添加约束的方式:
1.通过使用NSLayoutConstraints添加约束到约束数组中,之前必须设置translatesAutoresizingMaskIntoConstraints = NO,即取消自动布局;
2.通过使用MASConstraintMaker在block中添加约束,不需要再设置translatesAutoresizingMaskIntoConstraintst 属性,block内部已经帮助完成;
约束的关系:
equalTo
<=======> NSLayoutRelationEqual 等于
lessThanOrEqualTo
<======> NSLayoutRelationLessThanOrEqual 小于或等于
greaterThanOrEqualTo
<=======> NSLayoutRelationGreaterThanOrEqual 大于或等于
MASViewAttribute:视图约束属性
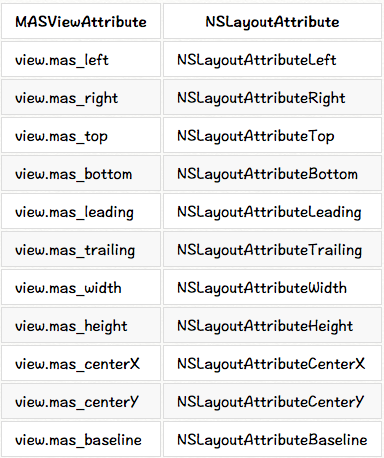
UIView/NSView
这两个约束完全相同,都是view左边大于等于label的左边位置
make.left.greaterThanOrEqualTo(label);
make.left.greaterThanOrEqualTo(label.mas_left);
NSNumber给约束设置具体的值
<1>//width >= 200 && width <= 400
make.width.greaterThanOrEqualTo(@200); make.width.lessThanOrEqualTo(@400)
<2>//creates view.left = view.superview.left + 10
make.left.lessThanOrEqualTo(@10)
代替NSNumber,使用原始的数据或者结构体设置约束数据
make.top.mas_equalTo(42); make.height.mas_equalTo(20); make.size.mas_equalTo(CGSizeMake(50, 100)); make.edges.mas_equalTo(UIEdgeInsetsMake(10, 0, 10, 0)); make.left.mas_equalTo(view).mas_offset(UIEdgeInsetsMake(10, 0, 10, 0));
使用数组NSArray设置约束
make.height.equalTo(@[view1.mas_height, view2.mas_height]); make.height.equalTo(@[view1, view2]); make.left.equalTo(@[view1, @100, view3.right]);
使用优先级设置约束
.priorityHigh
<======> UILayoutPriorityDefaultHigh 高优先级
.priorityMedium
<========> between high and low 介于高/低之间
.priorityLow
<=========> UILayoutPriorityDefaultLow 低优先级
make.left.greaterThanOrEqualTo(label.mas_left).with.priorityLow(); make.top.equalTo(label.mas_top).with.priority(600);
使用MASCompositeConstraints添加约束
edges:边缘
// make top, left, bottom, right equal view2 make.edges.equalTo(view2); // make top = superview.top + 5, left = superview.left + 10, // bottom = superview.bottom - 15, right = superview.right - 20 make.edges.equalTo(superview).insets(UIEdgeInsetsMake(5, 10, 15, 20))
// All edges but the top should equal those of the superview make.left.right.and.bottom.equalTo(superview); make.top.equalTo(otherView);
size:大小
// make width and height greater than or equal to titleLabel make.size.greaterThanOrEqualTo(titleLabel) // make width = superview.width + 100, height = superview.height - 50 make.size.equalTo(superview).sizeOffset(CGSizeMake(100, -50))
center:中心
// make centerX and centerY = button1 make.center.equalTo(button1) // make centerX = superview.centerX - 5, centerY = superview.centerY + 10 make.center.equalTo(superview).centerOffset(CGPointMake(-5, 10))
有时候,你需要修改现有的约束,以动画或删除/替换约束。在砌体中有几个不同的方法来更新约束。
1.使用设置References
