九宫格布局,用手机输入法时经常见到。先按3行3列写。
代码的实现主要是计算插入图片的位置。
每一张图片的位置和所在的行列密切相关。分析过程如下:
界面:
代码实现
1、把需要的图片资源添加进来
然后给界面添加两个按钮,一个删除按钮,一个添加按钮。和一个lable表示图片状态。
1 // 添加按钮 2 - (UIButton *)addButtonWithImage:(NSString *)image highImage:(NSString *)highImage disableImage:(NSString *)disableImage frame:(CGRect)frame action:(SEL)action 3 { 4 // 创建按钮 5 UIButton *btn = [[UIButton alloc] init]; 6 // 设置背景图片 7 [btn setBackgroundImage:[UIImage imageNamed:image] forState:UIControlStateNormal]; 8 [btn setBackgroundImage:[UIImage imageNamed:highImage] forState:UIControlStateHighlighted]; 9 [btn setBackgroundImage:[UIImage imageNamed:disableImage] forState:UIControlStateDisabled]; 10 // 设置位置和尺寸 11 btn.frame = frame; 12 // 监听按钮点击 13 [btn addTarget:self action:action forControlEvents:UIControlEventTouchUpInside]; 14 // 添加按钮 15 [self.view addSubview:btn]; 16 return btn; 17 }
2、响应添加按钮
1 // 添加 2 - (void)add 3 { 4 // 图片索引 5 if (_ShopIndex > 5) { 6 return; 7 } 8 9 NSLog(@"添加。。。。%ld",_ShopIndex); 10 11 // 计算每次新 view 的位置 12 // 每个view 的宽度和高度 13 CGFloat viewW = 70; 14 CGFloat viewH = 110; 15 // 列数,控制显示的列数,可以修改其他值 16 NSInteger col = 3; 17 // 每个view的索引 18 NSInteger index = self.shopsView.subviews.count; 19 // 计算间隔 20 CGFloat margin = (self.shopsView.frame.size.width - col*viewW) / (col - 1); 21 // 计算xy坐标值 22 CGFloat viewX = (index % col ) * (viewW + margin); 23 CGFloat viewY = (index / col ) * (viewH + 10); 24 25 26 // 创建一个父控件显示图片和文字 27 UIView *shopView = [[UIView alloc] init]; 28 shopView.backgroundColor = [UIColor redColor]; 29 shopView.frame = CGRectMake(viewX, viewY, viewW, viewH); 30 [self.shopsView addSubview:shopView]; 31 32 33 // 添加图片 34 UIImageView *iconView = [[UIImageView alloc] init]; 35 36 iconView.image = [UIImage imageNamed:_shops[_ShopIndex][@"icon"]]; 37 iconView.frame = CGRectMake(0, 0, viewW, viewH - 20); 38 iconView.backgroundColor = [UIColor blueColor]; 39 [shopView addSubview:iconView]; 40 41 // 添加文字 42 UILabel *label = [[UILabel alloc] init]; 43 label.text = _shops[_ShopIndex][@"name"]; 44 label.frame = CGRectMake(0,90, viewW, 20); 45 label.font = [UIFont systemFontOfSize:11]; 46 label.backgroundColor = [UIColor greenColor]; 47 label.textAlignment = NSTextAlignmentCenter; 48 [shopView addSubview:label]; 49 // 索引自增 50 _ShopIndex ++; 51 [self checkBtn]; 52 53 }
3、响应删除按钮
1 // 删除 2 - (void)remove 3 { 4 // 图片索引 5 _ShopIndex --; 6 [self checkBtn]; 7 8 if(_ShopIndex < 0) 9 { 10 _ShopIndex = 0; 11 return ; 12 } 13 14 NSLog(@"删除。。。。%ld",_ShopIndex); 15 // 删除子控件 16 [[self.shopsView.subviews lastObject] removeFromSuperview]; 17 }
4、检查按钮状态
如果图片添加完毕,则添加按钮失效,如果一张图片也没有,那么删除按钮失效。
1 // 判断按钮状态, 2 - (void)checkBtn 3 { 4 // 添加按钮的状态 5 self.addBtn.enabled = (self.shopsView.subviews.count < _shops.count); 6 // 删除按钮的状态 7 self.removeBtn.enabled = (self.shopsView.subviews.count > 0); 8 // 删除完毕 9 if(self.removeBtn.enabled == NO ) 10 { 11 self.hudLable.hidden = NO; 12 self.hudLable.text = @"商品已经删除完毕!!"; 13 [self performSelector:@selector(hideHUD) withObject:nil afterDelay:2.0]; 14 } 15 // 添加完毕 16 else if (self.addBtn.enabled == NO && _ShopIndex == 6) 17 { 18 self.hudLable.hidden = NO; 19 self.hudLable.text = @"商品已经添加完毕!!"; 20 } 21 // 计时器3 22 [NSTimer scheduledTimerWithTimeInterval:3.0 target:self selector:@selector(hideHUD) userInfo:nil repeats:NO]; 23 }
5、隐藏提示信息
- (void)hideHUD
{
// 隐藏 lable
_hudLable.hidden = YES;
}
效果如下:
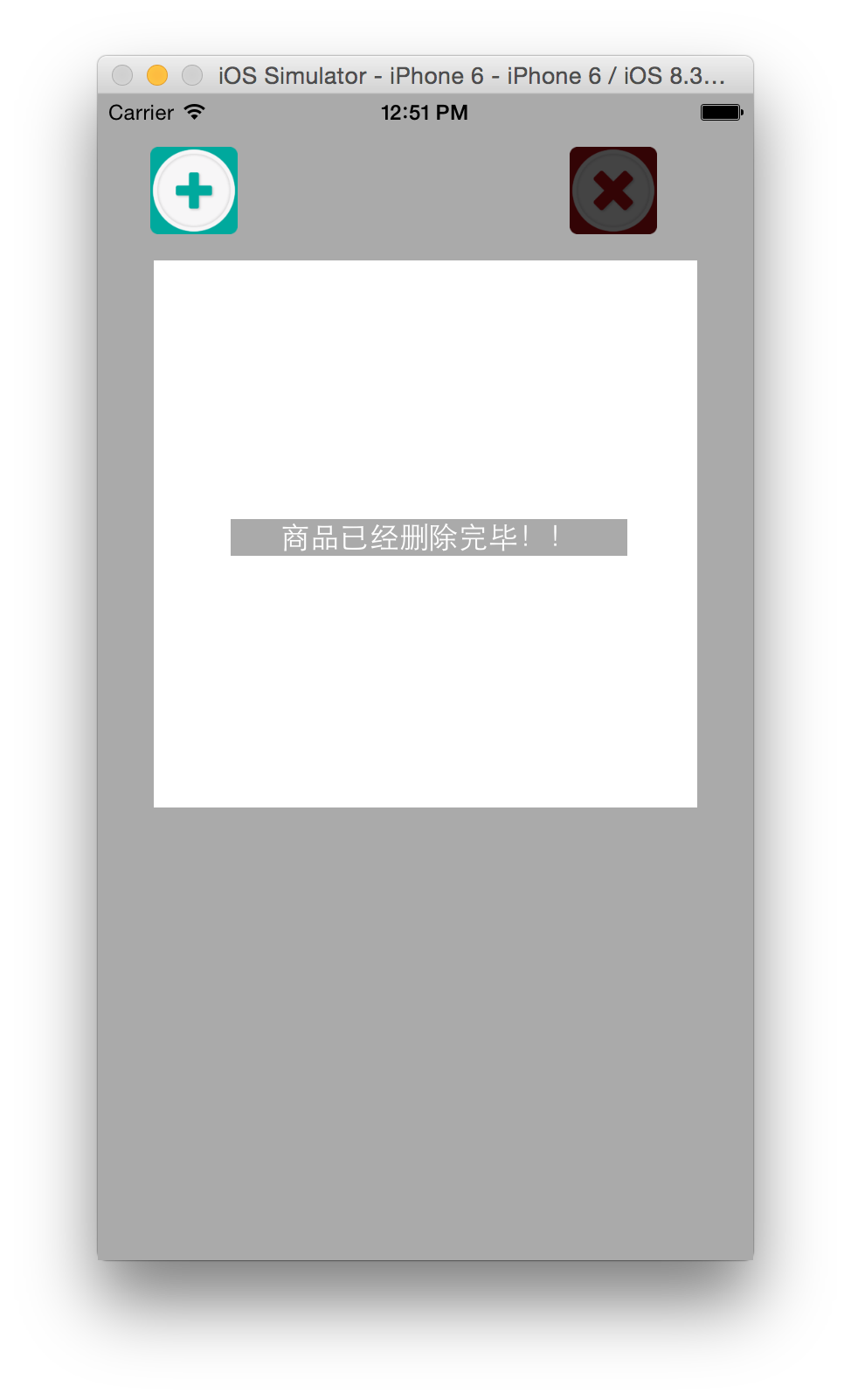
在add方法中可以修改要显示的列数,来显示多列。
