Strategic Game
Time Limit: 20000/10000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Description
Bob enjoys playing computer games, especially strategic games, but sometimes he cannot find the solution fast enough and then he is very sad. Now he has the following problem. He must defend a medieval city, the roads of which form a tree. He has to put the minimum number of soldiers on the nodes so that they can observe all the edges. Can you help him?
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
The input file contains several data sets in text format. Each data set represents a tree with the following description:
the number of nodes
the description of each node in the following format
node_identifier:(number_of_roads) node_identifier1 node_identifier2 ... node_identifier
or
node_identifier:(0)
The node identifiers are integer numbers between 0 and n-1, for n nodes (0 < n <= 1500). Every edge appears only once in the input data.
For example for the tree:
the solution is one soldier ( at the node 1).
The output should be printed on the standard output. For each given input data set, print one integer number in a single line that gives the result (the minimum number of soldiers). An example is given in the following table:
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
The input file contains several data sets in text format. Each data set represents a tree with the following description:
the number of nodes
the description of each node in the following format
node_identifier:(number_of_roads) node_identifier1 node_identifier2 ... node_identifier
or
node_identifier:(0)
The node identifiers are integer numbers between 0 and n-1, for n nodes (0 < n <= 1500). Every edge appears only once in the input data.
For example for the tree:
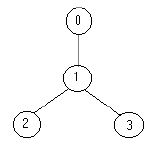
the solution is one soldier ( at the node 1).
The output should be printed on the standard output. For each given input data set, print one integer number in a single line that gives the result (the minimum number of soldiers). An example is given in the following table:
Sample Input
4
0:(1) 1
1:(2) 2 3
2:(0)
3:(0)
5
3:(3) 1 4 2
1:(1) 0
2:(0)
0:(0)
4:(0)
Sample Output
1 2
题意:给你一棵树,每个节点都需要被士兵看管,一个士兵可以看管他所在的节点和与这个节点相邻的节点,问最少要多少个士兵才能看到所有的节点
分析:二分图最小点覆盖,就是最大匹配。由于点比较多,使用匈牙利算法的邻接矩阵形式会超时,用邻接表就行了。当然也可以用Hopcroft-Carp算法
#include<stdio.h> #include<string.h> #include<iostream> using namespace std; const int MAXN = 2000; struct Edge { int to,next; }edge[MAXN*2]; int head[MAXN],tol; int n; int linker[MAXN]; bool used[MAXN]; void init() { tol=0; memset(head,-1,sizeof(head)); } void addedge(int u,int v) { edge[tol].to=v; edge[tol].next=head[u]; head[u]=tol++; } bool dfs(int u) { for(int i=head[u];i!=-1;i=edge[i].next) { int v=edge[i].to; if(!used[v]) { used[v]=true; if(linker[v]==-1||dfs(linker[v])) { linker[v]=u; return true; } } } return false; } int Hungary() { int res=0; memset(linker,-1,sizeof(linker)); for(int u=0;u<n;u++) { memset(used,false,sizeof(used)); if(dfs(u)) res++; } return res; } int main() { int u,v,t; while(scanf("%d",&n)==1&&n) { init(); for(u=0;u<n;u++) { scanf("%d:(%d)",&u,&t); for(int i=1;i<=t;i++) { scanf("%d",&v); addedge(u,v); addedge(v,u); } } printf("%d ",Hungary()/2); } return 0; }