日常工作中,主要是通过ssh终端(putty等)远程开发,经常涉及到传输文件,因为本地系统为Win10,所以没办法利用强大的linux脚本来进行文件传输。之前用过python的SimpleHttp模块写了一个简单的文件服务器(http://www.cnblogs.com/sxhlinux/p/6694904.html),但是缺少GUI,且仍然依赖curl等相关命令。所以,就想着写一个简单的http文件服务器,满足普通的文件上传、下载文件。
代码采用python 3.4版本,结构如下
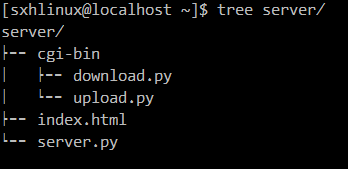
代码如下
#### server.py ####
#!/usr/bin/python3 import sys from http.server import BaseHTTPRequestHandler, HTTPServer, CGIHTTPRequestHandler if __name__ == '__main__': try: handler = CGIHTTPRequestHandler handler.cgi_directories = ['/cgi-bin', '/htbin'] port = int(sys.argv[1]) print('port is %d'% port) server = HTTPServer(('', port), handler) print('Welcome to my website !') server.serve_forever() except KeyboardInterrupt: print ('^C received, shutting down server') server.socket.close()
#### index.html ####
<!DOCTYPE html> <html> <meta charset="utf-8"> <head> <title>File Server</title> </head> <body> <form action="/cgi-bin/download.py" method="get"> 要下载的文件: <input type="text" name="filename" /> <input type="submit" value="download"> </form> <form enctype="multipart/form-data" action="/cgi-bin/upload.py" method="post"> 要上传的文件: <input type="file" name="filename" /> <input type="submit" value="upload"> </form> </body> </html>
#### download.py ####
#!/usr/bin/python3 import os import sys import cgi form = cgi.FieldStorage() filename = form.getvalue('filename') dir_path = "/home/sxhlinux/data/" target_path = dir_path + str(filename) if os.path.exists(target_path) == True: print ('Content-Type: application/octet-stream') print ('Content-Disposition: attachment; filename = "%s"' % filename) sys.stdout.flush() fo = open(target_path, "rb") sys.stdout.buffer.write(fo.read()) fo.close() else: print(""" Content-type: text/html <html> <head> <meta charset="utf-8"> <title>File server</title> </head> <body> <h1> %s doesn't exist in the server: files in the server list below: </h1>""" % filename) for line in os.popen("ls -lh ~/data/"): name = line.strip().split(' ', 8) if len(name) == 9: print('''<form action="/cgi-bin/download.py" method="get"> %s <input type="submit" name="filename" value="%s"> </form>''' % (line, name[8])) print('</body> <html>')
#### upload.py ####
#!/usr/bin/python3 import cgi, os form = cgi.FieldStorage() item = form['filename'] if item.filename: fn = os.path.basename(item.filename) open('/home/sxhlinux/data/' + fn, 'wb').write(item.file.read()) msg = 'File ' + fn + ' upload successfully !' else: msg = 'no file is uploaded ' print(""" Content-type: text/html <html> <head> <meta charset="utf-8"> <title>Hello world</title> </head> <body> <h2>名称: %s</h2> </body> <html> """ % (msg,))
将上述代码按照前面的目录结构放好,然后执行命令 nohup /usr/bin/python3 server.py 8001 >/dev/null & 启动文件服务端,监听8001端口,远程可以在浏览器中 输入 http://SERVER_ADDR:8001 来访问该文件服务器进行相关的文件上传下载操作。
注:附件中是整个demo的代码(download.py,upload.py两个文件中的目录路径 /home/sxhlinux/data 需要改成自己要存储文件的文件夹路径),有兴趣的小伙伴可以下载修改,刚学习python,欢迎大家多多提意见。