先放运行界面: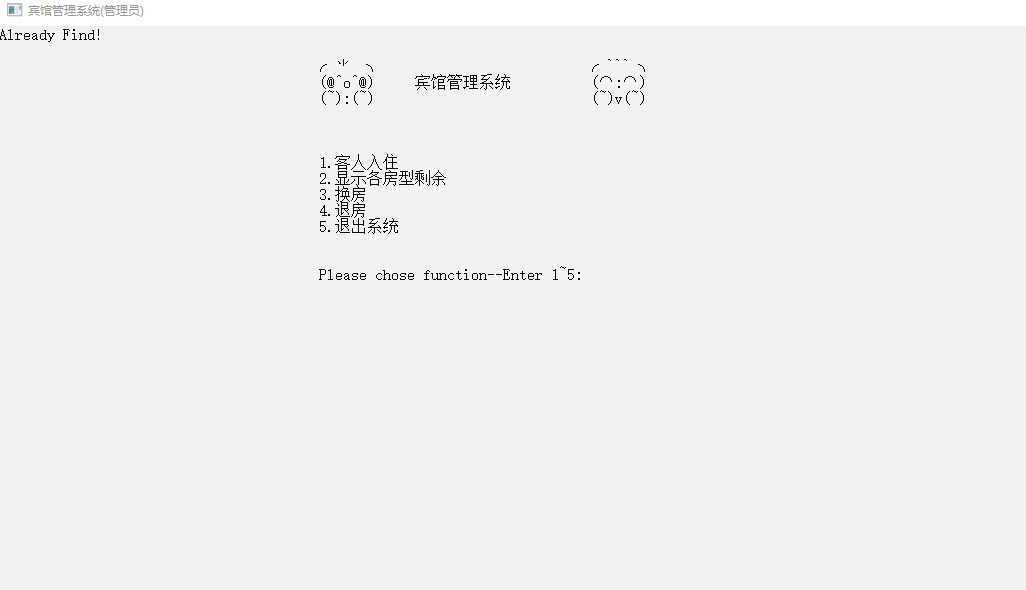
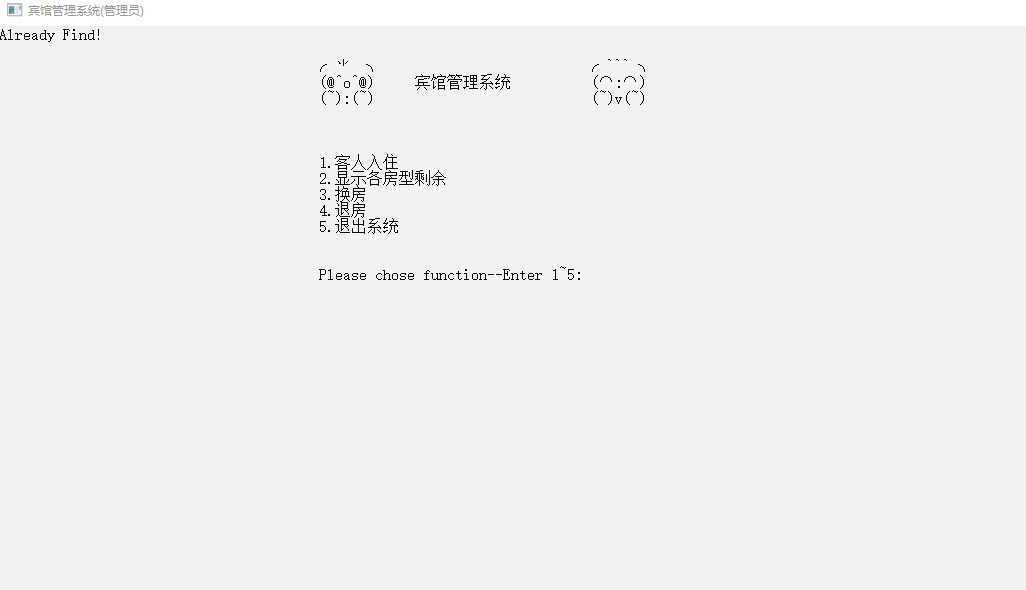
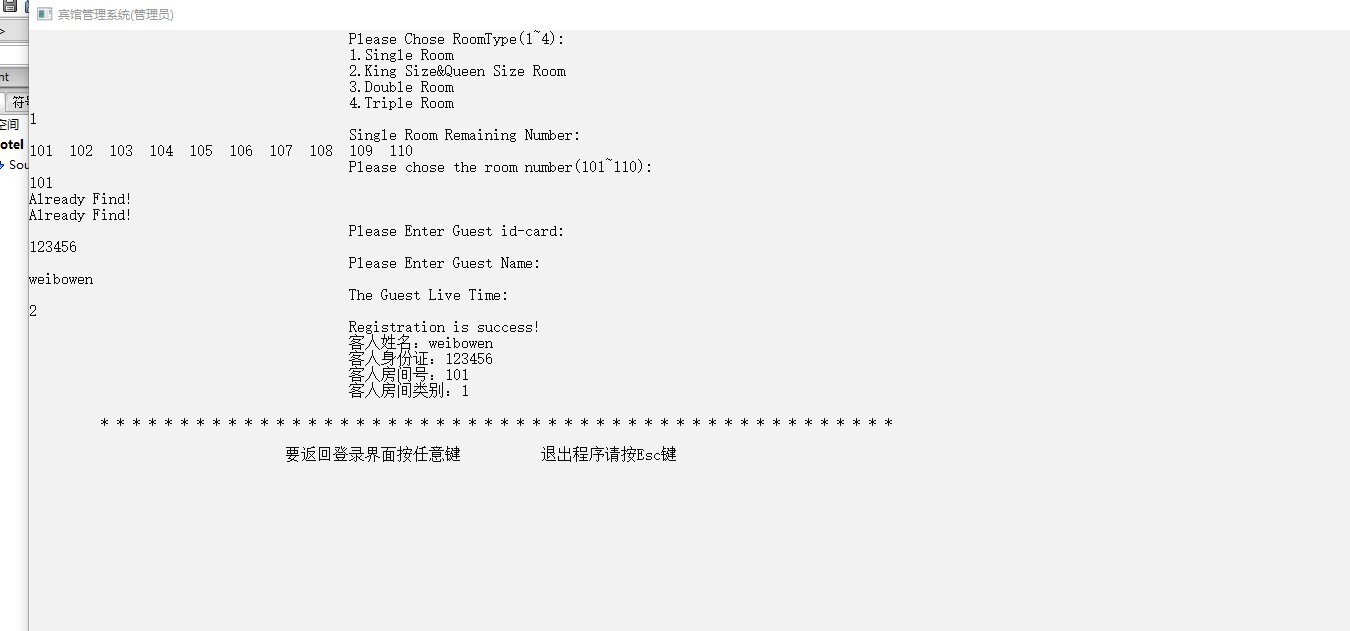
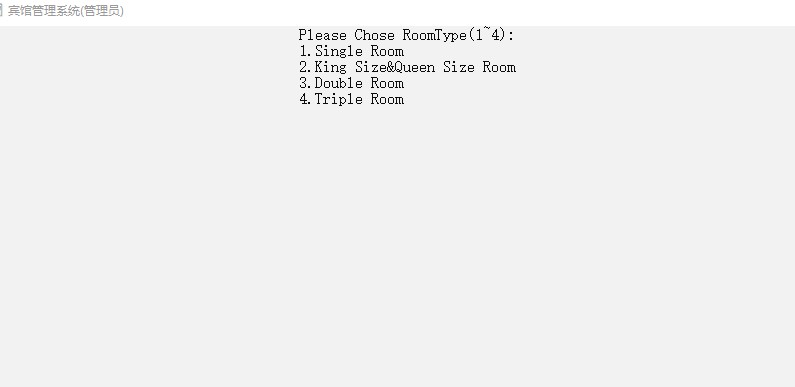
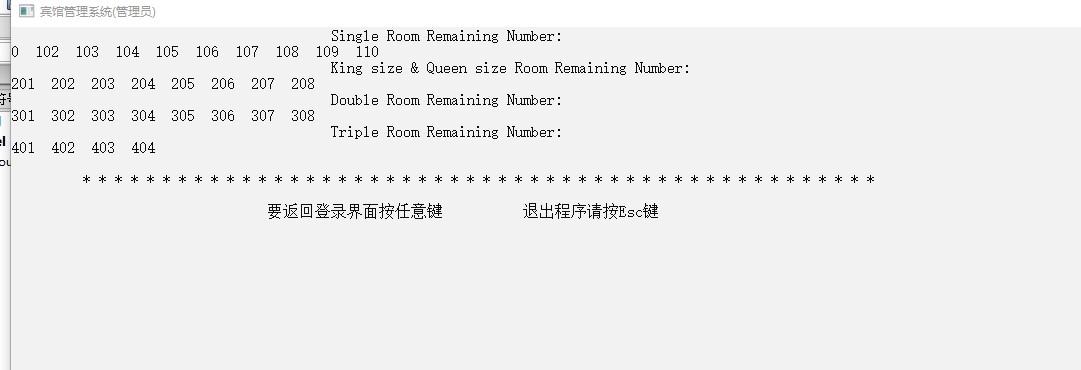
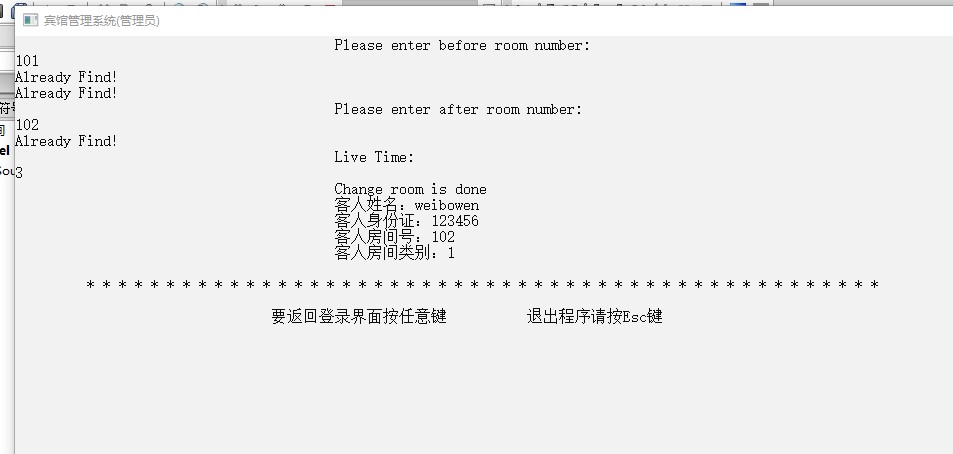
需求分析:客人登记入住,显示空房信息,显示客人信息,客人换房,客人退房结算,退出系统。
房间种类:大床房,单人间,双人间,三人间。
房间数:30.
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <string.h> 4 #include<conio.h> 5 #include<Windows.h> 6 7 int type[4] = {1,2,3,4}; //用来存储宾馆房型的种类1~4 8 int cost[6] = {100,118,122,125,134,168}; //宾馆房间的价格 9 int num[4] = {101,201,301,401}; //初始的每楼的房间号 10 int rm[30]; //用来储存空房间号,因为我设的总房间数是三十,所以这个数组长度就是三十 11 char cr1[7]; //cr1~cr7都是用来存储要求换房的客人信息的临时储存信息。就像A->B->C. B就是一个媒介。 12 char cr2[10]; 13 char cr3[7]; 14 char cr4[10]; 15 char cr5[7]; 16 char cr6[10]; 17 18 struct User //用户信息结构体 19 { 20 char idcard[18]; //身份证号 21 char name[10]; //姓名 22 int roomnumber; //入住房间号 23 int roomtype; //房间类型 24 }; 25 26 struct Room //房间信息结构体 27 { 28 int number; //房间号 29 int rtype; //房间种类 30 struct User guest[3]; //客人信息结构体数组 31 double price; //房间价格 32 int isIn; //是否为空 33 int time; //这个房间被占用时间=客人住这个房间多长时间。客人可能会换房 34 struct Room *next; //方便构建链表 35 }; 36 37 struct Hotel //宾馆信息结构体 38 { 39 int Allroom; //所有房间数=30 40 int isNull; //是否住满 41 double income; //收益 42 double deposit; //客人押金 43 }; 44 45 void Menu(struct Room *L,struct Hotel h); //主菜单函数声明 46 struct Room *GetRoom(struct Room *L,int n); //定位房间声明
以上是头文件,全局变量,结构体,函数声明等等的定义。
接下来是一堆函数。懒得注释了。
struct Room * InitialHotel(struct Hotel h) { h.Allroom = 30; h.isNull = 1; h.income = 0; h.deposit = 0; int i; struct Room *p,*L,*tail; p=tail=(struct Room *)malloc(sizeof(struct Room)); L=NULL; for(i=1;i<=h.Allroom;i++) { rm[i-1] = 0; p->isIn = 0; p->time = 0; if(i==1) //1 { p->number=num[0]; p->rtype=type[0]; p->price=cost[0]; num[0]++; L = p; } else { tail->next=p; if(i>=2&&i<=10) //9 { p->number=num[0]; p->rtype=type[0]; p->price=cost[0]; num[0]++; } else if(i<=18&&i>=11) //8 { p->number = num[1]; p->rtype = type[1]; p->price = cost[1]; num[1]++; } else if(i<=26&&i>=19) //8 { p->number = num[2]; p->rtype = type[2]; p->price = cost[2]; num[2]++; } else //4 { p->number = num[3]; p->rtype = type[3]; p->price = cost[5]; num[3]++; } } tail = p; p=(struct Room *)malloc(sizeof(struct Room)); } tail->next=L; return (L); } void Remaining(struct Room *L) { struct Room *p; int i; p=GetRoom(L,101); int