1、web.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
3 <display-name>springmvc1</display-name>
4
5 <filter>
6 <filter-name>characterEncoding</filter-name>
7 <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
8 <init-param>
9 <param-name>encoding</param-name>
10 <param-value>UTF-8</param-value>
11 </init-param>
12 </filter>
13 <filter-mapping>
14 <filter-name>characterEncoding</filter-name>
15 <url-pattern>/*</url-pattern>
16 </filter-mapping>
17
18 <servlet>
19 <servlet-name>springmvc</servlet-name>
20 <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
21 <init-param>
22 <param-name>contextConfigLocation</param-name>
23 <param-value>classpath:springmvc.xml</param-value>
24 </init-param>
25 </servlet>
26 <servlet-mapping>
27 <servlet-name>springmvc</servlet-name>
28 <url-pattern>*.do</url-pattern>
29 </servlet-mapping>
30 <!-- <servlet-mapping>
31 <servlet-name>springmvc</servlet-name>
32 <url-pattern>/rest/*</url-pattern>
33 </servlet-mapping> -->
34
35 <welcome-file-list>
36 <welcome-file>index.html</welcome-file>
37 <welcome-file>index.htm</welcome-file>
38 <welcome-file>index.jsp</welcome-file>
39 <welcome-file>default.html</welcome-file>
40 <welcome-file>default.htm</welcome-file>
41 <welcome-file>default.jsp</welcome-file>
42 </welcome-file-list>
43
44 </web-app>
2、springmvc.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <beans xmlns="http://www.springframework.org/schema/beans"
3 xmlns:mvc="http://www.springframework.org/schema/mvc"
4 xmlns:aop="http://www.springframework.org/schema/aop"
5 xmlns:tx="http://www.springframework.org/schema/tx"
6 xmlns:context="http://www.springframework.org/schema/context"
7 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
8 xsi:schemaLocation="http://www.springframework.org/schema/beans
9 http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
10 http://www.springframework.org/schema/mvc
11 http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
12 http://www.springframework.org/schema/context
13 http://www.springframework.org/schema/context/spring-context-3.2.xsd
14 http://www.springframework.org/schema/aop
15 http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
16 http://www.springframework.org/schema/tx
17 http://www.springframework.org/schema/tx/spring-tx-3.2.xsd">
18
19 <!-- 把Controller交给spring管理 -->
20 <context:component-scan base-package="com.xiaostudy"/>
21
22 <!-- <mvc:annotation-driven/> --><!-- 这个可以替代下面处理器映射器和处理器适配器,仅在注解情况下可用 -->
23
24 <!-- 配置注解处理器映射器 功能:寻找执行类Controller -->
25 <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"/>
26
27 <!-- 配置注解处理器适配器 功能:调用controller方法,执行controller -->
28 <bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter"/>
29
30 <!-- 配置sprigmvc视图解析器:解析逻辑试图
31 后台返回逻辑试图:index
32 视图解析器解析出真正物理视图:前缀+逻辑试图+后缀====/WEB-INF/index.jsp -->
33 <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
34 <property name="prefix" value="/WEB-INF/"/>
35 <property name="suffix" value=".jsp"/>
36 </bean>
37 </beans>
3、domain类
1 package com.xiaostudy.domain;
2
3 public class User {
4
5 private int id;
6 private String username;
7 private String password;
8 private int age;
9
10 public int getId() {
11 return id;
12 }
13
14 public void setId(int id) {
15 this.id = id;
16 }
17
18 public String getUsername() {
19 return username;
20 }
21
22 public void setUsername(String username) {
23 this.username = username;
24 }
25
26 public String getPassword() {
27 return password;
28 }
29
30 public void setPassword(String password) {
31 this.password = password;
32 }
33
34 public int getAge() {
35 return age;
36 }
37
38 public void setAge(int age) {
39 this.age = age;
40 }
41
42 @Override
43 public String toString() {
44 return "User [id=" + id + ", username=" + username + ", password=" + password + ", age=" + age + "]";
45 }
46
47 }
4、注解类
1 package com.xiaostudy.controller;
2
