超级简陋的记账本(完结):
MainActivity.java
package com.example.myapplicationsecond; import android.content.DialogInterface; import android.database.Cursor; import android.os.Bundle; import com.google.android.material.floatingactionbutton.FloatingActionButton; import com.google.android.material.snackbar.Snackbar; import androidx.appcompat.app.AlertDialog; import androidx.appcompat.app.AppCompatActivity; import androidx.appcompat.widget.Toolbar; import android.view.LayoutInflater; import android.view.View; import android.view.Menu; import android.view.MenuItem; import android.widget.DatePicker; import android.widget.EditText; import android.widget.ListView; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity { private List<CostBean> mCostBeanList; private DatabaseHelper mDatabaseHelper; private CostListAdapter mAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = findViewById(R.id.toolbar); setSupportActionBar(toolbar); mDatabaseHelper = new DatabaseHelper(this); mCostBeanList = new ArrayList<>(); ListView costList = findViewById(R.id.lv_main); initCostDate(); mAdapter = new CostListAdapter(this, mCostBeanList); costList.setAdapter(mAdapter); FloatingActionButton fab = findViewById(R.id.fab); fab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this); LayoutInflater inflater = LayoutInflater.from(MainActivity.this); View viewDialog = inflater.inflate(R.layout.new_cost_data,null); final EditText title = viewDialog.findViewById(R.id.et_cost_money); final EditText money = viewDialog.findViewById(R.id.et_cost_title); final DatePicker date = viewDialog.findViewById(R.id.dp_cost_date); builder.setView(viewDialog); builder.setTitle("New Cost"); builder.setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { CostBean costBean = new CostBean(); costBean.costTitle = title.getText().toString(); costBean.costMoney = money.getText().toString(); costBean.costDate = date.getYear() + "-" + (date.getMonth() + 1) + "-" + date.getDayOfMonth(); mDatabaseHelper.insertCost(costBean); mCostBeanList.add(costBean); mAdapter.notifyDataSetChanged(); } }); builder.setNegativeButton("Cancel", null); builder.create().show(); } }); } private void initCostDate() { mDatabaseHelper.deleteAllData(); // for (int i = 0; i < 6; i++) { // CostBean costBean = new CostBean(); // costBean.costTitle = i + "mock"; // costBean.costDate = "11-11"; // costBean.costMoney = "20"; // mDatabaseHelper.insertCost(costBean); // } Cursor cursor = mDatabaseHelper.getAllCostData(); if (cursor != null){ while (cursor.moveToNext()){ CostBean costBean = new CostBean(); costBean.costTitle = cursor.getString(cursor.getColumnIndex("cost_title")); costBean.costDate = cursor.getString(cursor.getColumnIndex("cost_date")); costBean.costMoney = cursor.getString(cursor.getColumnIndex("cost_money")); mCostBeanList.add(costBean); } cursor.close(); } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } }
DatabaseHelper.java
package com.example.myapplicationsecond; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import androidx.annotation.Nullable; public class DatabaseHelper extends SQLiteOpenHelper { public static final String COST_MONEY = "cost_money"; public static final String COST_DATE = "cost_date"; public static final String COST_TITLE = "cost_title"; public static final String IMOOC_COST = "imooc_cost"; public DatabaseHelper(@Nullable Context context) { super(context, "imooc_daily", null, 1); } @Override public void onCreate(SQLiteDatabase db) { db.execSQL("create table if not exists IMOOC_COST("+ "id integer primary key,"+ "cost_title varchar,"+ "cost_date varchar,"+ "cost_money varchar)"); } public void insertCost(CostBean costBean){ SQLiteDatabase database = getWritableDatabase(); ContentValues cv = new ContentValues(); cv.put(COST_TITLE,costBean.costTitle); cv.put(COST_DATE,costBean.costDate); cv.put(COST_MONEY,costBean.costMoney); database.insert(IMOOC_COST,null,cv); } public Cursor getAllCostData(){ SQLiteDatabase database = getWritableDatabase(); return database.query("IMOOC_COST",null,null,null,null,null,COST_DATE + " ASC "); } public void deleteAllData(){ SQLiteDatabase database = getWritableDatabase(); database.delete("IMOOC_COST",null,null); } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { } }
CostListAdapter.java
