本节内容
- 列表、
- 元组操作
- 购物车程序
- 字符串操作
- 字典操作
- 3级菜单
- 作业(购物车优化)
1. 列表操作
1.定义列表
names = ['Alex',"Tenglan",'Eric']
2.追加
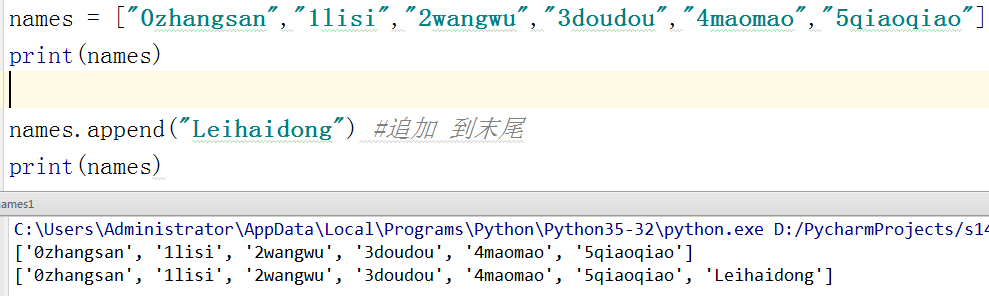
3.插入

4.修改

5.打印元素
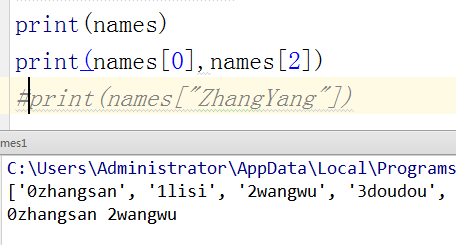
6.切片


7.索引(获取下标) 和统计

8.删除 和 清除

9.翻转和排序

10.扩展

11.Copy

12.浅copy ,深copy
13.循环,打印列表

14.步长切片

2.元组操作
元组其实跟列表差不多,也是存一组数,只不是它一旦创建,便不能再修改,所以又叫只读列表 它只有2个方法,一个是count,一个是index,完毕。

3.购物车程序
请闭眼写出以下程序。 程序:购物车程序 需求: 1. 启动程序后,让用户输入工资,然后打印商品列表 2. 允许用户根据商品编号购买商品 3. 用户选择商品后,检测余额是否够,够就直接扣款,不够就提醒 可随时退出,退出时,打印已购买商品和余额




# coding=utf-8 # Author:L product_list = [ ("Iphone",5800), ("Mac pro",1200), ("Bike",100), ("watch",1000), ("Coffee",12), ("Alex Python",120) ] shopping_list = [] salary = input("Input your salary: ") if salary.isdigit(): salary = int(salary) while True: for index,item in enumerate(product_list): #print(product_list.index(item),item) print(index,item) user_choic = input("选择要买的?》》》:") if user_choic.isdigit(): user_choic = int(user_choic) if user_choic < len(product_list) and user_choic >= 0: p_item = product_list[user_choic] if p_item[1] <= salary: #买的起 shopping_list.append(p_item) salary -= p_item[1] print("Added %s into your shopping cart,your current balance is 33[31;1m%s 33[0m" %(p_item,salary) ) else: print("