count[4]={0,0,0,0}; for(i=1;i<=30;i++) { rm[i-1]= 0; if(i<=10) { if(p->isIn==0) { count[0]++; //single rm[i-1]=p->number; } } else if(i>=11&&i<=18) { if(p->isIn==0) { count[1]++; //king size&queen size room rm[i-1]=p->number; } } else if(i>=19&&i<=26) { if(p->isIn==0) { count[2]++; //double room rm[i-1]=p->number; } } else { if(p->isIn==0) { count[3]++; //triple room rm[i-1]=p->number; } } p=p->next; } /*char *str[4]={ "Single Room Remaining", "King size & Queen size Room Remaining", "Double Room Remaining", "Triple Room Remaining" }; for(i=0;i<4;i++) {printf("%s:%d ",str[i],count[i]);}*/ } struct Room *GetRoom(struct Room *L,int n) //n is room number that guest wants. { struct Room *p; p=L; int j = 1; for(j=1;j<=30;j++) { if(p->number==n) { L=p; j=31; } p=p->next; } /*if(p==NULL||p->number!=n) return NULL;*/ printf("Already Find! "); return (L); } struct Room * EnterIfo(struct Room *L) { struct Room *p; p=L; int flag = 1; int i,j; if(p->rtype==1) { p->isIn=1; Remaining(p); int f=1; while(f) { printf(" Please Enter Guest id-card: "); scanf("%s",p->guest[0].idcard); if(strlen(p->guest[0].idcard)==18) {f=0;} else { printf("请重新输入客户身份证号: "); f=1; } } printf(" Please Enter Guest Name: "); scanf("%s",p->guest[0].name); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; printf(" The Guest Live Time: "); scanf("%d",&j); p->time = j; } else { p->isIn =1; Remaining(p); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; /* for(k=0;k<=30;k++) { if(rm[k-1]==p->guest[0].roomnumber) rm[k-1]=0; }*/ while(flag) { printf(" Please Enter Guest id-card: "); scanf("%s",p->guest[flag-1].idcard); //gets(p->guest[flag-1].idcard); printf(" Please Enter Guest Name: "); scanf("%s",p->guest[flag-1].name); //gets(p->guest[flag-1].name); printf(" Continue or not?Enter 0 (no)or 1(yes) "); scanf("%d",&i); if(i==0) flag = i; if(i==1) { flag++; printf(" The Guest Live Time: "); scanf("%d",&j); if(flag==2) p->time = j; if(flag==3) p->time = j; } } } return (p); } void RoomInfo(int types) { int j; switch(types) { case 1: printf(" Single Room Remaining Number: "); for(j=1;j<=10;j++) {printf("%d ",rm[j-1]);} printf(" "); break; case 2: printf(" King size & Queen size Room Remaining Number: "); for(j=11;j<=18;j++) {printf("%d ",rm[j-1]);} printf(" "); break; case 3: printf(" Double Room Remaining Number: "); for(j=19;j<=26;j++) {printf("%d ",rm[j-1]);} printf(" "); break; case 