#include "stdafx.h"
#include <Windows.h>
#include <iostream>
using namespace std;
/************************************
@ Brief: 打开注册表,读取Key对应value
@ Author: woniu201
@ Created: 2018/09/07
@ Return:
************************************/
int ReadReg(char* path, char* key, char* value)
{
HKEY hKey;
int ret = RegOpenKeyEx(HKEY_CURRENT_USER, path, 0, KEY_EXECUTE, &hKey);
if (ret != ERROR_SUCCESS)
{
cout << "打开注册表失败" << endl;
return 1;
}
//读取KEY
DWORD dwType = REG_SZ; //数据类型
DWORD cbData = 256;
ret = RegQueryValueEx(hKey, key, NULL, &dwType, (LPBYTE)value, &cbData);
if (ret == ERROR_SUCCESS)
{
cout << value << endl;
}
else
{
cout << "读取注册表中KEY 失败" << endl;
RegCloseKey(hKey);
return 1;
}
RegCloseKey(hKey);
return 0;
}
/************************************
@ Brief: 写注册表,如不存在自动创建
@ Author: woniu201
@ Created: 2018/09/07
@ Return:
************************************/
int WriteReg(char* path, char* key, char* value)
{
HKEY hKey;
DWORD dwDisp;
DWORD dwType = REG_SZ; //数据类型
int ret = RegCreateKeyEx(HKEY_CURRENT_USER, path,0, NULL, REG_OPTION_NON_VOLATILE, KEY_ALL_ACCESS, NULL, &hKey, &dwDisp);
if (ret != ERROR_SUCCESS)
{
cout << "创建注册表失败" << endl;
return 1;
}
ret == RegSetValueEx(hKey, key, 0, dwType, (BYTE*)value, strlen(value));
if (ret != ERROR_SUCCESS)
{
cout << "注册表中创建KEY VALUE失败" << endl;
RegCloseKey(hKey);
return 1;
}
RegCloseKey(hKey);
return 0;
}
/************************************
@ Brief: 删除注册表
@ Author: woniu201
@ Created: 2018/09/07
@ Return:
************************************/
int DelReg(char* path)
{
int ret = RegDeleteKey(HKEY_CURRENT_USER, path);
if (ret == ERROR_SUCCESS)
{
cout << "删除成功" << endl;
}
else
{
cout << "删除失败" << endl;
return 1;
}
return 0;
}
int _tmain(int argc, _TCHAR* argv[])
{
char value[32] = {0};
ReadReg("Software\Woniu", "aaa", value);
WriteReg("Software\Woniu", "aaa", "bbb");
DelReg("Software\Woniu");
getchar();
return 0;
}
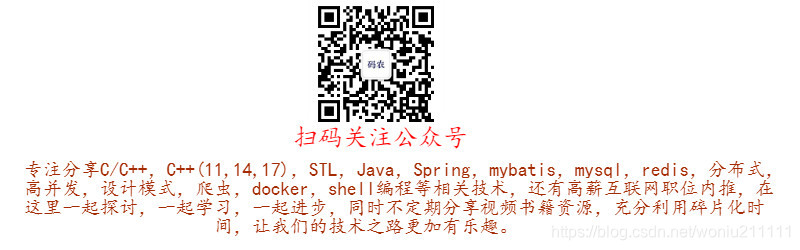