1.开闭原则(OCP)
遵循开闭原则设计出的模块具有两个主要特征:
(1)对于扩展是开放的(Open for extension)。这意味着模块的行为是可以扩展的。当应用的需求改变时,我们可以对模块进行扩展,使其具有满足那些改变的新行为。也就是说,我们可以改变模块的功能。
(2)对于修改是关闭的(Closed for modification)。对模块行为进行扩展时,不必改动模块的源代码或者二进制代码。模块的二进制可执行版本,无论是可链接的库、DLL或者.EXE文件,都无需改动。
2.通过UML认识OCP
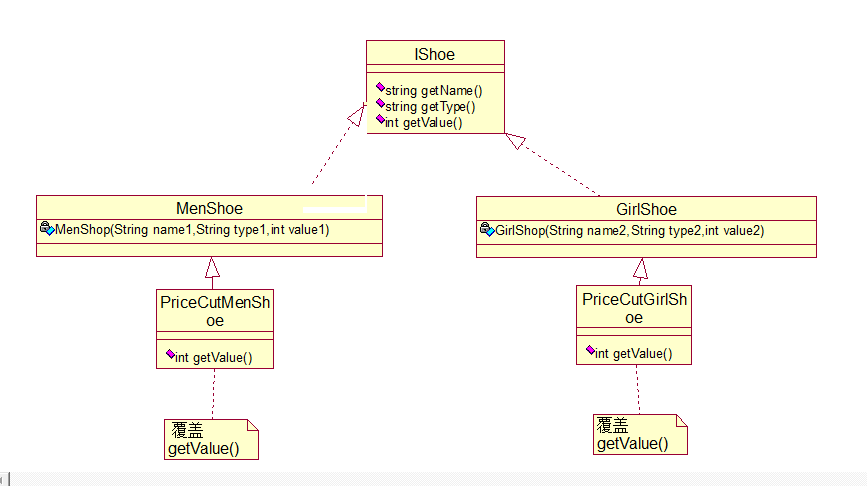
3.代码
(1)IShoe接口

1 package com.alibaba.www.miao; 2 3 public interface IShoe { 4 5 public String getName(); 6 public String getType(); 7 public int getValue(); 8 }
(2)MenShoe实现IShoe接口

1 package com.alibaba.www.miao; 2 3 public class MenShoe implements IShoe { 4 5 private String name; 6 private String type; 7 private int value; 8 9 public MenShoe(String name, String type, int value) { 10 super(); 11 this.name = name; 12 this.type = type; 13 this.value = value; 14 } 15 16 @Override 17 public String getName() { 18 return this.name; 19 } 20 21 @Override 22 public String getType() { 23 return this.type; 24 } 25 26 @Override 27 public int getValue() { 28 return this.value; 29 } 30 31 }
(3)GirlShoe实现IShoe接口

1 package com.alibaba.www.miao; 2 3 public class GirlShoe implements IShoe { 4 5 private String name; 6 private String type; 7 private int value; 8 9 public GirlShoe(String name, String type, int value) { 10 super(); 11 this.name = name; 12 this.type = type; 13 this.value = value; 14 } 15 16 @Override 17 public String getName() { 18 return this.name; 19 } 20 21 @Override 22 public String getType() { 23 return this.type; 24 } 25 26 @Override 27 public int getValue() { 28 return this.value; 29 } 30 31 }
(4)PriceCutMenShoe继承MenShoe类

1 package com.alibaba.www.miao; 2 3 public class PriceCutMenShoe extends MenShoe { 4 5 public PriceCutMenShoe(String name, String type, int value) { 6 super(name, type, value); 7 } 8 9 public int getValue() { 10 int primeCost = super.getValue(); 11 int cutPrice = 0; 12 if (primeCost > 200) { 13 cutPrice = cutPrice * 80 / 100; 14 } 15 if (primeCost > 150) { 16 cutPrice = cutPrice * 85 / 100; 17 } 18 return cutPrice; 19 } 20 }
(5)PriceCutGirlShoe继承GirlShoe类

1 package com.alibaba.www.miao; 2 3 public class PriceCutGirlShoe extends GirlShoe { 4 5 public PriceCutGirlShoe(String name, String type, int value) { 6 super(name, type, value); 7 } 8 9 public int getValue() { 10 int primeCost = super.getValue(); 11 int cutPrice = 0; 12 if (primeCost > 200) { 13 cutPrice = cutPrice * 75 / 100; 14 System.out.println("如果原价大于200元,则打折7.5折"); 15 } 16 if (primeCost > 150) { 17 cutPrice = cutPrice * 80 / 100; 18 System.out.println("如果原价大于150元,则打折8折"); 19 } else { 20 cutPrice = cutPrice * 85 / 100; 21 System.out.println("其他。则打8.5折"); 22 } 23 return primeCost; 24 } 25 }
(6)测试ocp.jsp,验证开闭原则

1 <%@page import="com.alibaba.www.miao.PriceCutGirlShoe"%> 2 <%@ page language="java" contentType="text/html; charset=UTF-8" 3 pageEncoding="UTF-8"%> 4 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> 5 <html> 6 <head> 7 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> 8 <title>Insert title here</title> 9 </head> 10 <body> 11 12 <% 13 PriceCutGirlShoe a = new PriceCutGirlShoe("女鞋名","女鞋类型",160); 14 out.print("鞋价为:"+a.getValue()); 15 %> 16 17 </body> 18 </html>
(7)参考目录结构