Nearest Common Ancestors
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 30147 | Accepted: 15413 |
Description
A rooted tree is a well-known data structure in computer science and engineering. An example is shown below:
In the figure, each node is labeled with an integer from {1, 2,...,16}. Node 8 is the root of the tree. Node x is an ancestor of node y if node x is in the path between the root and node y. For example, node 4 is an ancestor of node 16. Node 10 is also an ancestor of node 16. As a matter of fact, nodes 8, 4, 10, and 16 are the ancestors of node 16. Remember that a node is an ancestor of itself. Nodes 8, 4, 6, and 7 are the ancestors of node 7. A node x is called a common ancestor of two different nodes y and z if node x is an ancestor of node y and an ancestor of node z. Thus, nodes 8 and 4 are the common ancestors of nodes 16 and 7. A node x is called the nearest common ancestor of nodes y and z if x is a common ancestor of y and z and nearest to y and z among their common ancestors. Hence, the nearest common ancestor of nodes 16 and 7 is node 4. Node 4 is nearer to nodes 16 and 7 than node 8 is.
For other examples, the nearest common ancestor of nodes 2 and 3 is node 10, the nearest common ancestor of nodes 6 and 13 is node 8, and the nearest common ancestor of nodes 4 and 12 is node 4. In the last example, if y is an ancestor of z, then the nearest common ancestor of y and z is y.
Write a program that finds the nearest common ancestor of two distinct nodes in a tree.
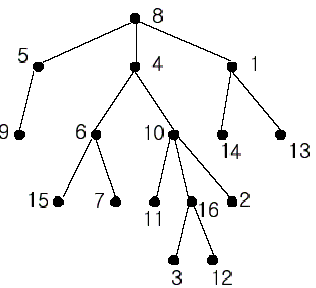
In the figure, each node is labeled with an integer from {1, 2,...,16}. Node 8 is the root of the tree. Node x is an ancestor of node y if node x is in the path between the root and node y. For example, node 4 is an ancestor of node 16. Node 10 is also an ancestor of node 16. As a matter of fact, nodes 8, 4, 10, and 16 are the ancestors of node 16. Remember that a node is an ancestor of itself. Nodes 8, 4, 6, and 7 are the ancestors of node 7. A node x is called a common ancestor of two different nodes y and z if node x is an ancestor of node y and an ancestor of node z. Thus, nodes 8 and 4 are the common ancestors of nodes 16 and 7. A node x is called the nearest common ancestor of nodes y and z if x is a common ancestor of y and z and nearest to y and z among their common ancestors. Hence, the nearest common ancestor of nodes 16 and 7 is node 4. Node 4 is nearer to nodes 16 and 7 than node 8 is.
For other examples, the nearest common ancestor of nodes 2 and 3 is node 10, the nearest common ancestor of nodes 6 and 13 is node 8, and the nearest common ancestor of nodes 4 and 12 is node 4. In the last example, if y is an ancestor of z, then the nearest common ancestor of y and z is y.
Write a program that finds the nearest common ancestor of two distinct nodes in a tree.
Input
The input consists of T test cases. The number of test cases (T) is given in the first line of the input file. Each test case starts with a line containing an integer N , the number of nodes in a tree, 2<=N<=10,000. The nodes are labeled with integers 1, 2,..., N. Each of the next N -1 lines contains a pair of integers that represent an edge --the first integer is the parent node of the second integer. Note that a tree with N nodes has exactly N - 1 edges. The last line of each test case contains two distinct integers whose nearest common ancestor is to be computed.
Output
Print exactly one line for each test case. The line should contain the integer that is the nearest common ancestor.
