{
"name": "张三",
"age": 33,
"sex": true,
"weight": 123.456,
"tel": ["86-1111111", "86-2222222"],
"addresses":{"address":"A省B市",
"pc":"100001"},
"children": [
{
"name": "张继",
"age": "22",
"sex": true
},
{
"name": "张承",
"age": "11",
"sex": false
}
]
}
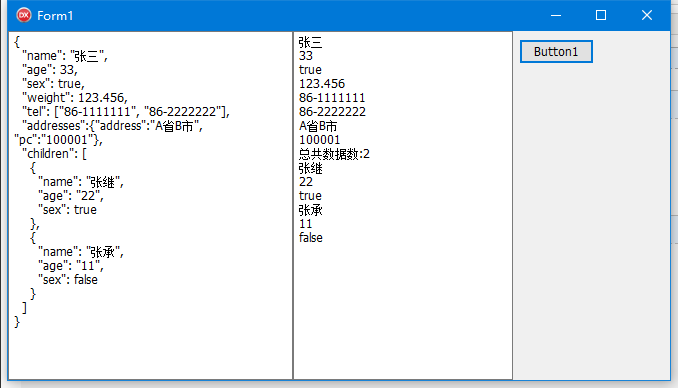
uses JsonDataObjects;
procedure TForm1.Button1Click(Sender: TObject);
var
jo: TJsonObject;
i: Integer;
begin
jo := TJsonObject.Parse( Memo1.Text) as TJsonObject;
Memo2.Lines.Add(jo['name']);
Memo2.Lines.Add(jo['age']);
Memo2.Lines.Add(jo['sex']);
Memo2.Lines.Add(jo['weight']);
Memo2.Lines.Add(jo['tel'].Items[0]);
Memo2.Lines.Add(jo['tel'].Items[1]);
Memo2.Lines.Add(jo['addresses'].S['address']);
Memo2.Lines.Add(jo['addresses'].S['pc']);
Memo2.Lines.Add('总共数据数:' + inttostr(jo['children'].Count));
for i := 0 to jo['children'].Count - 1 do
begin
Memo2.Lines.Add(jo['children'].Items[i]['name']);
Memo2.Lines.Add(jo['children'].Items[i]['age']);
Memo2.Lines.Add(jo['children'].Items[i]['sex']);
end;
jo.Free;
-----------------------------------------