1.工程结构
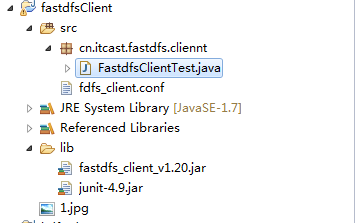
2.代码内容
FastdfsClientTest.java代码
package cn.itcast.fastdfs.cliennt;import java.io.File;import java.io.FileNotFoundException;import java.io.FileOutputStream;import java.io.IOException;import java.util.UUID;import org.csource.common.NameValuePair;import org.csource.fastdfs.ClientGlobal;import org.csource.fastdfs.FileInfo;import org.csource.fastdfs.StorageClient;import org.csource.fastdfs.StorageClient1;import org.csource.fastdfs.StorageServer;import org.csource.fastdfs.TrackerClient;import org.csource.fastdfs.TrackerServer;import org.junit.Before;import org.junit.Test;/*** 测试FastDFS API使用* @FileName:FastdfsClientTest.java* @Description: TODO()* @Author: tangwan* @Date: 2015年4月23日 下午1:48:45* @since: JDK 1.8*/public class FastdfsClientTest {public String conf_filename = null;public String local_filename = null;@Beforepublic void confInit(){conf_filename = FastdfsClientTest.class.getResource("/fdfs_client.conf").getPath();System.out.println("配置文件夹路径:"+conf_filename);local_filename = "1.jpg";System.out.println("待上传文件路径:"+local_filename);}// 客户端配置文件(绝对路径);使用下面注释的路径,请注释上面confInit方法//public String conf_filename = "D:\DebugLove\workspace\DEV-2\fastdfsClient\src\cn\itcast\fastdfs\cliennt\fdfs_client.conf";// 本地文件,要上传的文件//public String local_filename = "C:\Users\Administrator\Desktop\2.jpg";// 将字节流写到磁盘生成文件private void saveFile(byte[] b, String path, String fileName) {File file = new File(path + fileName);FileOutputStream fileOutputStream = null;try {fileOutputStream = new FileOutputStream(file);fileOutputStream.write(b);} catch (FileNotFoundException e) {e.printStackTrace();} catch (IOException e) {e.printStackTrace();} finally {if (fileOutputStream != null) {try {fileOutputStream.close();} catch (IOException e) {e.printStackTrace();}}}}// 上传文件//文件保存在storage设置的baseUrl下 /home/FastDFS/fdfs_storage/data/00/00包括:wKgBFVcMJNyAZtDnAASUcjwYjng393.jpg和wKgBFVcMJNyAZtDnAASUcjwYjng393.jpg-m@Testpublic void testUpload() {//上传5次for (int i = 0; i < 1; i++) {try {ClientGlobal.init(conf_filename);TrackerClient tracker = new TrackerClient();TrackerServer trackerServer = tracker.getConnection();StorageServer storageServer = null;StorageClient storageClient = new StorageClient(trackerServer, storageServer);NameValuePair nvp[] = new NameValuePair[] { new NameValuePair("item_id", "100010"), new NameValuePair("width", "80"),new NameValuePair("height", "90") };String fileIds[] = storageClient.upload_file(local_filename, null, nvp);System.out.println(fileIds.length);System.out.println("组名:" + fileIds[0]);System.out.println("路径: " + fileIds[1]);} catch (FileNotFoundException e) {e.printStackTrace();} catch (IOException e) {e.printStackTrace();} catch (Exception e) {e.printStackTrace();}}}// 下载文件@Testpublic void testDownload() {try {ClientGlobal.init(conf_filename);TrackerClient tracker = new TrackerClient();TrackerServer trackerServer = tracker.getConnection();StorageServer storageServer = null;// StorageClient storageClient = new StorageClient(trackerServer,storageServer);StorageClient1 storageClient = new StorageClient1(trackerServer, storageServer);byte[] b = storageClient.download_file("group1", "M00/00/00/wKgBFVcMJN6AGuYuAASUcjwYjng476.jpg");if (b != null) {System.out.println(b.length);saveFile(b, "d:\temp\", UUID.randomUUID().toString() + ".jpg");}} catch (Exception e) {e.printStackTrace();}}// 获取文件信息@Testpublic void testGetFileInfo() {try {ClientGlobal.init(conf_filename);TrackerClient tracker = new TrackerClient();TrackerServer trackerServer = tracker.getConnection();StorageServer storageServer = null;StorageClient storageClient = new StorageClient(trackerServer, storageServer);FileInfo fi = storageClient.get_file_info("group1", "M00/00/00/wKgBFVcMJN6AGuYuAASUcjwYjng476.jpg");System.out.println(fi.getSourceIpAddr());System.out.println(fi.getFileSize());System.out.println(fi.getCreateTimestamp());System.out.println(fi.getCrc32());} catch (Exception e) {e.printStackTrace();}}// 获取文件自定义的mate信息(key、value)@Testpublic void testGetFileMate() {try {ClientGlobal.init(conf_filename);TrackerClient tracker = new TrackerClient();TrackerServer trackerServer = tracker.getConnection();StorageServer storageServer = null;StorageClient storageClient = new StorageClient(trackerServer, storageServer);NameValuePair nvps[] = storageClient.get_metadata("group1", "M00/00/00/wKgBFVcMJN6AGuYuAASUcjwYjng476.jpg");if (nvps != null) {for (NameValuePair nvp : nvps) {System.out.println(nvp.getName() + ":" + nvp.getValue());}}} catch (Exception e) {e.printStackTrace();}}// 删除文件//从操作系统storage节点,物理删除/home/FastDFS/fdfs_storage/data/00/00直接消失@Testpublic void testDelete() {try {ClientGlobal.init(conf_filename);TrackerClient tracker = new TrackerClient();TrackerServer trackerServer = tracker.getConnection();StorageServer storageServer = null;StorageClient storageClient = new StorageClient(trackerServer, storageServer);int i = storageClient.delete_file("group1", "M00/00/00/wKgBFVcMJNyAZtDnAASUcjwYjng393.jpg");System.out.println(i == 0 ? "删除成功" : "删除失败:" + i);} catch (Exception e) {e.printStackTrace();}}}
2.fdfs_client.conf #配置文件
# connect timeout in seconds# default value is 30sconnect_timeout=30# network timeout in seconds# default value is 30snetwork_timeout=60# the base path to store log filesbase_path=/home/FastDFS# tracker_server can ocur more than once, and tracker_server format is# "host:port", host can be hostname or ip addresstracker_server=192.168.1.20:22122#tracker_server=192.168.101.4:22122#standard log level as syslog, case insensitive, value list:### emerg for emergency### alert### crit for critical### error### warn for warning### notice### info### debuglog_level=info# if use connection pool# default value is false# since V4.05use_connection_pool = false# connections whose the idle time exceeds this time will be closed# unit: second# default value is 3600# since V4.05connection_pool_max_idle_time = 3600# if load FastDFS parameters from tracker server# since V4.05# default value is falseload_fdfs_parameters_from_tracker=false# if use storage ID instead of IP address# same as tracker.conf# valid only when load_fdfs_parameters_from_tracker is false# default value is false# since V4.05use_storage_id = false# specify storage ids filename, can use relative or absolute path# same as tracker.conf# valid only when load_fdfs_parameters_from_tracker is false# since V4.05storage_ids_filename = storage_ids.conf#HTTP settingshttp.tracker_server_port=80#use "#include" directive to include HTTP other settiongs##include http.conf
3.待上传文件,美女,给大家看看
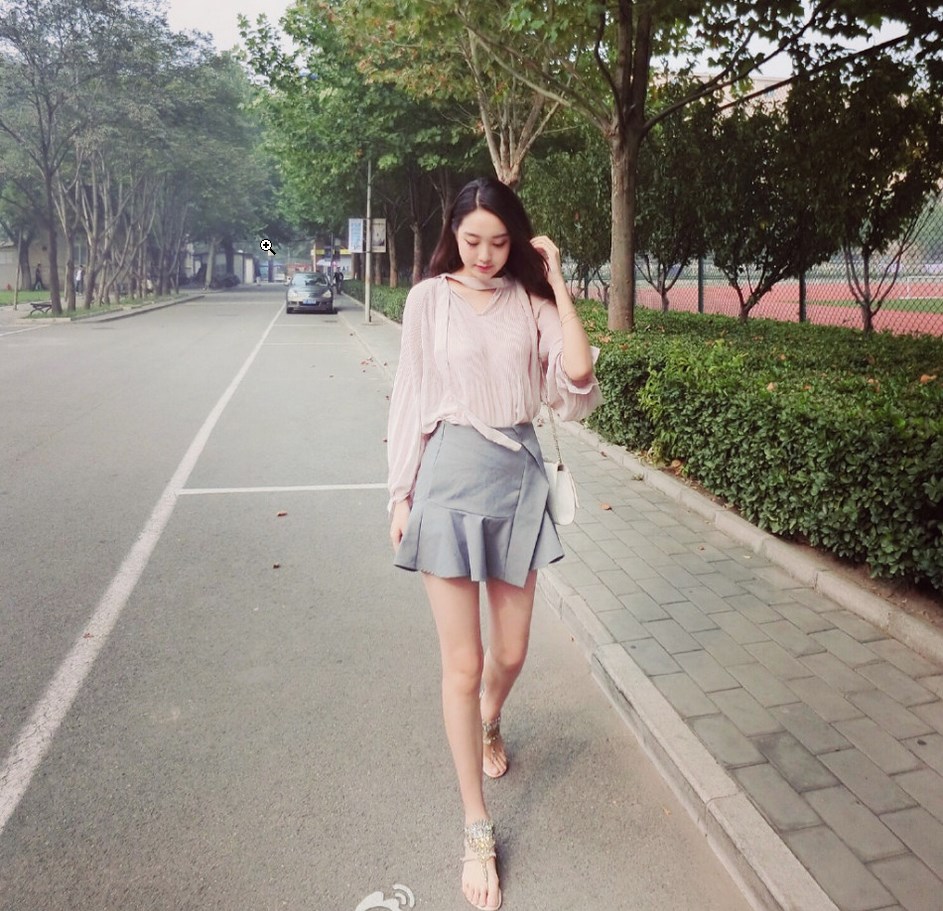
4.jar包下载