Sample Input
2 16 1 14 8 5 10 16 5 9 4 6 8 4 4 10 1 13 6 15 10 11 6 7 10 2 16 3 8 1 16 12 16 7 5 2 3 3 4 3 1 1 5 3 5
Sample Output
4 3
Source
题目大意:
输入T组数据,
然后输入n个节点,
紧接着输入n-1条边 x y,表示x是y的父亲节点
最后输入要询问的 s,t
求s t的最近公共祖先
题解:LCA
#include<iostream> #include<vector> #include<cstdio> #include<cmath> #include<algorithm> using namespace std; const int N=10005; const int logN=20; vector<int> G[N]; int root; int parent[21][N]; int fa[N],n,x,y,s,t; int depth[N]; void dfs(int v,int p,int d) { parent[0][v]=p; depth[v]=d; for(int i=0;i<G[v].size();i++) if (G[v][i]!=p) { fa[G[v][i]]=v; dfs(G[v][i],v,d+1); } } void init(int V) { int root; for(int i=1;i<=n;i++) if (fa[i]==0) {root=i; break;} dfs(root,-1,0); for(int k=0;k+1<logN;k++) { for(int v=1;v<=V;v++) { if(parent[k][v]<0) parent[k+1][v]=-1; else parent[k+1][v]=parent[k][parent[k][v]]; } } } int lca(int u,int v) { if (depth[u]>depth[v]) swap(u,v); for(int k=0;k<logN;k++) { if ((depth[v]-depth[u])>>k & 1) v=parent[k][v]; } if (u==v) return u; for(int k=logN-1;k>=0;k--) { if (parent[k][u]!=parent[k][v]) { u=parent[k][u]; v=parent[k][v]; } } return parent[0][u]; } int main() { int T; scanf("%d",&T); while(T--) { scanf("%d",&n); for(int i=1;i<=n;i++) {G[i].clear(); fa[i]=0;} for(int i=1;i<=n-1;i++) { scanf("%d%d",&x,&y); G[x].push_back(y); fa[y]=x; } init(n); scanf("%d%d",&s,&t); int croot=lca(s,t); printf("%d ",croot); } return 0; }
ST&RMQ 的LCA
#include<iostream> #include<vector> #include<cstdio> #include<cmath> #include<algorithm> #include<cstring> using namespace std; const int N=10005; const int M=25; int tot,cnt,n,T,s,t; int head[N]; //记录i节点在e数组中的其实位置 int ver[2*N]; //ver:保存遍历的节点序列,长度为2n-1,从下标1开始保存 int R[2*N]; // R:和遍历序列对应的节点深度数组,长度为2n-1,从下标1开始保存 int first[N]; //first:每个节点在遍历序列中第一次出现的位置 bool vis[N]; //遍历时的标记数组 int dp[2*N][M],fa[N]; struct edge { int u,v,next; }e[2*N]; void dfs(int u ,int dep) { vis[u] = true; ver[++tot] = u; first[u] = tot; R[tot] = dep; for(int k=head[u]; k!=-1; k=e[k].next) if( !vis[e[k].v] ) //这里可以省个vis数组,如果在dfs改成dfs(当前节点,当前节点的父亲,当前节点的深度) 具体可以参照connections with cities { int v=e[k].v; dfs(v,dep+1); ver[++tot]=u; R[tot]=dep; } } void ST(int n) { for(int i=1;i<=n;i++) dp[i][0] = i; for(int j=1;(1<<j)<=n;j++) { for(int i=1;i+(1<<j)-1<=n;i++) { int a = dp[i][j-1] , b = dp[i+(1<<(j-1))][j-1]; dp[i][j] = R[a]<R[b]?a:b; } } } int RMQ(int l,int r) { int k=(int)(log((double)(r-l+1))/log(2.0)); int a = dp[l][k], b = dp[r-(1<<k)+1][k]; //保存的是编号 return R[a]<R[b]?a:b; } int LCA(int u ,int v) { int x = first[u] , y = first[v]; if(x > y) swap(x,y); int res = RMQ(x,y); return ver[res]; } void addedge(int u,int v) { e[++cnt].u=u; e[cnt].v=v; e[cnt].next=head[u]; head[u]=cnt; } int main() { int T; scanf("%d",&T); while(T--) { scanf("%d",&n); memset(head,-1,sizeof(head)); memset(vis,0,sizeof(vis)); memset(fa,0,sizeof(fa)); cnt=0; tot=0; for(int i=1;i<n;i++) { int x,y; scanf("%d%d",&x,&y); addedge(x,y); fa[y]=x; } for(int i=1;i<=n;i++) if (fa[i]==0) { dfs(i,1); break;} ST(2*n-1); scanf("%d%d",&s,&t); printf("%d ",LCA(s,t)); } return 0; }