概要:
当java类中含有集合属性:如List、Set、Map、Pros等时,Spring配置文件中该如何配置呢?
下面将进行讲解。
整体结构:
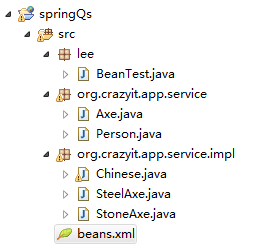
接口
Axe.java
package org.crazyit.app.service;
public interface Axe
{
public String chop();
}
Person.java
package org.crazyit.app.service;
public interface Person
{
public void test();
}
实现类
Chinese.java
package org.crazyit.app.service.impl;
import java.util.*;
import org.crazyit.app.service.*;
public class Chinese implements Person
{
// 下面是系列集合类型的成员变量
private List<String> schools;
private Map scores;
private Map<String , Axe> phaseAxes;
private Properties health;
private Set axes;
private String[] books;
public Chinese()
{
System.out.println("Spring实例化主调bean:Chinese实例...");
}
// schools的setter方法
public void setSchools(List schools)
{
this.schools = schools;
}
// scores的setter方法
public void setScores(Map scores)
{
this.scores = scores;
}
// phaseAxes的setter方法
public void setPhaseAxes(Map<String , Axe> phaseAxes)
{
this.phaseAxes = phaseAxes;
}
// health的setter方法
public void setHealth(Properties health)
{
this.health = health;
}
// axes的setter方法
public void setAxes(Set axes)
{
this.axes = axes;
}
// books的setter方法
public void setBooks(String[] books)
{
this.books = books;
}
// 访问上面全部的集合类型的成员变量
public void test()
{
System.out.println(schools);
System.out.println(scores);
System.out.println(phaseAxes);
System.out.println(health);
System.out.println(axes);
System.out.println(java.util.Arrays.toString(books));
}
}
SteelAxe.java
package org.crazyit.app.service.impl;
import org.crazyit.app.service.*;
public class SteelAxe implements Axe
{
public String chop()
{
return "钢斧砍柴真快";
}
}
StoneAxe.java
package org.crazyit.app.service.impl;
import org.crazyit.app.service.*;
public class StoneAxe implements Axe
{
public String chop()
{
return "石斧砍柴好慢";
}
}
配置文件:
beans.xml
<?xml version="1.0" encoding="GBK"?>
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://www.springframework.org/schema/beans"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd">
<!-- 定义2个普通Axe Bean -->
<bean id="stoneAxe" class="org.crazyit.app.service.impl.StoneAxe"/>
<bean id="steelAxe" class="org.crazyit.app.service.impl.SteelAxe"/>
<!-- 定义chinese Bean -->
<bean id="chinese" class="org.crazyit.app.service.impl.Chinese">
<property name="schools">
<!-- 为调用setSchools()方法配置List集合作为参数值 -->
<list>
<!-- 每个value、ref、bean...都配置一个List元素 -->
<value>小学</value>
<value>中学</value>
<value>大学</value>
</list>
</property>
<property name="scores">
<!-- 为调用setScores()方法配置Map集合作为参数值 -->
<map>
<!-- 每个entry配置一个key-value对 -->
<entry key="数学" value="87"/>
<entry key="英语" value="89"/>
<entry key="语文" value="82"/>
</map>
</property>
<property name="phaseAxes">
<!-- 为调用setPhaseAxes()方法配置Map集合作为参数值 -->
<map>
<!-- 每个entry配置一个key-value对 -->
<entry key="原始社会" value-ref="stoneAxe"/>
<entry key="农业社会" value-ref="steelAxe"/>
</map>
</property>
<property name="health">
<!-- 为调用setHealth()方法配置Properties集合作为参数值 -->
<props>
<!-- 每个prop元素配置一个属性项,其中key指定属性名 -->
<prop key="血压">正常</prop>
<prop key="身高">175</prop>
</props>
<!--
<value>
pressure=normal
height=175
</value> -->
</property>
<property name="axes">
<!-- 为调用setAxes()方法配置Set集合作为参数值 -->
<set>
<!-- 每个value、ref、bean..都配置一个Set元素 -->
<value>普通的字符串</value>
<bean class="org.crazyit.app.service.impl.SteelAxe"/>
<ref bean="stoneAxe"/>
<!-- 为Set集合配置一个List集合作为元素 -->
<list>
<value>20</value>
<!-- 再次为List集合配置一个Set集合作为元素 -->
<set>
<value type="int">30</value>
</set>
</list>
</set>
</property>
<property name="books">
<!-- 为调用setBooks()方法配置数组作为参数值 -->
<list>
<!-- 每个value、ref、bean...都配置一个数组元素 -->
<value>疯狂Java讲义</value>
<value>疯狂Android讲义</value>
<value>轻量级Java EE企业应用实战</value>
</list>
</property>
</bean>
</beans>
测试文件:
package lee;
import org.springframework.context.*;
import org.springframework.context.support.*;
import org.crazyit.app.service.*;
public class BeanTest
{
public static void main(String[] args)throws Exception
{
ApplicationContext ctx = new
ClassPathXmlApplicationContext("beans.xml");
// 获取容器中Bean,并调用方法。
Person p = ctx.getBean("chinese" , Person.class);
p.test();
}
}
运行结果:
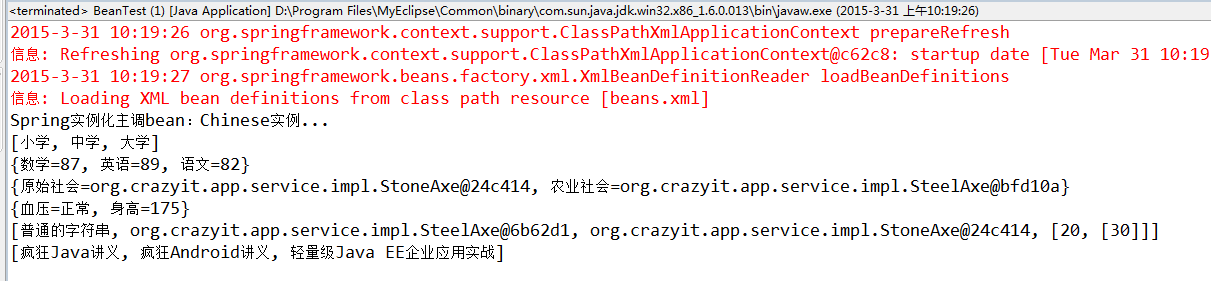
Spring实例化主调bean:Chinese实例...
[小学, 中学, 大学]
{数学=87, 英语=89, 语文=82}
{原始社会=org.crazyit.app.service.impl.StoneAxe@24c414, 农业社会=org.crazyit.app.service.impl.SteelAxe@bfd10a}
{血压=正常, 身高=175}
[普通的字符串, org.crazyit.app.service.impl.SteelAxe@6b62d1, org.crazyit.app.service.impl.StoneAxe@24c414, [20, [30]]]
[疯狂Java讲义, 疯狂Android讲义, 轻量级Java EE企业应用实战]