HTML
<!-- 自定义音乐播放器 -->
<view class="audio" wx:elif="{{articleType===3&&articleData.videoUrl}}">
<image class="bg" src="{{util.isUrl(articleData.coverPicture)?articleData.coverPicture:'/assets/bg_lv.png'}}"></image>
<image mode="aspectFill" class="poster" src="{{util.isUrl(articleData.coverPicture)?articleData.coverPicture:'/assets/bg_lv.png'}}"></image>
<view class="control-process">
<text class="current-process">{{current_process}}</text>
<slider class="slider" bindchange="hanle_slider_change" bindtouchstart="handle_slider_move_start" bindtouchend="handle_slider_move_end" min="0" block-size="16" max="{{slider_max}}" activeColor="#fff" backgroundColor="rgba(255,255,255,.3)" value="{{slider_value}}" />
<text class="total-process">{{total_process}}</text>
</view>
<view class="icon-list ">
<image bindtap="prev" mode="widthFix" src="/assets/icon_last.png" class="audio-icon"></image>
<image mode="widthFix" src="{{is_play?'/assets/lALPBFf_6KlotvJWVg_86_86.png':'/assets/icon_play.png'}}" class="audio-icon audio-play" bindtap="audio_play"></image>
<image bindtap="next" mode="widthFix" src="/assets/icon_next.png" class="audio-icon"></image>
</view>
</view>
CSS
.audio {
position: relative;
height: 600rpx;
padding: 60rpx 32rpx 52rpx;
box-sizing: border-box;
text-align: center;
overflow: hidden;
background: rgba(0,0,0,.18);
}
.audio .bg {
position: absolute;
top: 0;
left: -100%;
bottom: 0;
right: 0;
margin: auto;
width: 300%;
height: 300%;
z-index: -1;
filter: blur(40rpx);
}
.editor {
padding: 32rpx;
box-sizing: border-box;
color: #333;
font-size: 28rpx;
background: #fff;
}
.editor view {
max-width: 100% !important;
}
.audio .poster {
width: 250rpx;
height: 250rpx;
border-radius: 5px;
background: transparent;
}
/* 音频滚动条start */
.control-process {
margin: 20rpx 0;
display: flex;
justify-content: space-between;
align-items: center;
}
.control-process .slider {
width: 526rpx;
}
.control-process text {
font-size: 24rpx;
color: #fff;
}
/* 音频滚动条end */
.audio .icon-list {
position: relative;
margin: 0 auto;
line-height: 102rpx;
}
.audio .icon-list .audio-icon + .audio-icon {
margin-left: 72rpx;
}
.audio .icon-list .pattern {
position: absolute;
right: 20rpx;
}
.audio image {
width: 64rpx;
height: 64rpx;
vertical-align: middle;
}
.audio .audio-play {
width: 112rpx;
height: 112rpx;
}
.audio .pattern {
position: absolute;
top: 0;
bottom: 0;
margin: auto 0;
width: 44rpx;
height: 44rpx;
}
/* 音频end */
JS
// 设置注册音频
getaudio (item) {
let that = this
console.log(item)
// item.audioUrl为音频播放链接
let response = item.audioUrl
InnerAudioContext.src = response
InnerAudioContext.volume = 0
InnerAudioContext.autoplay = true
setTimeout(function() {
InnerAudioContext.volume = 1
InnerAudioContext.autoplay = false
InnerAudioContext.pause(() => {})
}, 200)
if (InnerAudioContext.duration == 0) {
InnerAudioContext.onCanplay(() => {
// 必须。可以当做是初始化时长
InnerAudioContext.duration;
// 必须。不然也获取不到时长
console.log(InnerAudioContext.duration)
setTimeout(() => {
that.setData({
current_process: format(InnerAudioContext.currentTime),
slider_value: Math.floor(InnerAudioContext.currentTime),
total_process: format(InnerAudioContext.duration),
slider_max: Math.floor(InnerAudioContext.duration)
})
setTimeout(() => {
if (that.data.total_process == 0) {
that.setData({
current_process: format(InnerAudioContext.currentTime),
slider_value: Math.floor(InnerAudioContext.currentTime),
total_process: format(InnerAudioContext.duration),
slider_max: Math.floor(InnerAudioContext.duration)
})
}
}, 1500)
}, 1000)
})
} else {
that.setData({
current_process: format(InnerAudioContext.currentTime),
slider_value: Math.floor(InnerAudioContext.currentTime),
total_process: format(InnerAudioContext.duration),
slider_max: Math.floor(InnerAudioContext.duration)
})
}
//监听音乐更新
InnerAudioContext.onTimeUpdate(() => {
if (!that.data.is_moving_slider) {
that.setData({
current_process: format(InnerAudioContext.currentTime),
slider_value: Math.floor(InnerAudioContext.currentTime),
total_process: format(InnerAudioContext.duration),
slider_max: Math.floor(InnerAudioContext.duration)
})
}
})
//监听音乐结束
InnerAudioContext.onEnded(() => {
that.setData({
is_play: false,
slider_value: 0,
current_process: '00:00',
is_ended: true
})
})
},
//销毁的时候置空音乐播放器
onUnload() {
let that = this
InnerAudioContext.stop()
InnerAudioContext.seek(0)
that.setData({
is_play: false,
slider_value: 0,
current_process: '00:00',
is_ended: true
})
},
// 拖动进度条,到指定位置
hanle_slider_change(e) {
const position = e.detail.value
this.seekCurrentAudio(position)
},
// 拖动进度条控件
seekCurrentAudio(tion) {
// 更新进度条
let that = this,
position = Math.floor(tion);
InnerAudioContext.seek(position)
that.setData({
current_process: format(position),
slider_value: Math.floor(position)
})
},
// 进度条滑动
handle_slider_move_start() {
this.setData({
is_moving_slider: true
});
},
handle_slider_move_end() {
this.setData({
is_moving_slider: false
});
},
// 点击播放暂停
audio_play: function() {
let that = this
console.log()
if (this.data.is_play) {
that.setData({
is_play: false
})
InnerAudioContext.pause(() => {
console.log('暂停播放')
})
} else if (!this.data.is_play) {
that.setData({
is_play: true
})
InnerAudioContext.play(() => {
console.log('播放')
})
}
},
// 上一首
prev: function() {
let that = this
if (that.data.audio_article.preArticleId != 0) {
wx.redirectTo({
url: '/pages/audio_article/audio_article?articleId=' +
that.data.audio_article.preArticleId
})
}
},
// 下一首
next: function() {
let that = this
if (that.data.audio_article.nextArticleId != 0) {
wx.redirectTo({
url: '/pages/audio_article/audio_article?articleId=' +
that.data.audio_article.nextArticleId
})
} else { // 如果是最后一首
that.setData({
is_play: false,
slider_value: 0,
current_process: '00:00',
is_ended: true
})
}
},
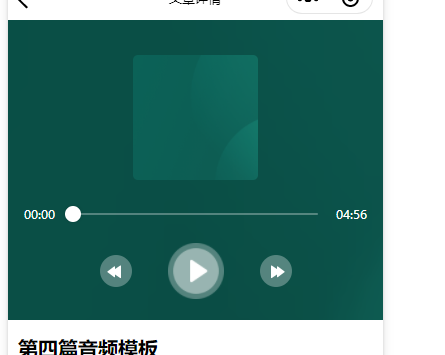