商品分页查询 ego-prc 实现
1.1 功能分析
使用 easyui 的 DataGrid 控件实现商品的分页查询,DataGrid 控件提交分页所需要的 page 和
rows 参数( page:第几页, rows:每页显示的记录数),
后台响应包含总记录数 total 和需要显示的商品对象的集合 rows 的 json 对象。
1.2PageHelper 说明
1.2.1PageHelper 资源地址
https://github.com/pagehelper/Mybatis-PageHelper
1.2.2PageHelper 实现原理
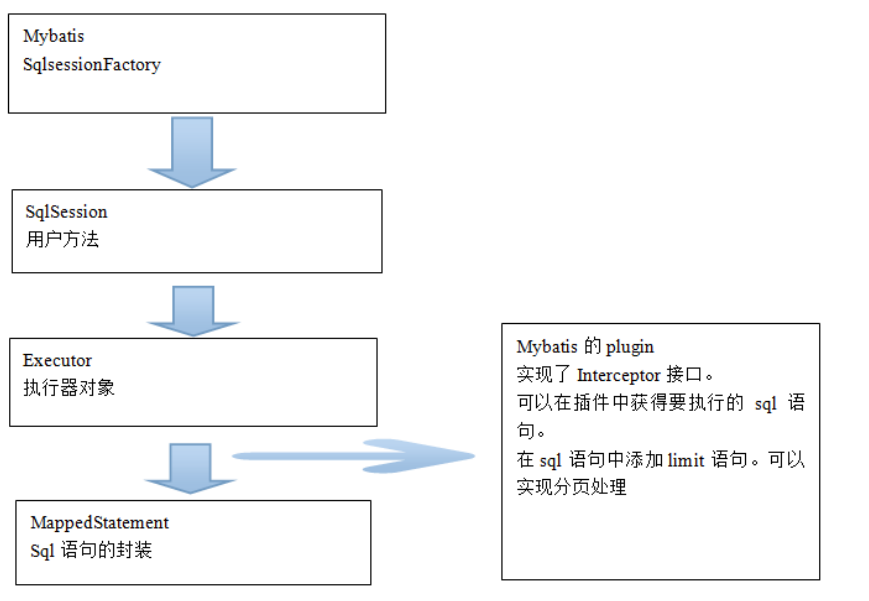
在查询的sql语句执行之前,添加一行代码PageHelper.startPage(1, 10);第一个参数表示第几页,第二个参数表示每页显示的记录数。这样在执行sql后就会将记录按照语句中设置的那样进行分页。如果需要获取总记录数的话,需要PageInfo类的对象,这个对象可以获取总记录数,下面看下测试的代码。
1.2.3PageHelper 配置
(在mybatis配置文件中配置)
<!-- 配置分页插件 --> <plugins> <plugin interceptor="com.github.pagehelper.PageHelper"> <!-- 设置数据库类型 Oracle,Mysql,MariaDB,SQLite,Hsqldb,PostgreSQL六 种数据库 --><property name="dialect" value="mysql" /> </plugin> </plugins>
1.3ego-common
创建 PageResult 类

package com.bjsxt.ego.beans; import java.io.Serializable; import java.util.List; /** * 封装datagrid控件需要的数据模型 * **/ public class PageResult<T> implements Serializable{ private List<T> rows; private Long total; public List<T> getRows() { return rows; } public void setRows(List<T> rows) { this.rows = rows; } public Long getTotal() { return total; } public void setTotal(Long total) { this.total = total; } }
1.4 创建 ItemService 接口

package com.bjsxt.ego.rpc.service; import com.bjsxt.ego.beans.PageResult; import com.bjsxt.ego.rpc.pojo.TbItem; public interface ItemService { /** * 实现商品信息的分页查询 * **/ public PageResult<TbItem> selectItemList(Integer page,Integer rows); }
1.5 创建 ItemServiceImpl 实现类
package com.bjsxt.ego.rpc.service.impl; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.bjsxt.ego.beans.PageResult; import com.bjsxt.ego.rpc.mapper.TbItemMapper; import com.bjsxt.ego.rpc.pojo.TbItem; import com.bjsxt.ego.rpc.pojo.TbItemExample; import com.bjsxt.ego.rpc.service.ItemService; import com.github.pagehelper.Page; import com.github.pagehelper.PageHelper; @Service public class ItemServiceImpl implements ItemService { //注入mapper接口代理对象 @Autowired private TbItemMapper tbItemMapper; @Override public PageResult<TbItem> selectItemList(Integer page, Integer rows) { // TODO Auto-generated method stub //执行分页操作 Page ps = PageHelper.startPage(page, rows); TbItemExample example=new TbItemExample(); //执行数据库查询操作 List<TbItem> list = tbItemMapper.selectByExample(example); PageResult<TbItem> result = new PageResult<TbItem>(); result.setRows(list); result.setTotal(ps.getTotal()); return result; } }
1.6 配置 applicationContext-dubbo.xml
<!-- 发布dubbo服务 --> <dubbo:service interface="com.bjsxt.ego.rpc.service.ItemService" ref="itemServiceImpl"></dubbo:service>
1.7 启动 ego-rpc-service-impl 发布 RPC 服务

