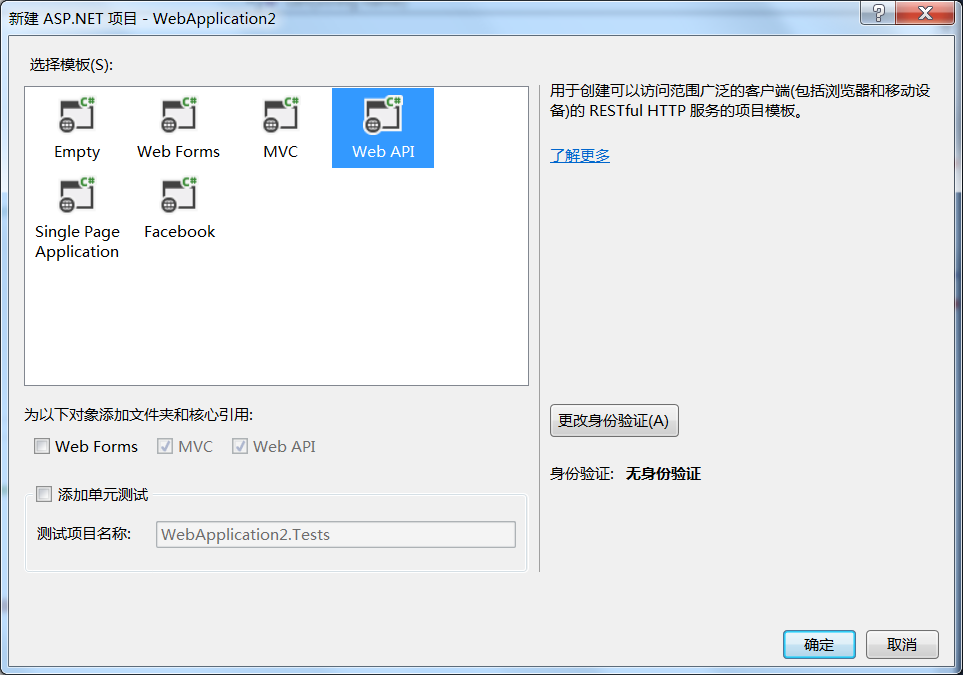
。
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
//配置API路由
GlobalConfiguration.Configure(WebApiConfig.Register);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
App_Start/WebApiConfig.cs
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API configuration and services
// Web API routes
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
Storages.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace TmplWebApiDemo.Models
{
public class Storages
{
public static IEnumerable<Student> Students { get; set; }
public static IEnumerable<Teacher> Teachers { get; set; }
static Storages()
{
Students = new List<Student>
{
new Student{Id =1,Name="张三",Age =11,Gender=false},
new Student{Id =2,Name="李四",Age =21,Gender=true},
new Student{Id =3,Name="王五",Age =22,Gender=false},
new Student{Id =4,Name="赵飞燕",Age =25,Gender=true},
new Student{Id =5,Name="王刚",Age =31,Gender=true}
};
Teachers = new List<Teacher>
