Crawling in process... Crawling failed Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu
Description
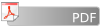
Suppose you have to evaluate an expression like A*B*C*D*E where A,B,C,D and E are matrices. Since matrix multiplication is associative, the order in which multiplications are performed is arbitrary. However, the number of elementary multiplications needed strongly depends on the evaluation order you choose.
For example, let A be a 50*10 matrix, B a 10*20 matrix and C a 20*5 matrix. There are two different strategies to compute A*B*C, namely (A*B)*C and A*(B*C).
The first one takes 15000 elementary multiplications, but the second one only 3500.
Your job is to write a program that determines the number of elementary multiplications needed for a given evaluation strategy.
Input Specification
Input consists of two parts: a list of matrices and a list of expressions.
The first line of the input file contains one integer n ( ), representing the number of matrices in the first part. The next n lines each contain one capital letter, specifying the name of the matrix, and two integers, specifying the number of rows and columns of the matrix.
The second part of the input file strictly adheres to the following syntax (given in EBNF):
SecondPart = Line { Line } <EOF> Line = Expression <CR> Expression = Matrix | "(" Expression Expression ")" Matrix = "A" | "B" | "C" | ... | "X" | "Y" | "Z"
Output Specification
For each expression found in the second part of the input file, print one line containing the word "error" if evaluation of the expression leads to an error due to non-matching matrices. Otherwise print one line containing the number of elementary multiplications needed to evaluate the expression in the way specified by the parentheses.
Sample Input
9 A 50 10 B 10 20 C 20 5 D 30 35 E 35 15 F 15 5 G 5 10 H 10 20 I 20 25 A B C (AA) (AB) (AC) (A(BC)) ((AB)C) (((((DE)F)G)H)I) (D(E(F(G(HI))))) ((D(EF))((GH)I))
Sample Output
0 0 0 error 10000 error 3500 15000 40500 47500 15125
题目大意:给你若干个矩阵(x*y),然后给你若干种计算公式,问你在该种计算公式情况下能否进行矩阵乘法运算,
若能进行,输出需进行乘法的次数。
思路分析:首先要对矩阵的乘法运算有一定了解,首先,A(x*y)和B(x*y)矩阵能否进行A*B运算的充要条件是是否满足
A.y==B.x,如果满足,则会得到矩阵C(A.x*B.y),这次运算进行的乘法的次数是A,x*A.y*B.y.下面就考虑如何进行
实现,首先括号里面是优先计算的,也就是说我们刚开始要计算的是最内层的括号里面的表达式,也就是在碰到第一个“)”
进行运算的表达式,每碰到一个”)“,就要进行一次矩阵运算,因此可以考虑用栈这种数据结构来实现,碰到”)“就进行
矩阵运算,把运算得到的矩阵再压入栈中,对于每一个矩阵,需要维护的信息是它的x和y,矩阵的类型可以用数组下标来区分。
代码:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <map>
#include <string>
#include <queue>
#include <stack>
using namespace std;
const int maxn=30;
struct nod
{
int x;
int y;
};
nod mat[maxn];
stack<nod> sta;
int main()
{
int n,i;
cin>>n;
char s;
int a,b;
char q[100];
memset(mat,0,sizeof(mat));
while(n--)
{
cin>>s>>a>>b;
int i=s-'A';
mat[i].x=a;
mat[i].y=b;
}
int sum;
while(scanf("%s",q)!=EOF)
{
sum=0;
int l=strlen(q);
for( i=0;i<l;i++)
{
if(q[i]=='(') continue;
if(q[i]>='A'&&q[i]<='Z')
{
int k=q[i]-'A';
sta.push(mat[k]);
}
if(q[i]==')')
{
nod m,k,w;
m=sta.top();
sta.pop();
k=sta.top();
sta.pop();
if(k.y!=m.x)
break;
w.x=k.x,w.y=m.y;
sta.push(w);
sum+=k.x*k.y*m.y;
}
}
if(i==l) cout<<sum<<endl;
else cout<<"error"<<endl;
}
return 0;
}