4: printf(" Triple Room Remaining Number: "); for(j=27;j<=30;j++) {printf("%d ",rm[j-1]);} printf(" "); break; default: printf(" Not Found The Type Room!"); } } struct Room * Registration(struct Room *L,struct Hotel h) { struct Room *p; p=L; printf(" Please Chose RoomType(1~4): "); printf(" 1.Single Room "); printf(" 2.King Size&Queen Size Room "); printf(" 3.Double Room "); printf(" 4.Triple Room "); int i,n; scanf("%d",&i); switch(i) { h.deposit+=100; case 1: //Remaining(L); RoomInfo(1); printf(" Please chose the room number(101~110): "); scanf("%d",&n); p=GetRoom(p,n); L=EnterIfo(p); printf(" Registration is success! "); break; case 2: //Remaining(L); RoomInfo(2); printf(" Please chose the room number(201~208): "); scanf("%d",&n); p=GetRoom(L,n); L=EnterIfo(p); printf(" Registration is success! "); break; case 3: // Remaining(L); RoomInfo(3); printf(" Please chose the room number(301~308): "); scanf("%d",&n); p=GetRoom(L,n); L=EnterIfo(p); printf(" Registration is success! "); break; case 4: // Remaining(L); RoomInfo(4); printf(" Please chose the room number(401~404): "); scanf("%d",&n); p=GetRoom(L,n); L=EnterIfo(p); printf(" Registration is success! "); break; default: printf(" Your Enter is Wrong!"); } printf(" 客人姓名:%s ",p->guest[0].name); printf(" 客人身份证:%s ",p->guest[0].idcard); printf(" 客人房间号:%d ",p->guest[0].roomnumber); printf(" 客人房间类别:%d ",p->guest[0].roomtype); return (L); } struct Room * CheckOut(struct Room *L,struct Hotel h,int n,int b) { struct Room *p; p=L; int i=0; if(b==1) { p = GetRoom(L,n); p->isIn=0; h.income=(p->time)*(p->price)-100; h.deposit-=100; p->time = 0; if(p->rtype==1) { strcpy(p->guest[0].idcard,"nobody"); strcpy(p->guest[0].name,"nobody"); p->guest[0].roomnumber = 0; p->guest[0].roomtype = 0; } else { for(i=0;i<3;i++) { strcpy(p->guest[i].idcard,"nobody"); strcpy(p->guest[i].name,"nobody"); p->guest[i].roomnumber = 0; p->guest[i].roomtype = 0; } } Remaining(L); printf("收益:%f ",h.income); printf("退房成功! "); } if(b==0) { p = GetRoom(L,n); printf(" 客人姓名:%s ",p->guest[0].name); printf(" 客人身份证:%s ",p->guest[0].idcard); printf(" 客人房间号:%d ",p->guest[0].roomnumber); printf(" 客人房间类别:%d ",p->guest[0].roomtype); p->isIn=0; h.income=(p->time)*(p->price); p->time = 0; if(p->rtype==1) { strcpy(cr1,p->guest[0].idcard); strcpy(cr2, p->guest[0].name); strcpy(p->guest[0].idcard,"nobody"); strcpy(p->guest[0].name,"nobody"); p->guest[0].roomnumber = 0; p->guest[0].roomtype = 0; } else { strcpy(cr1,p->guest[0].idcard); strcpy(cr2, p->guest[0].name); strcpy(p->guest[0].idcard,"nobody"); strcpy(p->guest[0].name,"nobody"); p->guest[0].roomnumber = 0; p->guest[0].roomtype = 0; strcpy(cr3,p->guest[1].idcard); strcpy(cr4, p->guest[1].name); strcpy(p->guest[1].idcard,"nobody"); strcpy(p->guest[1].name,"nobody"); strcpy(cr5,p->guest[2].idcard); strcpy(cr6, p->guest[2].name); strcpy(p->guest[2].idcard,"nobody"); strcpy(p->guest[2].name,"nobody"); } /* for(i=1;i<=30;i++) { if(rm[i-1]==0) rm[i-1]=p->number; }*/ Remaining(L); } L = p; return (L); } struct Room * Change(struct Room *L,struct Hotel h) { struct Room *p; p = L; int i=0,j=0; printf(" Please enter before room number: "); scanf("%d",&i); p=CheckOut(L,h,i,0); printf(" Please enter after room number: "); scanf("%d",&j); p=GetRoom(L,j); if(p->rtype==1) { strcpy(p->guest[0].idcard,cr1); strcpy(p->guest[0].name,cr2); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; } else { strcpy(p->guest[0].idcard,cr1); strcpy(p->guest[0].name,cr2); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; strcpy(p->guest[1].idcard,cr3); strcpy(p->guest[1].name,cr4); strcpy(p->guest[2].idcard,cr5); strcpy(p->guest[2].name,cr6); } p->isIn = 1; printf(" Live Time: "); scanf("%d",&i); p->time = i; printf(" Change room is done "); printf(" 客人姓名:%s ",p->guest[0].name); printf(" 客人身份证:%s ",p->guest[0].idcard); printf(" 客人房间号:%d ",p->guest[0].roomnumber); printf(" 客人房间类别:%d ",p->guest[0].roomtype); L = p; return (L); } void Quit(struct Room *L,struct Hotel h) { char ch; int i; printf(" "); for (i = 0; i < 50; i++) { printf("* "); Sleep(10); } printf(" 要返回登录界面按任意键 退出程序请按Esc键 "); ch = getch(); if (ch == 0x1b) //esc键按下 { _exit(0); } system("cls"); Menu(L,h); } void Menu(struct Room *L,struct Hotel h) { int i; struct Room *p; p=L; SetConsoleTitle("宾馆管理系统(管理员)"); system("color f0"); printf(" "); printf(" ╭ � % ╮ ╭ ```╮ "); printf(" (@^o^@) 宾馆管理系统 (⌒:⌒) "); printf(" (~):(~) (~)v(~) "); printf(" "); //printf(" "); printf(" 1.客人入住 "); printf(" 2.显示各房型剩余 "); printf(" 3.换房 "); printf(" 4.退房 "); printf(" 5.退出系统 "); printf(" "); printf(" Please chose function--Enter 1~5: "); scanf("%d",&i); switch(i) { case 1: system("cls"); p=Registration(p,h); Quit(p,h); break; case 2: Remaining(L); system("cls"); int j=0; for(j=1;j<=4;j++) { RoomInfo(j); } Quit(p,h); break; case 3: system("cls"); L=Change(p,h); Quit(p,h); break; case 4: system("cls"); printf("Check Out Number: "); int n; scanf("%d",&n); p=CheckOut(p,h,n,1); Quit(p,h); break; case 5: system("cls"); Quit(p,h); break; default: printf(" Wrong Number! "); } }
