@Entity("bands") public class Band { @Id ObjectId id; String name; String genre; @Reference Distributor distributor; @Reference("catalog") List<Song> songs = new ArrayList<Song>(); @Embedded List<String> members = new ArrayList<String>(); @Embedded("info") ContactInfo info;
@Entity("songs") public class Song { @Id ObjectId id; String name;
}
@Entity("distributors") public class Distributor { @Id ObjectId id; String name;
}
@Entity
注释是必需的。其声明了在专用MongoDB集合上该类作为文档将持久保存。在默认情况下,Morphia使用类名称来命名集合。
可以使用value 注释MongoDB集合名称,设置noClassNameStored为true,
如@Entity(value = "params",noClassnameStored=true)
@ID
@Id
注释指示 Morphia 哪个字段用作文档 ID。如果您试图持久保存对象(其 @Id
注释的字段为 null),则 Morphia 会为您自动生成 ID 值。
id 可以时ObjectId,也可以时String类型。
@Transient
类属性标记@Transient注释则表明这个字段将不被持久化到数据库。
Morphia 试图持久保存每一个它遇到的没有注释的字段,除非它们标有 @Transient
注释,才会不保存到数据库中。
没有任何注解的,就会默认持久化到数据库中,相当于使用 @Embedded注解,只不过用 @Embedded可以指定别名。
@Embedded
使用此注解说明成员对象将被视为嵌入的(embedded)。它会显示为集合中父文档的子集。
除非注释有@Transient或 @Reference
,否则默认成员对象将被视为嵌入的(embedded)
@Entity("bands")
public class Band {
@Id
ObjectId id;
String name;
String genre;
@Reference
Distributor distributor;
@Reference("catalog")
List<
Song
> songs = new ArrayList<
Song
>();
@Embedded
List<
String
> members = new ArrayList<
String
>();
@Embedded("info")
ContactInfo info;
public class ContactInfo {
public ContactInfo() {
}
String city;
String phoneNumber;
Band band = new Band();
band.setName("Love Burger");
band.getMembers().add("Jim");
band.getMembers().add("Joe");
band.getMembers().add("Frank");
band.getMembers().add("Tom");
band.setGenre("Rock");
datastore.save(band);
members List
看上去如下所示
"members" : [ "Jim", "Joe", "Frank", "Tom"]
info
属性是另外一个嵌入的对象。在本例中,我通过 info
值明确地设置 @Embedded
注释。这会覆盖文档中子集的默认命名,否则就会被称为 contactInfo
。例如:
"info" : { "city" : "Brooklyn", "phoneNumber" : "718-555-5555" }
查询结果:
> db.bands.find();
{ "_id" : ObjectId("4cf7cbf9e4b3ae2526d72587"), "className" :
"com.bandmanager.model.Band", "name" : "Love Burger", "genre" : "Rock",
"members" : [ "Jim", "Joe", "Frank", "Tom" ] }
可以看到:ContactInfo
类缺少 @Entity
注释。这是故意而为的,因为我不需要 ContactInfo
的专用集合。它实例总是被嵌入 band
文档。@Entity(value = "MCH_Community_Clew", noClassnameStored = true) public class CommunityClew extends MongoBaseEntity implements Serializable { private static final long serialVersionUID = 1L; private long id;//id private String mobile; private String community;//社区 private String status; private Date createTime;//加入时间 private Date modifyTime;//修改时间 private String modifyUser;//修改人 private String remark; //记录最新一次备注 }
public class CommunityClewRecord extends MongoBaseEntity implements Serializable { private static final long serialVersionUID = 1L; private long id;//id private long fatherId; private String beforeStatus;//之前状态 private String status;//当前状态 private String remark;//备注 private Date modifyTime;//时间 private String modifyUser;//修改人
@Embedded("records")
private List<CommunityClewRecord> communityClewRecords;
}
CommunityClewRecord record=new CommunityClewRecord(1, 1, "测试", status, remark,now,user); CommunityClew clew=new CommunityClew(); clew.setId(communityClewMongo.getMaxId()+1); clew.setCommunity(community); clew.setMobile(mobile); clew.setCreateTime(new Date()); clew.setStatus("待沟通"); clew.setCommunityClewRecords(record); communityClewMongo.insert(clew);