3 import org.springframework.stereotype.Controller;
4 import org.springframework.ui.Model;
5 import org.springframework.web.bind.annotation.PathVariable;
6 import org.springframework.web.bind.annotation.RequestMapping;
7 import org.springframework.web.bind.annotation.RequestMethod;
8 import org.springframework.web.bind.annotation.RequestParam;
9
10 import com.xiaostudy.domain.User;
11
12 @Controller//<bean class="com.xiaostudy.controller.MyController"/>
13 @RequestMapping(value="/myController")//访问该类的方法时,前面多这样一个路径
14 public class MyController {
15
16 // @RequestMapping("hello")//http://localhost:8080/demo2/hello.do
17 // @RequestMapping("/hello")//http://localhost:8080/demo2/hello.do
18 // @RequestMapping(value="/hello.do")//http://localhost:8080/demo2/hello.do
19 // @RequestMapping(value="/hello.do",method=RequestMethod.GET)//http://localhost:8080/demo2/hello.do
20 // @RequestMapping(value="/hello.do",method= {RequestMethod.GET,RequestMethod.POST})//http://localhost:8080/demo2/hello.do
21 public String print() {
22 return "index";
23 }
24
25 @RequestMapping("hi")//http://localhost:8080/demo2/myController/hi.do
26 public String hello() {
27 return "index";
28 }
29
30 @RequestMapping("requestint")//http://localhost:8080/demo2/myController/requestint.do
31 public String requestint(int id) {
32 System.out.println(id);
33 return "index";
34 }
35
36 @RequestMapping("requestint_2")//http://localhost:8080/demo2/myController/requestint2.do
37 public String requestint_2(@RequestParam(value="id2",required=true)int id) {//value="id2"表示:更改参数别名,required=true表示:直接访问地址会报错,必须要转跳
38 System.out.println(id);
39 return "index";
40 }
41
42 @RequestMapping("requestint2")//http://localhost:8080/demo2/myController/requestint2.do
43 public String requestint2(int id, int i) {
44 System.out.println(id + " " + i);
45 return "index";
46 }
47
48 @RequestMapping("requestint3")//http://localhost:8080/demo2/myController/requestint3.do
49 public String requestint3(User user) {
50 System.out.println(user);
51 return "index";
52 }
53
54 @RequestMapping("requestint4")//http://localhost:8080/demo2/myController/requestint4.do
55 public String requestint4(CustomUser customUser) {
56 System.out.println(customUser);
57 return "index";
58 }
59
60 @RequestMapping("xiaostudy")//http://localhost:8080/demo2/myController/xiaostudy.do
61 public String add() {
62 return "xiaostudy";
63 }
64
65 @RequestMapping("ok")//http://localhost:8080/demo2/myController/ok.do
66 public String ok(Model model) {//Model作用:用于回显数据
67 User user = new User();
68 user.setId(2);
69 user.setUsername("xiaostudy");
70 user.setPassword("123456");
71 user.setAge(23);
72 model.addAttribute("user", user);
73 return "ok";
74 }
75
76 @RequestMapping("id/{id}")//http://localhost:8080/demo2/myController/id/?.do
77 public String id(@PathVariable int id) {//@PathVariable:说明{id}是对参数的id
78 System.out.println(id);
79 return "index";
80 }
81
82 @RequestMapping("test")//http://localhost:8080/demo2/myController/test.do
83 public String test() {
84 return "forward:index";//转发
85 }
86
87 @RequestMapping("test2")//http://localhost:8080/demo2/myController/test2.do
88 public String test2() {
89 return "redirect:index";//重定向
90 }
91
92 }
5、回显数据jsp
1 <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
2 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
3 "http://www.w3.org/TR/html4/loose.dtd">
4 <html>
5 <head>
6 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
7 <title>springMVC_demo</title>
8 </head>
9 <body>
10 ${user.id }||${user.username }||${user.password }||${user.age }<br/>
11 <a href="${pageContext.request.contextPath }/myController/id/${user.id}.do">id</a><br/>
12 <%-- <a href="${pageContext.request.contextPath }/rest/myController/id/${user.id}">id2</a><br/> --%>
13 </body>
14 </html>
项目文件结构
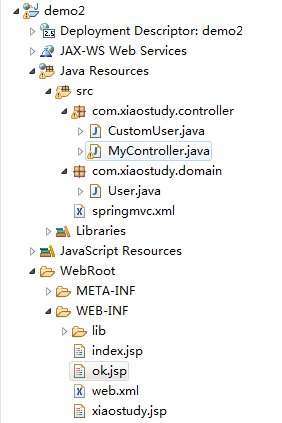