1、、使用到的jar包,为apache的一个子项目


2、页面展示fileUpload.jsp
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'fileUpload.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="UploadServlet" method="post" enctype="multipart/form-data">
username: <input type="text" name="username"><br>
file: <input type="file" name="file"><br>
file2: <input type="file" name="file2"><br>
<input type="submit" value="submit">
</form>3、servlet处理UploadServlet.javapackage com.shengsiyuan.servlet;
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
public class UploadServlet extends HttpServlet
{
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException
{
DiskFileItemFactory factory = new DiskFileItemFactory();
String path = req.getRealPath("/upload");//定义上传文件的路径为webroot下的upload文件
factory.setRepository(new File(path));
factory.setSizeThreshold(1024 * 1024);//上传文件的大小为1024*1024字节,也就是1兆
ServletFileUpload upload = new ServletFileUpload(factory);
try
{
List<FileItem> list = (List<FileItem>)upload.parseRequest(req);
for(FileItem item : list)
{
String name = item.getFieldName();
if(item.isFormField())//如果上传的是文本域的话,对文本与进行处理
{
String value = item.getString();
System.out.println(name + "=" + value);
req.setAttribute(name, value);
}
else//如果上传的是文件
{
String value = item.getName();
int start = value.lastIndexOf("\");//查找文件名称,从最后一个开始查找
String fileName = value.substring(start + 1);//这时候对应的文件应该从1开始,因为0是
req.setAttribute(name, fileName);
item.write(new File(path, fileName));//将文件写到磁盘上来,path为上传的路径,filename为文件名称 //以下是自己写的操作输入输出流的代码,当然也可以不使用下面的写法
//
// OutputStream os = new FileOutputStream(new File(path, fileName));
//
// InputStream is = item.getInputStream();
//
// byte[] buffer = new byte[400];
//
// int length = 0;
//
// while((length = is.read(buffer)) != -1)
// {
// os.write(buffer, 0, length);
// }
//
// is.close();
// os.close();
}
}
}
catch(Exception ex)
{
ex.printStackTrace();
}
req.getRequestDispatcher("fileUploadResult.jsp").forward(req, resp);
}
}4、结果页fileUploadResult.jsp<%@ page language="java" import="java.util.*, java.io.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'fileUploadResult.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<%
/*
InputStream is = request.getInputStream();
BufferedReader br = new BufferedReader(new InputStreamReader(is));
String buffer = null;
while(null != (buffer = br.readLine()))
{
out.print(buffer + "<br>");
}
br.close();
is.close();
*/
%>
username : ${requestScope.username }<br>
file: ${requestScope.file}<br>
file2: ${requestScope.file2 }<br>
</body>
</html>5、页面展示
结果展示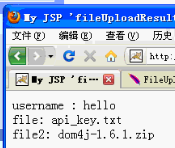