对CommunityClew的communityClewRecords列表添加两条CommunityClewRecord记录,查询monggo标,结果如下:
@Reference
@Reference 注释说明对象是对另外一个集合中的文档的引用。在从 MongoDB集合中加载对象时,Morphia遵循着这些引用来建立对象关系。即引用的字段会根据内部建立的关系图,自动加载。
这就要求被引用的对象先保存,然后再把被对象赋值给引用的字段,包含引用字段的对象再保存。
如:
Band band = new Band(); band.setName("Love Burger"); band.getMembers().add("Jim"); band.getMembers().add("Joe"); band.getMembers().add("Frank"); band.getMembers().add("Tom"); band.setGenre("Rock");datastore.save(band);
//持久化
查询:
> db.bands.find();
{ "_id" : ObjectId("4cf7cbf9e4b3ae2526d72587"), "className" :
"com.bandmanager.model.Band", "name" : "Love Burger", "genre" : "Rock",
"members" : [ "Jim", "Joe", "Frank", "Tom" ] }
Song song1 = new Song("Stairway");
Song song2 = new Song("Free Bird");
datastore.save(song1);
datastore.save(song2);
songs
集合,应该看到如下所示:> db.songs.find();
{ "_id" : ObjectId("4cf7d249c25eae25028ae5be"), "className" :
"com.bandmanager.model.Song", "name" : "Stairway" }
{ "_id" : ObjectId("4cf7d249c25eae25038ae5be"), "className" :
"com. bandmanager.model.Song", "name" : "Free Bird" }
Song
还没有被 band
引用。我将它们添加到 band
并查看发生了什么:band.getSongs().add(song1);
band.getSongs().add(song2);
datastore.save(band);
bands
集合时,应该看到songs
集合被保存为一个被称为 catalog
的数组,作为两个 DBRef
。因为@Reference("catalog")起了一个别名,如果不设置括号中的名称,存储的引用catalog
的数组应该是songs。{ "_id" : ObjectId("4cf7d249c25eae25018ae5be"), "name" : "Love Burger", "genre" : "Rock",
"catalog" : [
{
"$ref" : "songs",
"$id" : ObjectId("4cf7d249c25eae25028ae5be")
},
{
"$ref" : "songs",
"$id" : ObjectId("4cf7d249c25eae25038ae5be")
}
], "members" : [ "Jim", "Joe", "Frank", "Tom"] }
@Reference
例子:@Entity(value = "MCH_Community_Clew", noClassnameStored = true) public class CommunityClew extends MongoBaseEntity implements Serializable { private static final long serialVersionUID = 1L; private long id;//id private String mobile; private String community;//社区 private String status; private Date createTime;//加入时间 private Date modifyTime;//修改时间 private String modifyUser;//修改人 private String remark; //记录最新一次备注 @Reference private List<CommunityClewRecord> communityClewRecords; }
@Entity(value = "MCH_Community_Clew_Record", noClassnameStored = true) public class CommunityClewRecord extends MongoBaseEntity implements Serializable { private static final long serialVersionUID = 1L; private long id;//id private long fatherId; private String beforeStatus;//之前状态 private String status;//当前状态 private String remark;//备注 private Date modifyTime;//时间 private String modifyUser;//修改人 }
public boolean saveCommunityClewRecordAndCommunityClew(Map<String, Object> map) { long id=MapUtils.getLong(map, "id"); String status = MapUtils.getString(map,"status"); String remark = MapUtils.getString(map,"remark"); String user = MapUtils.getString(map,"user"); long recordMaxId = communityClewRecordMongo.getMaxId(); CommunityClew clew = communityClewMongo.queryCommunityClew(id); Date now=new Date(); CommunityClewRecord record=new CommunityClewRecord(recordMaxId+1, clew.getId(), clew.getStatus(), status, remark,now,user); communityClewRecordMongo.insert(record); return communityClewMongo.saveCommunityClewByUpdate(record,user,id); }
MCH_Community_Clew_Record数据
MCH_Community_Clew数据

展开显示:
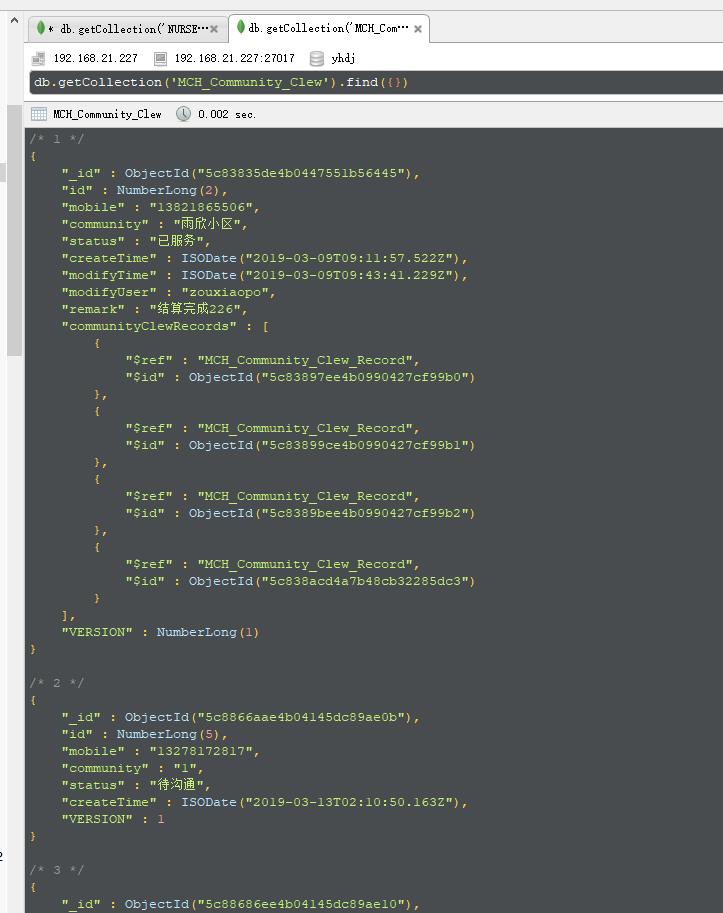
song1
和 song2
,然后将它们添加到 band
。参考文章: