Fox Ciel is playing a mobile puzzle game called "Two Dots". The basic levels are played on a board of size n × m cells, like this:
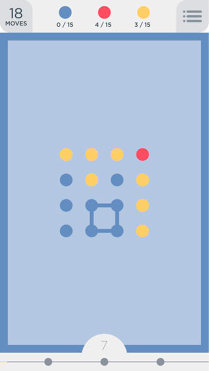
Each cell contains a dot that has some color. We will use different uppercase Latin characters to express different colors.
The key of this game is to find a cycle that contain dots of same color. Consider 4 blue dots on the picture forming a circle as an example. Formally, we call a sequence of dots d1, d2, ..., dk a cycle if and only if it meets the following condition:
- These k dots are different: if i ≠ j then di is different from dj.
- k is at least 4.
- All dots belong to the same color.
- For all 1 ≤ i ≤ k - 1: di and di + 1 are adjacent. Also, dk and d1 should also be adjacent. Cells x and y are called adjacent if they share an edge.
Determine if there exists a cycle on the field.
The first line contains two integers n and m (2 ≤ n, m ≤ 50): the number of rows and columns of the board.
Then n lines follow, each line contains a string consisting of m characters, expressing colors of dots in each line. Each character is an uppercase Latin letter.
Output "Yes" if there exists a cycle, and "No" otherwise.
3 4
AAAA
ABCA
AAAA
Yes
3 4
AAAA
ABCA
AADA
No
4 4
YYYR
BYBY
BBBY
BBBY
Yes
7 6
AAAAAB
ABBBAB
ABAAAB
ABABBB
ABAAAB
ABBBAB
AAAAAB
Yes
2 13
ABCDEFGHIJKLM
NOPQRSTUVWXYZ
No
In first sample test all 'A' form a cycle.
In second sample there is no such cycle.
The third sample is displayed on the picture above ('Y' = Yellow, 'B' = Blue, 'R' = Red).
题意:判断图中是否存在长度大于4的回路(由同一种字母组成的)。
思路:DFS,注意每次不往回搜索,直到搜索结束或者搜到有标记的位置停止。
1 #include<iostream> 2 #include<cstdio> 3 #include<cstdlib> 4 #include<cstring> 5 #include<string> 6 #include<queue> 7 #include<algorithm> 8 #include<map> 9 #include<iomanip> 10 #include<climits> 11 #include<string.h> 12 #include<numeric> 13 #include<cmath> 14 #include<stdlib.h> 15 #include<vector> 16 #include<stack> 17 #include<set> 18 #define FOR(x, b, e) for(int x=b;x<=(e);x++) 19 #define REP(x, n) for(int x=0;x<(n);x++) 20 #define INF 1e7 21 #define MAXN 100010 22 #define maxn 1000010 23 #define Mod 1000007 24 #define N 1010 25 using namespace std; 26 typedef long long LL; 27 28 29 char G[55][55]; 30 int vis[55][55]; 31 int dx[] = {-1, 0, 0, 1}, dy[] = {0, 1, -1, 0}; 32 int n, m; 33 bool ok; 34 35 void dfs(int x,int y, int d) 36 { 37 vis[x][y] = 1; 38 REP(i, 4) { 39 if (i == 3 - d) continue; 40 int nx = x + dx[i]; 41 int ny = y + dy[i]; 42 if (nx == 0 || ny == 0 || ny == m+1 || nx == n+1) continue; 43 if (G[x][y] == G[nx][ny]) { 44 if (vis[nx][ny]) { 45 ok = true; 46 return; 47 } 48 else dfs(nx, ny, i); 49 } 50 } 51 } 52 53 int main() 54 { 55 cin >> n >> m; 56 FOR(i, 1, n) 57 cin >> G[i] + 1; 58 FOR(i, 1, n) 59 FOR(j, 1, m) { 60 if (!vis[i][j]) 61 dfs(i, j, 4); 62 } 63 puts(ok ? "Yes" : "No"); 64 return 0; 65 }