1.创建/编辑
vim 1.sh
输出命令:echo "hello world!!"
显示hello.txt文本文件中的内容:$ cat hello.txt
显示日期:echo `date`
显示日历:cal
执行shell 脚本
2.编辑内容
i
退出:esc 键
:wq! 保存退出
:q! 不保存 退出
3.变量
name="zhangsan"
4.输出变量
echo ${name}
5.输出变量的长度
echo ${#name}
6.字符串的截取
echo ${name:1:3}
7.字符串的拼接
pj1 ="hello ,${name} "
8.数组
arr=(1 2 3 9 87 89 4584 548 54 45 4)
输出数字的所有元素:
echo ${arr[@]}
echo ${arr[*]}
计算数组的长度:
echo ${#arr[@]}
9. 单个数组元素的长度
arr1=(sdfer fgsafds mkj juihu juihuh jmiojijiohuigyg)
echo ${#arr[5]}
echo ${#arr[5]} ${arr[5:2:4]}
10.变量的只读
url="www.baidu.com"
readonly url
url='www.xinlang.com'
11.变量的删除:
myURL="www.gaoxiaojun.com"
unset myURL
echo ${myURL}
echo "myURL unset success"
12.只读的变量不允许删除
myURL="www.gaoxiaojun.com"
readonly myURL
unset myURL
echo ${myURL}
13.shell 注释
以# 开始 么有多行注释 只能每行添加#
14.运算符
var=`expr 2+6` expr 能完成表达式的运算
echo"sum:$var'
var=`expr 4 + 6`
r=`expr 4 + 5`
a=`expr 4 * 6`
b=`expr 8 / 2`
c=`expr 12 - 10`
echo $var $r $a $b $c
15 数字判断
-gt 大于
r=`expr 4 + 5` a=`expr 4 - 2` if [ $r -gt $a ] then echo $r 大于 $a else echo $r 小于 $a
fi
-lt 小于
r=`expr 4 + 5` a=`expr 4 - 2` if [ $r -lt $a ] then echo $r 小于 $a else echo $r 大于 $a fi
-ge 大于等于
r=`expr 4 + 5` a=`expr 4 - 2` if [ $r -ge $a ] then echo $r 大于等于 $a else echo $r 小于等于 $a
fi
-le 小于等于
r=`expr 4 + 5`
a=`expr 4 - 2`
if [ $r -le $a ]
then
echo $r 小于等于 $a
else
echo $r 大于等于 $a
fi
16.逻辑判断
-组合测试条件:
- -a: and
- -o: or
- !: 非
备注:如果同时存在三个运算符 优先级顺序:! > -a >-o
a=5 b=2 c=0 d =3 if [ $a -gt $b -a $c -lt $d ] then echo '条件为真' else echo ‘条件为假’ fi
16. 字符串判断
判断是否有包含;
1.利用字符串运算符 =~ 直接判断strA是否包含strB。
strA="helloworld" strB="low" if [[ $strA =~ $strB ]] then echo "包含" else echo "不包含" fi
2用通配符*号代理strA中非strB的部分,如果结果相等说明包含,反之不包含
A="helloworld" B="low" if [[ $A == *$B* ]] then echo "包含" else echo "不包含" fi
3. -z 字符串长度是否为0 为空返回true
a='asd' if [ -z $a ] then echo 'a 长度不为空'
4.-n 字符串长度是否为0 为空返回false
a='asd' if [ -n $a ] then echo 'a 长度不为空'
5. [ $str ] 检测字符串是否为空
a='asd' if [ str $a ] then echo 'a 不为空'
17.文件的判断
-d file 检测是否为目录
a=1.sh if [ -d $a ] then echo 'a 是目录'
-f file 检测是否为文件
a=1.sh if [ -f $a ] then echo 'a 是文件'
-r file 检测是否可读
a=1.sh if [ -r $a ] then echo 'a 是可读'
-w file 检测是否可写
a=1.sh if [ -w $a ] then echo 'a 是可写'
-x file 检测是否可执行
a=1.sh if [ -x $a ] then echo 'a 是可执行'
-s file 检测是否为空
a=1.sh if [ -s $a ] then echo 'a 为空'
-e file 检测是否存在
a=1.sh if [ -e $a ] then echo 'a 文件存在'
18. 显示不换行 c 搭配 -e 使用
echo -e 'hello world c'
19.显示结果保存在指定文件中 >
> 是覆盖
>> 是追加到最后
echo 'hello world ' > 2.sh
echo 'hello world ' >> 2.sh
20. 输出
printf format-string [arguments...]
printf %d-10s %s %c 10 python ss
printf "%-10s %-8s %-4.2f
" 郭靖 男 66.123 %-10s 指一个宽度为10个字符
printf “%s %s %s
” a b c d e f g h i j
结果:
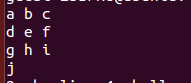
21 后退
换行
水平制表符
\反斜杠字符|
22.判断符号 [] 与 test 功能 一致
[] 每个中间数据都要用空格隔开
echo ‘请输入第一个数字 ’
read num1
echo ‘请输入第二个数字 ’
read num2
if test $num1 -gt $num2
then
echo "num1 大于 num2"
elif test $num1 -lt $num2
then
echo 'num1 小于 num2'
elif test $num1 -eq $num2
then
echo "num1 等于 num2"
else
echo "请输入有效数字"
fi
echo ‘请输入第一个数字 ’
read num1
echo ‘请输入第二个数字 ’
read num2
if [ $num1 -gt $num2 ]
then
echo "num1 大于 num2"
elif [ $num1 -lt $num2 ]
then
echo "num1 大于 num2"
elif [ $num1 -eq $num2 ]
then
echo "num1 等于 num2"
else
echo "请输入有效数字"
fi
23. case in 语句
aa=5 case $aa in 1) echo '变量是1' ;; 2) echo '变量是2' ;; *) echo '变量不是1 或2' 而是$aa ;;
esac
24. while 语句
let 执行一个或多个表达式 不需要加上 $
i=4 while(($<=5)) do echo $i let 'i++' done
25.whil 语句中的判断
i=1 while(( $i<=10 )) do if (( $i %2 ==0 )) then echo $i fi let 'i++' done
26. for 循环
for (( n=1;n<10;n++))
do
if [ $n -gt 8 ]
then
echo $n
fi
done
27 ping 链接
ping -c www.baidu.com