// in public/private interface @property (nonatomic, strong) MASConstraint *topConstraint; ... // when making constraints [view1 mas_makeConstraints:^(MASConstraintMaker *make) { self.topConstraint = make.top.equalTo(superview.mas_top).with.offset(padding.top); make.left.equalTo(superview.mas_left).with.offset(padding.left); }]; ... // then later you can call [self.topConstraint uninstall];
2.更新约束 mas_updateConstraints
- (void)updateConstraints { [self.growingButton mas_updateConstraints:^(MASConstraintMaker *make) { make.center.equalTo(self); make.width.equalTo(@(self.buttonSize.width)).priorityLow(); make.height.equalTo(@(self.buttonSize.height)).priorityLow(); make.width.lessThanOrEqualTo(self); make.height.lessThanOrEqualTo(self); }]; //according to apple super should be called at end of method [super updateConstraints]; }
3.重新设置mas_remakeConstraints
- (void)changeButtonPosition {
[self.button mas_remakeConstraints:^(MASConstraintMaker *make) { make.size.equalTo(self.buttonSize); if (topLeft) { make.top.and.left.offset(10); } else { make.bottom.and.right.offset(-10); } }]; }
具体的实例如下:设置view1举例父视图的四周距离均为50
方式一:
/** 方式一:使用NSLayoutConstraint实现手动布局 ---------------------------- 设置UIEdgeInsets ---------------------------- @interface UIView (UIConstraintBasedLayoutInstallingConstraints) - (NSArray *)constraints NS_AVAILABLE_IOS(6_0); - (void)addConstraint:(NSLayoutConstraint *)constraint NS_AVAILABLE_IOS(6_0); - (void)addConstraints:(NSArray *)constraints NS_AVAILABLE_IOS(6_0); - (void)removeConstraint:(NSLayoutConstraint *)constraint - (void)removeConstraints:(NSArray *)constraints NS_AVAILABLE_IOS(6_0); */ -(void)LayoutConstraint { UIView *superView = self.view; UIView *view1 = [[UIView alloc]init]; view1 .translatesAutoresizingMaskIntoConstraints = NO; view1.backgroundColor = [UIColor redColor]; [superView addSubview:view1]; //设置距离父视图边界距离 UIEdgeInsets pading = UIEdgeInsetsMake(50, 50, 50, 50); //添加给view1约束 [superView addConstraints:@[ [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:superView attribute:NSLayoutAttributeTop multiplier:1.0 constant:pading.top], [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:superView attribute:NSLayoutAttributeLeft multiplier:1.0 constant:pading.left], [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeBottom relatedBy:NSLayoutRelationEqual toItem:superView attribute:NSLayoutAttributeBottom multiplier:1.0 constant:-pading.bottom], [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:superView attribute:NSLayoutAttributeRight multiplier:1.0 constant:-pading.right], ]]; }
方式二:
