Stars
Description
Astronomers often examine star maps where stars are represented by points on a plane and each star has Cartesian coordinates. Let the level of a star be an amount of the stars that are not higher and not to the right of the given star. Astronomers want to know the distribution of the levels of the stars.
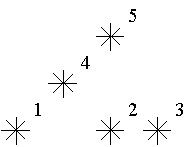
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
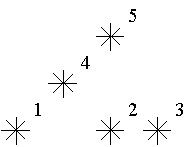
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
The first line of the input file contains a number of stars N (1<=N<=15000). The following N lines describe coordinates of stars (two integers X and Y per line separated by a space, 0<=X,Y<=32000). There can be only one star at one point of the plane. Stars are listed in ascending order of Y coordinate. Stars with equal Y coordinates are listed in ascending order of X coordinate.
Output
The output should contain N lines, one number per line. The first line contains amount of stars of the level 0, the second does amount of stars of the level 1 and so on, the last line contains amount of stars of the level N-1.
Sample Input
5 1 1 5 1 7 1 3 3 5 5
Sample Output
1 2 1 1 0
Hint
This problem has huge input data,use scanf() instead of cin to read data to avoid time limit exceed.
Source
题目大意:
在坐标上有n个星星,如果某个星星坐标为(x, y), 它的左下位置为:(x0,y0),x0<=x 且y0<=y。如果左下位置有a个星星,就表示这个星星属于level x
按照y递增,如果y相同则x递增的顺序给出n个星星,求出所有level水平的数量。
分析与总结:
因为输入是按照按照y递增,如果y相同则x递增的顺序给出的, 所以,对于第i颗星星,它的level就是之前出现过的星星中,横坐标x小于等于i星横坐标的那些星星的总数量(前面的y一定比后面的y小)。
所以,需要找到一种数据结构来记录所有星星的x值,方便的求出所有值为0~x的星星总数量。
树状数组和线段树都是很适合处理这种问题。
1 #include<cstdio> 2 #include<string.h> 3 #include<algorithm> 4 using namespace std; 5 #define clr(x,y) memset(x,y,sizeof(x)) 6 #define lson l,m,rt<<1 7 #define rson m+1,r,rt<<1|1 8 9 const int maxn=4e4; 10 int maxi,sum[maxn<<2],num[maxn]; 11 12 struct node 13 { 14 int x,y; 15 } star[maxn]; 16 17 void PushUp(int rt) 18 { 19 sum[rt]=sum[rt<<1]+sum[rt<<1|1]; 20 } 21 22 23 void Updata(int x,int l,int r,int rt) 24 { 25 int m; 26 27 if(l==r) { 28 sum[rt]++; 29 return; 30 } 31 32 m=(l+r)>>1; 33 if(x<=m) Updata(x,lson); 34 else Updata(x,rson); 35 36 PushUp(rt); 37 } 38 39 int query(int L,int R,int l,int r,int rt) 40 { 41 int m,ret=0; 42 43 if(L<=l && r<=R) { 44 return sum[rt]; 45 } 46 47 m=(l+r)>>1; 48 if(L<=m) ret+=query(L,R,lson); 49 if(R>m) ret+=query(L,R,rson); 50 51 return ret; 52 } 53 54 55 56 int main() 57 { 58 int n,ans; 59 60 maxi=-1; 61 clr(sum,0);clr(num,0); 62 scanf("%d",&n); 63 for(int i=0;i<n;i++) { 64 scanf("%d%d",&star[i].x,&star[i].y); 65 if(star[i].x>maxi) maxi=star[i].x; 66 } 67 68 for(int i=0;i<n;i++) { 69 ans=query(0,star[i].x,0,maxi,1); 70 Updata(star[i].x,0,maxi,1); 71 num[ans]++; 72 } 73 74 for(int i=0;i<n;i++) { 75 printf("%d ",num[i]); 76 } 77 78 79 return 0; 80 }