{
new Teacher{Id =1,Name="老师1",Age =11,Gender=false},
new Teacher{Id =2,Name="老师2",Age =21,Gender=true},
new Teacher{Id =3,Name="老师3",Age =22,Gender=false},
new Teacher{Id =4,Name="老师4",Age =25,Gender=true}
};
}
}
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public bool Gender { get; set; }
}
public class Student : Person
{
}
public class Teacher : Person
{
}
}
StudentsController.cs
/// <summary>
/// 学生资源集合
/// </summary>
public class StudentsController : ApiController
{
//c r u d
/// <summary>
/// GET / Students/
/// </summary>
public IEnumerable<Student> Get()
{
return Storages.Students;
}
/// <summary>
/// GET / students/zhangsan return entity
/// </summary>
/// <returns></returns>
public Student Get(string name)
{
return Storages.Students.FirstOrDefault(s => s.Name.Equals(name, StringComparison.InvariantCultureIgnoreCase));
}
}
测试结果
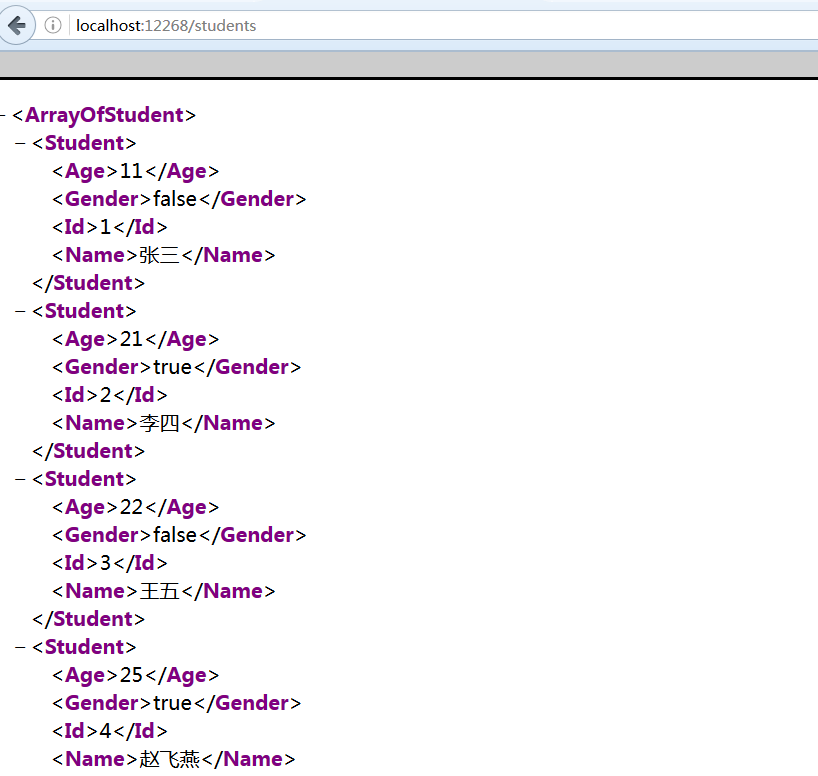
用 postman 测试
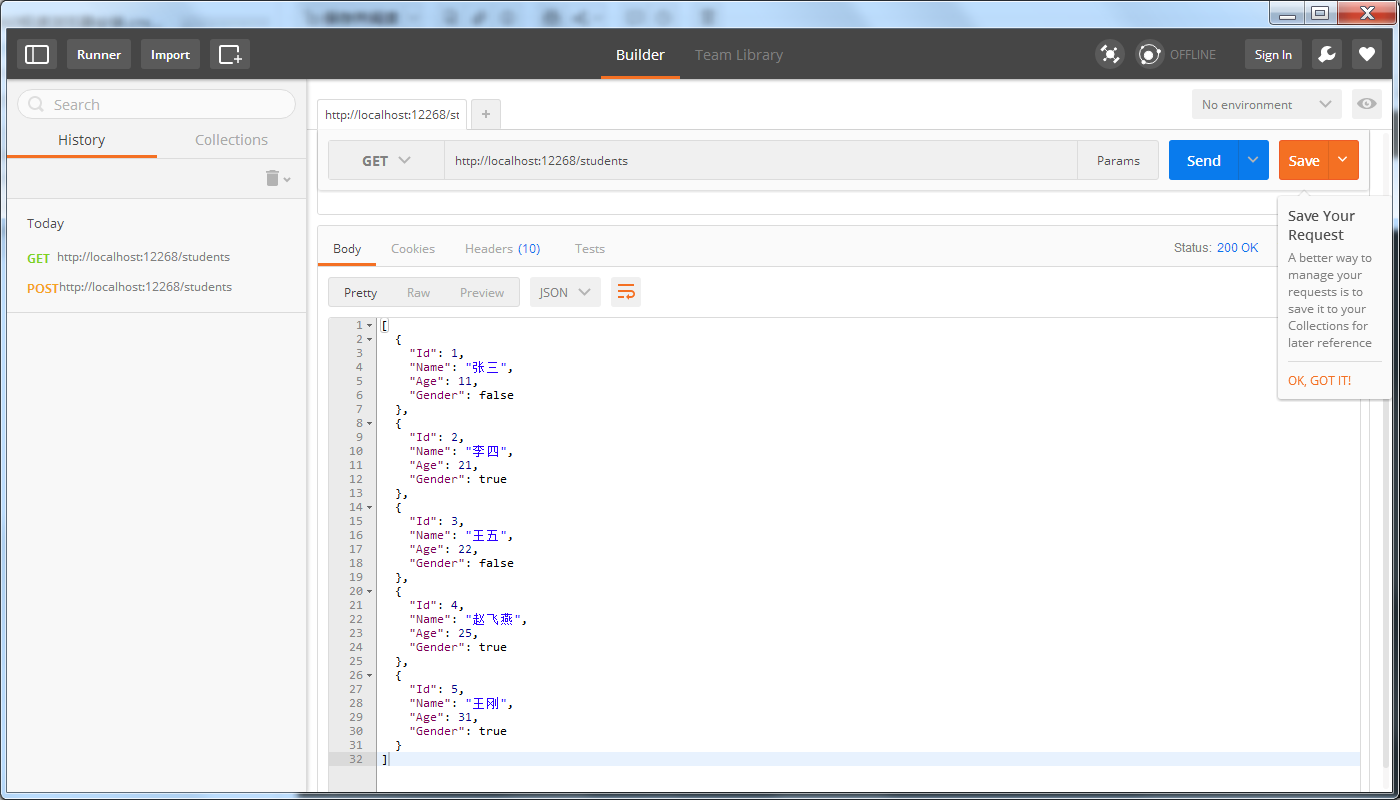