主函数调用:
int main() { struct Room *L; struct Hotel h; L=InitialHotel(h); Remaining(L); Menu(L,h); return 0; }
附完整代码:
#include <stdio.h> #include <stdlib.h> #include <string.h> #include<conio.h> #include<Windows.h> int type[4] = {1,2,3,4}; int cost[6] = {100,118,122,125,134,168}; int num[4] = {101,201,301,401}; int rm[30]; char cr1[7]; char cr2[10]; char cr3[7]; char cr4[10]; char cr5[7]; char cr6[10]; struct User { char idcard[18]; char name[10]; int roomnumber; int roomtype; }; struct Room { int number; int rtype; struct User guest[3]; double price; int isIn; int time; struct Room *next; }; struct Hotel { int Allroom; int isNull; double income; double deposit; }; void Menu(struct Room *L,struct Hotel h); struct Room *GetRoom(struct Room *L,int n); struct Room * InitialHotel(struct Hotel h) { h.Allroom = 30; h.isNull = 1; h.income = 0; h.deposit = 0; int i; struct Room *p,*L,*tail; p=tail=(struct Room *)malloc(sizeof(struct Room)); L=NULL; for(i=1;i<=h.Allroom;i++) { rm[i-1] = 0; p->isIn = 0; p->time = 0; if(i==1) //1 { p->number=num[0]; p->rtype=type[0]; p->price=cost[0]; num[0]++; L = p; } else { tail->next=p; if(i>=2&&i<=10) //9 { p->number=num[0]; p->rtype=type[0]; p->price=cost[0]; num[0]++; } else if(i<=18&&i>=11) //8 { p->number = num[1]; p->rtype = type[1]; p->price = cost[1]; num[1]++; } else if(i<=26&&i>=19) //8 { p->number = num[2]; p->rtype = type[2]; p->price = cost[2]; num[2]++; } else //4 { p->number = num[3]; p->rtype = type[3]; p->price = cost[5]; num[3]++; } } tail = p; p=(struct Room *)malloc(sizeof(struct Room)); } tail->next=L; return (L); } void Remaining(struct Room *L) { struct Room *p; int i; p=GetRoom(L,101); int count[4]={0,0,0,0}; for(i=1;i<=30;i++) { rm[i-1]= 0; if(i<=10) { if(p->isIn==0) { count[0]++; //single rm[i-1]=p->number; } } else if(i>=11&&i<=18) { if(p->isIn==0) { count[1]++; //king size&queen size room rm[i-1]=p->number; } } else if(i>=19&&i<=26) { if(p->isIn==0) { count[2]++; //double room rm[i-1]=p->number; } } else { if(p->isIn==0) { count[3]++; //triple room rm[i-1]=p->number; } } p=p->next; } /*char *str[4]={ "Single Room Remaining", "King size & Queen size Room Remaining", "Double Room Remaining", "Triple Room Remaining" }; for(i=0;i<4;i++) {printf("%s:%d ",str[i],count[i]);}*/ } struct Room *GetRoom(struct Room *L,int n) //n is room number that guest wants. { struct Room *p; p=L; int j = 1; for(j=1;j<=30;j++) { if(p->number==n) { L=p; j=31; } p=p->next; } /*if(p==NULL||p->number!=n) return NULL;*/ printf("Already Find! "); return (L); } struct Room * EnterIfo(struct Room *L) { struct Room *p; p=L; int flag = 1; int i,j; if(p->rtype==1) { p->isIn=1; Remaining(p); int f=1; while(f) { printf(" Please Enter Guest id-card: "); scanf("%s",p->guest[0].idcard); if(strlen(p->guest[0].idcard)==18) {f=0;} else { printf("请重新输入客户身份证号: "); f=1; } } printf(" Please Enter Guest Name: "); scanf("%s",p->guest[0].name); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; printf(" The Guest Live Time: "); scanf("%d",&j); p->time = j; } else { p->isIn =1; Remaining(p); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; /* for(k=0;k<=30;k++) { if(rm[k-1]==p->guest[0].roomnumber) rm[k-1]=0; }*/ while(flag) { printf(" Please Enter Guest id-card: "); scanf("%s",p->guest[flag-1].idcard); //gets(p->guest[flag-1].idcard); printf(" Please Enter Guest Name: "); scanf("%s",p->guest[flag-1].name); //gets(p->guest[flag-1].name); printf(" Continue or not?Enter 0 (no)or 1(yes) "); scanf("%d",&i); if(i==0) flag = i; if(i==1) { flag++; printf(" The Guest Live Time: "); scanf("%d",&j); if(flag==2) p->time = j; if(flag==3) p->time = j; } } } return (p); } void RoomInfo(int types) { int j; switch(types) { case 1: printf(" Single Room Remaining Number: "); for(j=1;j<=10;j++) {printf("%d ",rm[j-1]);} printf(" "); break; case 2: printf(" King size & Queen size Room Remaining Number: "); for(j=11;j<=18;j++) {printf("%d ",rm[j-1]);} printf(" "); break; case 3: printf(" Double Room Remaining Number: "); for(j=19;j<=26;j++) {printf("%d ",rm[j-1]);} printf(" "); break; case 4: printf(" Triple Room Remaining Number: "); for(j=27;j<=30;j++) {printf("%d ",rm[j-1]);} printf(" "); break; default: printf(" Not Found The Type Room!"); } } struct Room * Registration(struct Room *L,struct Hotel h) { struct Room *p; p=L; printf(" Please Chose RoomType(1~4): "); printf(" 1.Single Room "); printf(" 2.King Size&Queen Size Room "); printf(" 3.Double Room "); printf(" 4.Triple