A. The Useless Toy
Walking through the streets of Marshmallow City, Slastyona have spotted some merchants selling a kind of useless toy which is very popular nowadays – caramel spinner! Wanting to join the craze, she has immediately bought the strange contraption.
Spinners in Sweetland have the form of V-shaped pieces of caramel. Each spinner can, well, spin around an invisible magic axis. At a specific point in time, a spinner can take 4 positions shown below (each one rotated 90 degrees relative to the previous, with the fourth one followed by the first one):

After the spinner was spun, it starts its rotation, which is described by a following algorithm: the spinner maintains its position for a second then majestically switches to the next position in clockwise or counter-clockwise order, depending on the direction the spinner was spun in.
Slastyona managed to have spinner rotating for exactly n seconds. Being fascinated by elegance of the process, she completely forgot the direction the spinner was spun in! Lucky for her, she managed to recall the starting position, and wants to deduct the direction given the information she knows. Help her do this.
There are two characters in the first string – the starting and the ending position of a spinner. The position is encoded with one of the following characters: v (ASCII code 118, lowercase v), < (ASCII code 60), ^ (ASCII code 94) or > (ASCII code 62) (see the picture above for reference). Characters are separated by a single space.
In the second strings, a single number n is given (0 ≤ n ≤ 109) – the duration of the rotation.
It is guaranteed that the ending position of a spinner is a result of a n second spin in any of the directions, assuming the given starting position.
Output cw, if the direction is clockwise, ccw – if counter-clockwise, and undefined otherwise.
^ >
1
cw
< ^
3
ccw
^ v
6
undefined
不管n多大,余4就行。然后判断下。
1 #include <bits/stdc++.h> 2 using namespace std; 3 4 int main() { 5 char s1,s2; 6 int n; 7 cin>>s1>>s2>>n; 8 n%=4; 9 if(n==0 || n == 2) printf("undefined "); 10 else if(n==1){ 11 if(s1==94&&s2==62||s1==62&&s2==118||s1==118&&s2==60||s1==60&&s2==94)printf("cw "); 12 else if(s1==94&&s2==60||s1==60&&s2==118||s1==118&&s2==62||s1==62&&s2==94)printf("ccw "); 13 else printf("undefined "); 14 }else if(n == 3){ 15 if(s1==94&&s2==60||s1==62&&s2==94||s1==118&&s2==62||s1==60&&s2==118)printf("cw "); 16 else if(s1==94&&s2==62||s1==62&&s2==118||s1==118&&s2==60||s1==60&&s2==94)printf("ccw "); 17 else printf("undefined "); 18 } 19 return 0; 20 }
B. The Festive Evening
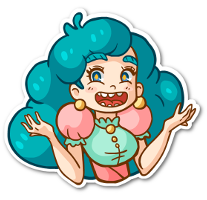
It's the end of July – the time when a festive evening is held at Jelly Castle! Guests from all over the kingdom gather here to discuss new trends in the world of confectionery. Yet some of the things discussed here are not supposed to be disclosed to the general public: the information can cause discord in the kingdom of Sweetland in case it turns out to reach the wrong hands. So it's a necessity to not let any uninvited guests in.
There are 26 entrances in Jelly Castle, enumerated with uppercase English letters from A to Z. Because of security measures, each guest is known to be assigned an entrance he should enter the castle through. The door of each entrance is opened right before the first guest's arrival and closed right after the arrival of the last guest that should enter the castle through this entrance. No two guests can enter the castle simultaneously.
For an entrance to be protected from possible intrusion, a candy guard should be assigned to it. There are k such guards in the castle, so if there are more than k opened doors, one of them is going to be left unguarded! Notice that a guard can't leave his post until the door he is assigned to is closed.
Slastyona had a suspicion that there could be uninvited guests at the evening. She knows the order in which the invited guests entered the castle, and wants you to help her check whether there was a moment when more than k doors were opened.
