素数环(暴力)(紫书194页)
Description
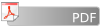
A ring is composed of n (even number) circles as shown in diagram. Put natural numbers into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
Input
n (0 < n <= 16)n (0 < n <= 16)
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements.You are to write a program that completes above process.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
题意:
输入正整数n,把1—n组成一个环,使相邻的两个整数为素数。输出时从整数1开始,逆时针排列。恰好能构成一个环输出一次,n (0 < n <= 16)
分析:
每个环对应于1——n的一个排列,但排列总数高达16!=2*10^13,普通写法会超时,应用回溯法。
DFS。深度优先遍历。
代码:

1 #include<cstdio> 2 #include<iostream> 3 using namespace std; 4 5 int n, A[50], isp[50], vis[50]; 6 7 int is(int x) 8 { 9 for(int i = 2; i*i <= x; i++) 10 if(x % i == 0) 11 return 0; 12 return 1; 13 } 14 15 16 17 void dfs(int cur) 18 { 19 if(cur==n&&isp[A[0]+A[n-1]]) 20 { 21 for(int i=0;i<n;i++) 22 printf("%d ",A[i]); 23 printf("\n"); 24 } 25 else for(int i=2;i<=n;i++) 26 if(!vis[i]&&isp[i+A[cur-1]]) 27 { 28 A[cur] = i; 29 vis[i] = 1; 30 dfs(cur+1); 31 vis[i] = 0; 32 } 33 } 34 35 int main() 36 { 37 //int n; 38 int m=0; 39 while(scanf("%d",&n)!=EOF) 40 { 41 for(int i=2;i<=n*2;i++) 42 isp[i] = is(i); 43 memset(vis, 0, sizeof(vis)); 44 A[0] = 1; 45 m++; 46 printf("Case %d:\n",m); 47 dfs(1); 48 printf("\n"); 49 } 50 return 0; 51 }