Room "); int i,n; scanf("%d",&i); switch(i) { h.deposit+=100; case 1: //Remaining(L); RoomInfo(1); printf(" Please chose the room number(101~110): "); scanf("%d",&n); p=GetRoom(p,n); L=EnterIfo(p); printf(" Registration is success! "); break; case 2: //Remaining(L); RoomInfo(2); printf(" Please chose the room number(201~208): "); scanf("%d",&n); p=GetRoom(L,n); L=EnterIfo(p); printf(" Registration is success! "); break; case 3: // Remaining(L); RoomInfo(3); printf(" Please chose the room number(301~308): "); scanf("%d",&n); p=GetRoom(L,n); L=EnterIfo(p); printf(" Registration is success! "); break; case 4: // Remaining(L); RoomInfo(4); printf(" Please chose the room number(401~404): "); scanf("%d",&n); p=GetRoom(L,n); L=EnterIfo(p); printf(" Registration is success! "); break; default: printf(" Your Enter is Wrong!"); } printf(" 客人姓名:%s ",p->guest[0].name); printf(" 客人身份证:%s ",p->guest[0].idcard); printf(" 客人房间号:%d ",p->guest[0].roomnumber); printf(" 客人房间类别:%d ",p->guest[0].roomtype); return (L); } struct Room * CheckOut(struct Room *L,struct Hotel h,int n,int b) { struct Room *p; p=L; int i=0; if(b==1) { p = GetRoom(L,n); p->isIn=0; h.income=(p->time)*(p->price)-100; h.deposit-=100; p->time = 0; if(p->rtype==1) { strcpy(p->guest[0].idcard,"nobody"); strcpy(p->guest[0].name,"nobody"); p->guest[0].roomnumber = 0; p->guest[0].roomtype = 0; } else { for(i=0;i<3;i++) { strcpy(p->guest[i].idcard,"nobody"); strcpy(p->guest[i].name,"nobody"); p->guest[i].roomnumber = 0; p->guest[i].roomtype = 0; } } Remaining(L); printf("收益:%f ",h.income); printf("退房成功! "); } if(b==0) { p = GetRoom(L,n); printf(" 客人姓名:%s ",p->guest[0].name); printf(" 客人身份证:%s ",p->guest[0].idcard); printf(" 客人房间号:%d ",p->guest[0].roomnumber); printf(" 客人房间类别:%d ",p->guest[0].roomtype); p->isIn=0; h.income=(p->time)*(p->price); p->time = 0; if(p->rtype==1) { strcpy(cr1,p->guest[0].idcard); strcpy(cr2, p->guest[0].name); strcpy(p->guest[0].idcard,"nobody"); strcpy(p->guest[0].name,"nobody"); p->guest[0].roomnumber = 0; p->guest[0].roomtype = 0; } else { strcpy(cr1,p->guest[0].idcard); strcpy(cr2, p->guest[0].name); strcpy(p->guest[0].idcard,"nobody"); strcpy(p->guest[0].name,"nobody"); p->guest[0].roomnumber = 0; p->guest[0].roomtype = 