Two integers are given in the first string: the number of guests n and the number of guards k (1 ≤ n ≤ 106, 1 ≤ k ≤ 26).
In the second string, n uppercase English letters s1s2... sn are given, where si is the entrance used by the i-th guest.
Output «YES» if at least one door was unguarded during some time, and «NO» otherwise.
You can output each letter in arbitrary case (upper or lower).
5 1
AABBB
NO
5 1
ABABB
YES
In the first sample case, the door A is opened right before the first guest's arrival and closed when the second guest enters the castle. The door B is opened right before the arrival of the third guest, and closed after the fifth one arrives. One guard can handle both doors, as the first one is closed before the second one is opened.
In the second sample case, the door B is opened before the second guest's arrival, but the only guard can't leave the door A unattended, as there is still one more guest that should enter the castle through this door.
直接模拟。
1 #include <bits/stdc++.h> 2 using namespace std; 3 const int MAX = 1e6+10; 4 char str[MAX]; 5 bool vis[30]; 6 int main() { 7 int n, k; 8 cin>>n>>k; 9 scanf("%s",str); 10 set<int> st; 11 for(int i = n-1; i >= 0; i --){ 12 if(!vis[str[i]-'A']){ 13 st.insert(i); 14 vis[str[i]-'A'] = true; 15 } 16 } 17 int ans = 0,maxn = -1; 18 memset(vis,false,sizeof(vis)); 19 for(int i = 0; i < n; i ++) { 20 if(!vis[str[i]-'A']){ 21 ans++; 22 if(ans > maxn)maxn = ans; 23 if(!st.count(i)) 24 vis[str[i]-'A'] = true; 25 else ans--; 26 }else { 27 if(st.count(i)){ 28 ans--; 29 } 30 } 31 } 32 printf("%s ",maxn>k?"YES":"NO"); 33 return 0; 34 }
C. The Meaningless Game
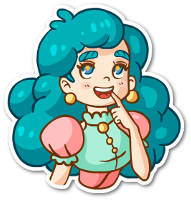
Slastyona and her loyal dog Pushok are playing a meaningless game that is indeed very interesting.
The game consists of multiple rounds. Its rules are very simple: in each round, a natural number k is chosen. Then, the one who says (or barks) it faster than the other wins the round. After that, the winner's score is multiplied by k2, and the loser's score is multiplied by k. In the beginning of the game, both Slastyona and Pushok have scores equal to one.
Unfortunately, Slastyona had lost her notepad where the history of all n games was recorded. She managed to recall the final results for each games, though, but all of her memories of them are vague. Help Slastyona verify their correctness, or, to put it another way, for each given pair of scores determine whether it was possible for a game to finish with such result or not.
In the first string, the number of games n (1 ≤ n ≤ 350000) is given.
Each game is represented by a pair of scores a, b (1 ≤ a, b ≤ 109) – the results of Slastyona and Pushok, correspondingly.
For each pair of scores, answer "Yes" if it's possible for a game to finish with given score, and "No" otherwise.
You can output each letter in arbitrary case (upper or lower).
6
2 4
75 45
8 8
16 16
247 994
1000000000 1000000
Yes
Yes
Yes
No
No
Yes
First game might have been consisted of one round, in which the number 2 would have been chosen and Pushok would have won.
The second game needs exactly two rounds to finish with such result: in the first one, Slastyona would have said the number 5, and in the second one, Pushok would have barked the number 3.
数学太渣了,看了别人的代码才直接可以用立法根。
1 #include <bits/stdc++.h> 2 #define ll long long 3 using namespace std; 4 5 int main() { 6 int n; 7 ll a,b; 8 scanf("%d",&n); 9 while(n--) { 10 scanf("%lld%lld",&a,&b); 11 ll x = cbrt(a*b+0.5); 12 ll xx = a/x, yy = b/x; 13 if(xx*yy*xx == a && xx*yy*yy == b)puts("Yes"); 14 else puts("No"); 15 } 16 return 0; 17 }