package com.bjsxt.ego.test; import java.io.IOException; import org.springframework.context.support.ClassPathXmlApplicationContext; public class ProviderTest { public static void main(String[] args) { /** * 加载spring容器,完成服务发布 * **/ ClassPathXmlApplicationContext ac= new ClassPathXmlApplicationContext("spring/applicationContext-dao.xml", "spring/applicationContext-service.xml", "spring/applicationContext-tx.xml", "spring/applicationContext-dubbo.xml"); ac.start(); //阻塞程序的运行 try { System.in.read(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } ac.stop(); } }
商品分页查询 ego-manager-web 实现
2.1 配置 applicationContext-dubbo.xml
<!-- spring容器中存在一个远程服务的代理对象 --> <dubbo:reference interface="com.bjsxt.ego.rpc.service.ItemService" id="itemServiceProxy"></dubbo:reference>
2.2 创建 ManagerItemService 接口

package com.bjsxt.ego.manager.service; import com.bjsxt.ego.beans.PageResult; import com.bjsxt.ego.rpc.pojo.TbItem; public interface ManagerItemService { /** * 完成商品信息的分页查询 * **/ public PageResult<TbItem> selectItemListService(Integer page ,Integer rows); }
2.3 创建 ManagerItemServiceImpl 实现类

package com.bjsxt.ego.manager.service.impl; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.bjsxt.ego.beans.PageResult; import com.bjsxt.ego.manager.service.ManagerItemService; import com.bjsxt.ego.rpc.pojo.TbItem; import com.bjsxt.ego.rpc.service.ItemService; @Service public class ManagerItemServiceImpl implements ManagerItemService { //注入的是远程服务的代理对象 @Autowired private ItemService itemServiceProxy; @Override public PageResult<TbItem> selectItemListService(Integer page, Integer rows) { // TODO Auto-generated method stub return itemServiceProxy.selectItemList(page, rows); } }
2.4 创建 ItemController 类

package com.bjsxt.ego.manager.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.MediaType; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.ResponseBody; import com.bjsxt.ego.beans.PageResult; import com.bjsxt.ego.manager.service.ManagerItemService; import com.bjsxt.ego.rpc.pojo.TbItem; @Controller public class ItemController { //注入service对象 @Autowired private ManagerItemService managerItemService; /*** * 处理商品信息分页查询的请求 * **/ @RequestMapping(value="item/list",produces=MediaType.APPLICATION_JSON_VALU E+";charset=UTF-8") @ResponseBody public PageResult<TbItem> itemList(@RequestParam(defaultValue="1")Integer page, @RequestParam(defaultValue="30")Integer rows){ return managerItemService.selectItemListService(page, rows); } }
2.5 发布 ego-manager-web 访问商品列表
JsonResult类

package com.hainei.common.utils; /** * FileName: JsonResult * Author: Administrator * Date: 2019/6/24 20:20 * Description: 返回数据api */ public class JsonResult { // 响应业务状态 private Integer status; // 响应消息 private String msg; // 响应中的数据 private Object data; public static JsonResult build(Integer status, String msg, Object data) { return new JsonResult(status, msg, data); } public static JsonResult ok(Object data) { return new JsonResult(data); } public static JsonResult ok() { return new JsonResult(null); } public JsonResult() { } public static JsonResult build(Integer status, String msg) { return new JsonResult(status, msg, null); } public JsonResult(Integer status, String msg, Object data) { this.status = status; this.msg = msg; this.data = data; } public JsonResult(Object data) { this.status = 200; this.msg = "OK"; this.data = data; } public Integer getStatus() { return status; } public void setStatus(Integer status) { this.status = status; } public String getMsg() { return msg; } public void setMsg(String msg) { this.msg = msg; } public Object getData() { return data; } public void setData(Object data) { this.data = data; } }
PageHelper.startPage(query.getPageNum(), query.getPageSize()); List<EmergencyFireCar> emergencyFireCars = emergencyFireCarService.listAll(query); PageInfo<EmergencyFireCar> pageInfo = new PageInfo<>(emergencyFireCars);
关于pagehelper的详细使用博客