0; strcpy(cr3,p->guest[1].idcard); strcpy(cr4, p->guest[1].name); strcpy(p->guest[1].idcard,"nobody"); strcpy(p->guest[1].name,"nobody"); strcpy(cr5,p->guest[2].idcard); strcpy(cr6, p->guest[2].name); strcpy(p->guest[2].idcard,"nobody"); strcpy(p->guest[2].name,"nobody"); } /* for(i=1;i<=30;i++) { if(rm[i-1]==0) rm[i-1]=p->number; }*/ Remaining(L); } L = p; return (L); } struct Room * Change(struct Room *L,struct Hotel h) { struct Room *p; p = L; int i=0,j=0; printf(" Please enter before room number: "); scanf("%d",&i); p=CheckOut(L,h,i,0); printf(" Please enter after room number: "); scanf("%d",&j); p=GetRoom(L,j); if(p->rtype==1) { strcpy(p->guest[0].idcard,cr1); strcpy(p->guest[0].name,cr2); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; } else { strcpy(p->guest[0].idcard,cr1); strcpy(p->guest[0].name,cr2); p->guest[0].roomnumber = p->number; p->guest[0].roomtype = p->rtype; strcpy(p->guest[1].idcard,cr3); strcpy(p->guest[1].name,cr4); strcpy(p->guest[2].idcard,cr5); strcpy(p->guest[2].name,cr6); } p->isIn = 1; printf(" Live Time: "); scanf("%d",&i); p->time = i; printf(" Change room is done "); printf(" 客人姓名:%s ",p->guest[0].name); printf(" 客人身份证:%s ",p->guest[0].idcard); printf(" 客人房间号:%d ",p->guest[0].roomnumber); printf(" 客人房间类别:%d ",p->guest[0].roomtype); L = p; return (L); } void Quit(struct Room *L,struct Hotel h) { char ch; int i; printf(" "); for (i = 0; i < 50; i++) { printf("* "); Sleep(10); } printf(" 要返回登录界面按任意键 退出程序请按Esc键 "); ch = getch(); if (ch == 0x1b) //esc键按下 { _exit(0); } system("cls"); Menu(L,h); } void Menu(struct Room *L,struct Hotel h) { int i; struct Room *p; p=L; SetConsoleTitle("宾馆管理系统(管理员)"); system("color f0"); printf(" "); printf(" ╭ � % ╮ ╭ ```╮ "); printf(" (@^o^@) 宾馆管理系统 (⌒:⌒) "); printf(" (~):(~) (~)v(~) "); printf(" "); //printf(" "); printf(" 1.客人入住 "); printf(" 2.显示各房型剩余 "); printf(" 3.换房 "); printf(" 4.退房 "); printf(" 5.退出系统 "); printf(" "); printf(" Please chose function--Enter 1~5: "); scanf("%d",&i); switch(i) { case 1: system("cls"); p=Registration(p,h); Quit(p,h); break; case 2: Remaining(L); system("cls"); int j=0; for(j=1;j<=4;j++) { RoomInfo(j); } Quit(p,h); break; case 3: system("cls"); L=Change(p,h); Quit(p,h); break; case 4: system("cls"); printf("Check Out Number: "); int n; scanf("%d",&n); p=CheckOut(p,h,n,1); Quit(p,h); break; case 5: system("cls"); Quit(p,h); break; default: printf(" Wrong Number! "); } } int main() { struct Room *L; struct Hotel h; L=InitialHotel(h); Remaining(L); Menu(L,h); return 0; }