package com.example.myapplicationsecond; import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseAdapter; import android.widget.TextView; import java.util.List; public class CostListAdapter extends BaseAdapter { private List<CostBean> mList; private Context mContext; private LayoutInflater mLayoutInflater; public CostListAdapter(Context context,List<CostBean> list){ mContext = context; mList = list; mLayoutInflater = LayoutInflater.from(context); } @Override public int getCount() { return mList.size(); } @Override public Object getItem(int position) { return mList.get(position); } @Override public long getItemId(int position) { return position; } @Override public View getView(int position, View convertView, ViewGroup parent) { ViewHolder viewHolder; if(convertView == null){ viewHolder = new ViewHolder(); convertView = mLayoutInflater.inflate(R.layout.list_item,null); viewHolder.mTvCostTitle = convertView.findViewById(R.id.tv_title); viewHolder.mTvCostDate = convertView.findViewById(R.id.tv_date); viewHolder.mTvCostMoney = convertView.findViewById(R.id.tv_cost); convertView.setTag(viewHolder); } else{ viewHolder = (ViewHolder) convertView.getTag(); } CostBean bean = mList.get(position); viewHolder.mTvCostTitle.setText(bean.costTitle); viewHolder.mTvCostDate.setText(bean.costDate); viewHolder.mTvCostMoney.setText(bean.costMoney); return convertView; } private static class ViewHolder{ public TextView mTvCostTitle; public TextView mTvCostDate; public TextView mTvCostMoney; } }
CostBean.java
package com.example.myapplicationsecond; public class CostBean { public String costTitle; public String costDate; public String costMoney; }
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.coordinatorlayout.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <com.google.android.material.appbar.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:theme="@style/AppTheme.AppBarOverlay"> <androidx.appcompat.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:popupTheme="@style/AppTheme.PopupOverlay" /> </com.google.android.material.appbar.AppBarLayout> <include layout="@layout/content_main" /> <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/fab" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="bottom|end" android:layout_margin="@dimen/fab_margin" app:srcCompat="@android:drawable/ic_dialog_email" /> </androidx.coordinatorlayout.widget.CoordinatorLayout>
content_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context=".MainActivity" tools:showIn="@layout/activity_main"> <ListView android:id="@+id/lv_main" android:layout_width="match_parent" android:layout_height="match_parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
list_item.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="80dp"> <TextView android:id="@+id/tv_title" android:layout_width="200dp" android:layout_height="80dp" android:layout_marginLeft="10dp" android:layout_alignParentLeft="true" android:gravity="center" android:singleLine="true" android:textSize="35sp" android:ellipsize="marquee" android:text="costTitle"/> <TextView android:id="@+id/tv_date" android:layout_width="wrap_content" android:layout_height="80dp" android:textSize="20sp" android:gravity="center" android:layout_marginLeft="15dp" android:layout_toRightOf="@id/tv_title" android:text="costDate"/> <TextView android:id="@+id/tv_cost" android:layout_width="wrap_content" android:layout_height="80dp" android:gravity="center" android:layout_alignParentRight="true" android:layout_marginRight="20dp" android:text="30" android:textSize="30sp"/> </RelativeLayout>
new_cost_data.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:gravity="center" android:layout_width="match_parent" android:layout_height="match_parent"> <EditText android:id="@+id/et_cost_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="4dp" android:hint="Cost Money"/> <EditText android:id="@+id/et_cost_money" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="4dp" android:hint="Cost Title"/> <DatePicker android:id="@+id/dp_cost_date" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="4dp" android:datePickerMode="spinner" android:calendarViewShown="false"/> </LinearLayout>
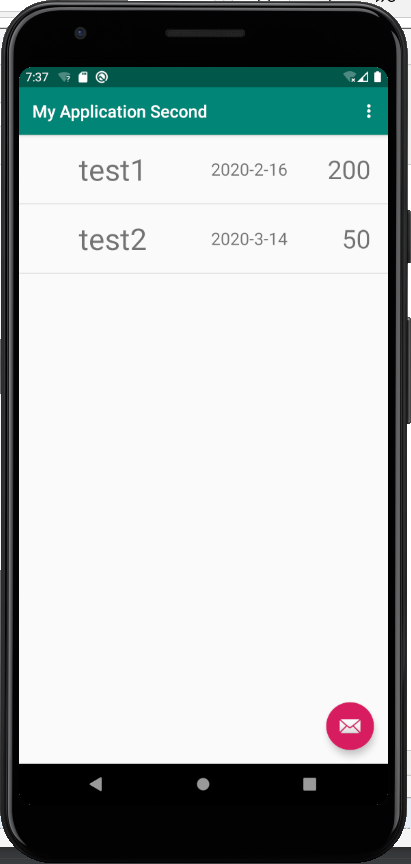
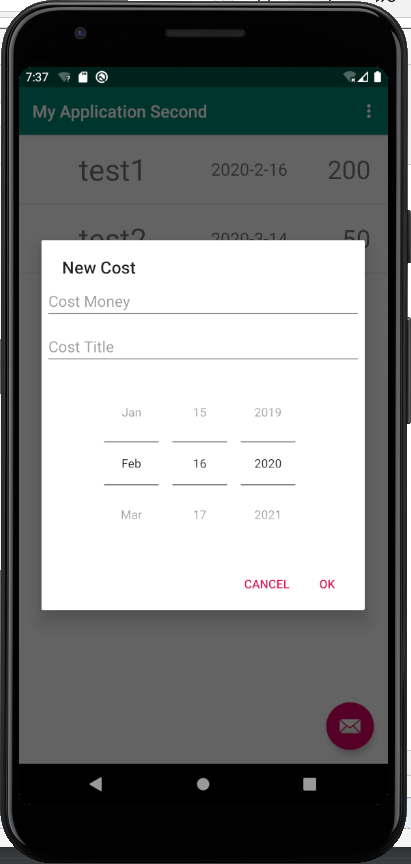