1、什么是策略模式?
策略模式指定义一组算法簇,并且每一个算法都有自己具体的算法实现,算法的使用可以根据业务需要在客户端进行替换。
2、策略模式角色
2.1 抽象策略角色
抽象策略角色(Istrategy)定义了算法功能。
2.2 具体策略角色
具体策略角色实现了抽象策略角色中定义的算法。
2.3 上下文角色
上下文角色(Context)封装了可能的变化,屏蔽上层对具体策略的直接引用。
3、代码实现
/**
* 抽象策略接口
*/
public interface IStrategy {
/**
* 定义一个计算商品价格的接口
* @param orginPrice
* @return
*/
double calPrice(double orginPrice);
}
/**
* 普通会员策略实现
* 没有优惠,按原价计算
*/
public class NormalStrategy implements IStrategy {
@Override
public double calPrice(double orginPrice) {
return orginPrice;
}
}
/**
* 黄金会员策略实现
* 按9折计价
*/
public class GoldStrategy implements IStrategy{
@Override
public double calPrice(double orginPrice) {
return orginPrice * 0.9;
}
}
/**
* Plus 会员策略实现
* Plus 按8折计价,如果折后价大于100,可以使用10块钱的优惠券
*/
public class PlusStrategy implements IStrategy {
@Override
public double calPrice(double orginPrice) {
double reamPrice = orginPrice * 0.8;
if (reamPrice > 100) {
reamPrice = reamPrice - 10;
}
return reamPrice;
}
}
/**
* 包装算法策略的使用
*/
public class Context {
private IStrategy strategy;
public IStrategy getStrategy() {
return strategy;
}
public void setStrategy(IStrategy strategy) {
this.strategy = strategy;
}
/**
* 调用具体策略计算商品价格
* @param orginPrice
* @return
*/
public double calPrice(double orginPrice) {
return strategy.calPrice(orginPrice);
}
}
/**
* 模拟客户端调用策略
*/
public class MainTest {
public static void main(String[] args) {
IStrategy normalStrategy = new NormalStrategy();
IStrategy goldStrategy = new GoldStrategy();
IStrategy plusStrategy = new PlusStrategy();
Context context = new Context();
context.setStrategy(normalStrategy);
double result = context.calPrice(153.32);
System.out.println("normal price= " + result);
context.setStrategy(goldStrategy);
result = context.calPrice(153.32);
System.out.println("gold price= " + result);
context.setStrategy(plusStrategy);
result = context.calPrice(153.32);
System.out.println("plus price= " + result);
}
}
4、运行结果
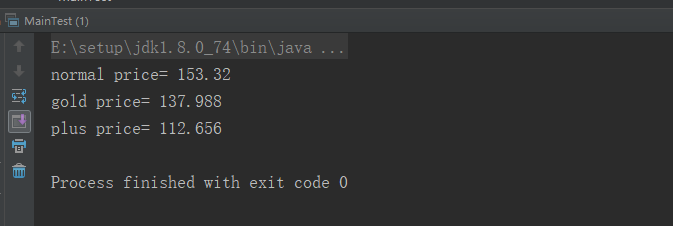