素数筛:需要一个数组进行标记
最小的素数2,所有是2的倍数的数都是合数,对合数进行标记,然后找大于2的第一个非标记的数(肯定是素数),将其倍数进行标记,如此反复,若是找n以内的所有素数,只需要对[2,n^0.5]进行循环即可,因为n以内的所有数如果不是[2,n^0.5]的倍数,则一定是素数。复杂度:O(nloglogn);
for(int i=2;i*i<=n;i++){
if(a[i]!=-1)
for(int k=i*i;k<=n;k+=i)
a[i]=-1;
}
poj3126题目链接:http://poj.org/problem?id=3126
素数筛所占用的空间较大,毕竟是需要数组进行标记的,如果n太大就不可以做出(内存超出)。
若是让求[a,b]内的素数,a<b<=1e12,b-a<=1e6; 这个如果直接用素数筛是内存超出,可以先用一个数组存储[0,b^0.5]的素数,再用这些素数筛选[a,b],注意令一个数组表示[a,b]的素数,不能让下标直接表示这个数字(>=a&&<=b),而是开一个数组,c[i]表示a+i是否是素数。
Description
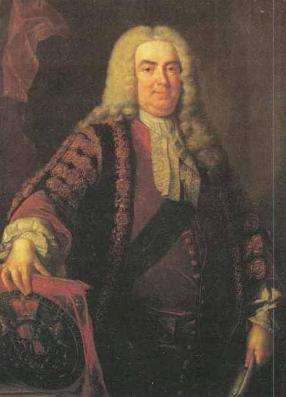
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
1733
3733
3739
3779
8779
8179
Input
Output
Sample Input
3 1033 8179 1373 8017 1033 1033
Sample Output
6 7 0
现附上AC代码:
#include<iostream>
#include<cstdio>
#include<queue>
#include<cmath>
using namespace std;
const int maxn=1e4+5;
int main(){
int T,num[4],temp;cin>>T;
int plu[maxn];
while(T--){
for(int i=0;i<=maxn;i++)
plu[i]=0;
for(int i=2;i*i<=maxn;i++){
for(int j=i*i;j<=maxn;j+=i){
plu[j]=-1;
}
}
int x,y;scanf("%d%d",&x,&y);
queue<int >q;
q.push(x);
plu[x]=1;//注意这一步的重要性,没有这一步的话,可能会导致 plu[temp]=plu[t]+1;当plu[t]==0时就执行了,
while(!q.empty()&&plu[y]==0){
int m=q.front(),t=q.front(); q.pop();
for(int i=3;i>=0;i--)
num[i]=m%10,m/=10;
for(int j=0;j<4;j++){
int mm=num[j],i;
if(j==0) i=1;
else i=0;
for(;i<=9;i++){
num[j]=i;
temp=num[0]*1000+num[1]*100+num[2]*10+num[3];
if(plu[temp]==0) {
plu[temp]=plu[t]+1;
q.push(temp);
}
}
num[j]=mm;
}
}
// printf("%d %d %d %d %d %d %d
",plu[1033],plu[1733],plu[3733],plu[3739],plu[3779],plu[8779],plu[8179]);
if(plu[y]==-1) printf("Impossible
");
else printf("%d
",plu[y]-1);
}
return 0;
}
这道题是要求最小步骤到达目标,就是一个变形的最短路问题。对于最短路问题,肯定是首选BFS,而我们首先对x进行,个位十位百位千位的遍历,若新生成的数是素数(且之前没有访问过,毕竟是求最短路,若之前已经到过,则再到肯定不是最短,便剪枝),则将其放进队列。最后得出plu[y]基本就可以知道答案了。
回过头来一想,发现的确是很简单,但之前却wrong answer了很多次,自己都对自己无语了。