1: package cn.cqu.huang;
2:
3: class BiTree{
4: //二叉树节点包含三个域
5: private int data;
6: private BiTree left;
7: private BiTree right;
8:
9:
10: public BiTree(int x){
11: data = x;
12: }
13:
14: public void add(BiTree b){
15: if(b.data<data){
16: if(left==null){
17: left = b; //让b成为当前节点的左孩子
18: }else
19: left.add(b);
20:
21: }else{
22: if(right==null){
23: right = b; //让b成为当前节点的右孩子
24: }else
25: right.add(b);
26: }
27: }
28:
29: public void travel(){
30: //二叉排序树采用中序遍历,可以按顺序从小到大输出数字
31: if(left!=null) left.travel();
32: System.out.println(data);
33: if(right!=null) right.travel();
34: }
35: }
36:
37:
38: public class BiTreeTest {
39: public static void main(String[] args) {
40: BiTree bt = new BiTree(10);
41: bt.add(new BiTree(20));
42: bt.add(new BiTree(9));
43: bt.add(new BiTree(15));
44: bt.add(new BiTree(7));
45:
46: bt.travel();
47: }
48: }
49:
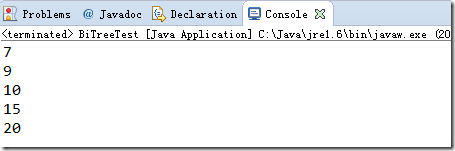