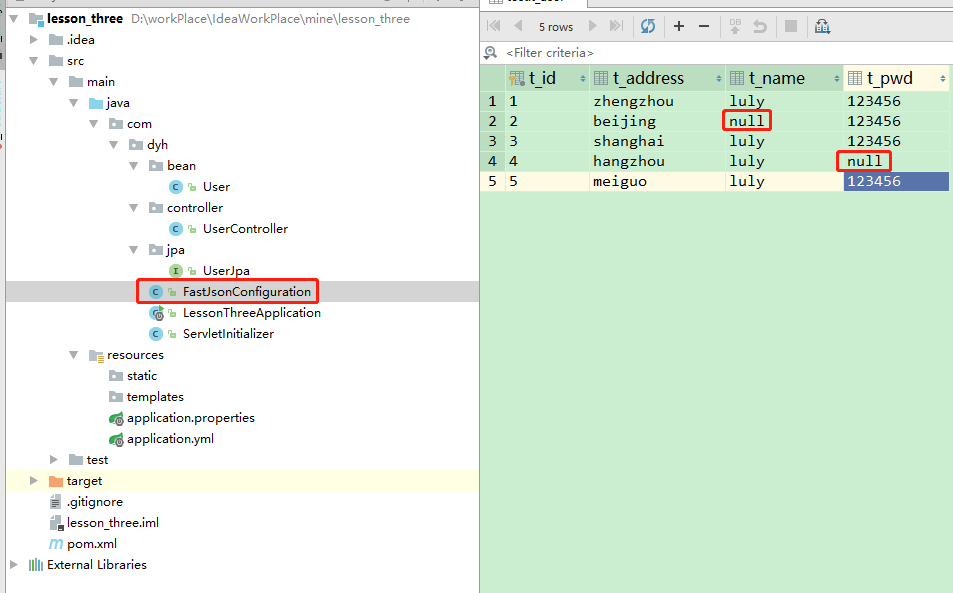
二、添加依赖(FastJson依赖)
spring-boot-stater-tomcat依赖的scope属性一定要注释掉,我们才能在IntelliJ IDEA工具使用SpringBootApplication的形式运行项目!
依赖地址:mvnrepository.com/artifact/com.alibaba/fastjson/1.2.31

<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.2.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.dyh</groupId> <artifactId>lesson_three</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>lesson_three</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <!--<scope>provided</scope>--> </dependency> <dependency> <!--fastJson--> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.51</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
三、配置文件(该项目用Spring Data JPA架构)

spring: datasource: url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT driverClassName: com.mysql.cj.jdbc.Driver username: root password: root jpa: database: MySQL show-sql: true hibernate: # naming_strategy: org.hibernate.cfg.ImprovedNamingStrategy # ddl-auto: create
四、创建一个FastJsonConfiguration配置信息类。
添加@Configuration注解让SpringBoot自动加载类内的配置,有一点要注意我们继承了WebMvcConfigurerAdapter这个类,这个类是SpringBoot内部提供专门处理用户自行添加的配置,里面不仅仅包含了修改视图的过滤还有其他很多的方法,包括我们后面章节要讲到的拦截器,过滤器,Cors配置等。
@Configuration public class FastJsonConfiguration extends WebMvcConfigurerAdapter { /** * 修改自定义消息转换器 * @param converters 消息转换器列表 */ @Override public void configureMessageConverters(List<HttpMessageConverter<?>> converters) { //调用父类的配置 super.configureMessageConverters(converters); //创建fastJson消息转换器 FastJsonHttpMessageConverter fastConverter = new FastJsonHttpMessageConverter(); //创建配置类 FastJsonConfig fastJsonConfig = new FastJsonConfig(); //修改配置返回内容的过滤 fastJsonConfig.setSerializerFeatures( SerializerFeature.DisableCircularReferenceDetect, SerializerFeature.WriteMapNullValue, SerializerFeature.WriteNullStringAsEmpty ); fastConverter.setFastJsonConfig(fastJsonConfig); //将fastjson添加到视图消息转换器列表内 converters.add(fastConverter); } }
FastJson SerializerFeatures
WriteNullListAsEmpty :List字段如果为null,输出为[],而非nullWriteNullStringAsEmpty : 字符类型字段如果为null,输出为"",而非null
DisableCircularReferenceDetect :消除对同一对象循环引用的问题,默认为false(如果不配置有可能会进入死循环)
WriteNullBooleanAsFalse:Boolean字段如果为null,输出为false,而非null
WriteMapNullValue:是否输出值为null的字段,默认为false。
五、测试,并比较用了FastJson与没有用之间的比较
使用前:

使用后:
name的值从NULL变成了"",那么证明我们的fastJson消息的转换配置完美生效了