/** 方法二:使用block @implementation MAS_VIEW (MASAdditions) ---------------------------- 设置offset偏移 或者 边缘edges ---------------------------- - (NSArray *)mas_makeConstraints:(void(^)(MASConstraintMaker *))block - (NSArray *)mas_updateConstraints:(void(^)(MASConstraintMaker *))block - (NSArray *)mas_remakeConstraints:(void(^)(MASConstraintMaker *make))block */ -(void)LayoutForMASConstraintMaker { UIView *superView = self.view; UIView *view1 = [[UIView alloc]init]; view1.backgroundColor = [UIColor redColor]; [superView addSubview:view1]; //设置距离父视图边界距离 UIEdgeInsets pading = UIEdgeInsetsMake(50, 50, 50, 50); //添加给view1约束 [view1 mas_makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(superView.mas_top).with.offset(pading.top); make.left.equalTo(superView.mas_left).with.offset(pading.left); make.bottom.equalTo(superView.mas_bottom).with.offset(-pading.bottom); make.right.equalTo(superView.mas_right).with.offset(-pading.right); //设置代码可以更简单(效果与上面的是一样的) //make.edges.equalTo(superView).with.insets(pading); }]; }
方式三:
/** 方式三:使用block @implementation MAS_VIEW (MASAdditions) ---------------------------- 设置margin距离 ---------------------------- - (NSArray *)mas_makeConstraints:(void(^)(MASConstraintMaker *))block - (NSArray *)mas_updateConstraints:(void(^)(MASConstraintMaker *))block - (NSArray *)mas_remakeConstraints:(void(^)(MASConstraintMaker *make))block */ -(void)LayoutForMASConstraintMakerWithMargin { UIView *superView = self.view; UIView *view1 = [[UIView alloc]init]; view1.backgroundColor = [UIColor redColor]; [superView addSubview:view1]; //添加给view1约束 [view1 mas_makeConstraints:^(MASConstraintMaker *make) { make.topMargin.equalTo(superView.mas_top).with.offset(50); make.leftMargin.equalTo(superView.mas_left).with.offset(50); make.bottomMargin.equalTo(superView.mas_bottom).with.offset(-50); make.rightMargin.equalTo(superView.mas_right).with.offset(-50); }]; }
方式四:
/** 方式四:使用block @implementation MAS_VIEW (MASAdditions) ---------------------------- 设置center和size ---------------------------- - (NSArray *)mas_makeConstraints:(void(^)(MASConstraintMaker *))block - (NSArray *)mas_updateConstraints:(void(^)(MASConstraintMaker *))block - (NSArray *)mas_remakeConstraints:(void(^)(MASConstraintMaker *make))block */ -(void)LayoutForMASConstraintMakerWithCenterWidthHeight { UIView *superView = self.view; UIView *view1 = [[UIView alloc]init]; view1.backgroundColor = [UIColor redColor]; [superView addSubview:view1]; //添加给view1约束 [view1 mas_makeConstraints:^(MASConstraintMaker *make) { make.centerX.equalTo(superView); make.centerY.equalTo(superView); make.size.equalTo(superView).sizeOffset(CGSizeMake(-100,-100)); }]; }
演示结果:
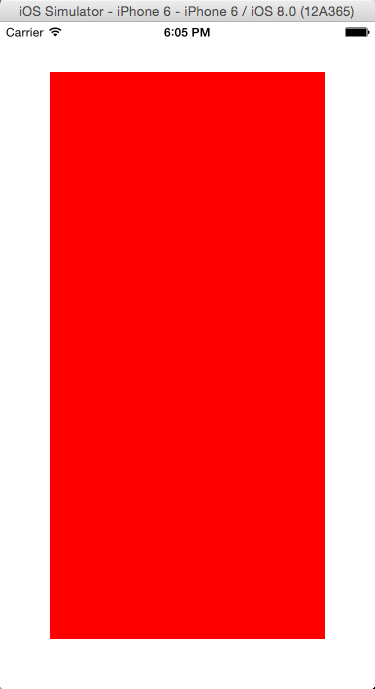
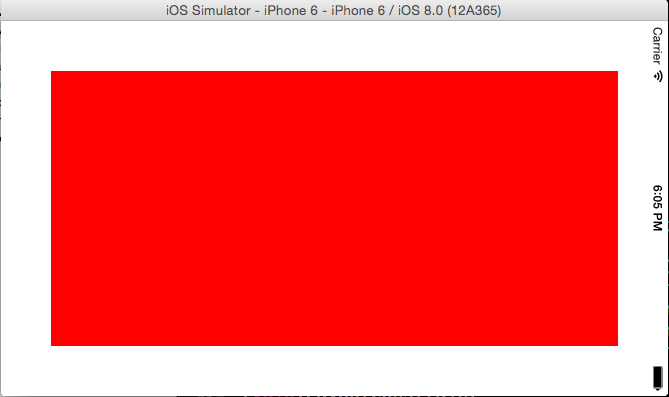
程序猿神奇的手,每时每刻,这双手都在改变着世界的交互方式!