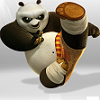
在游戏应用程序编码开始之前,首先问自己几个关于游戏设计问题,想清楚确定好目标后再着手开始设计开发。
- 它使什么类型的游戏?
- 游戏的目标是什么?
- 游戏的玩法是设计?
- 游戏的采用何种驱动?
- 游戏的艺术资源如何设计?
《礼记·中庸》:"凡事豫则立,不豫则废。言前定,则不跲;事前定,则不困;行前定,则不疚;道前定,则不穷。"任何事情,有准备就能成功,没有任何准备就会失败。话在事先准备好了,辩论时就不会理屈词穷了;在办事前先做好充分的准备,到时候就不至于处于困境了;在行动前先做好了充分的准备,到时候就不会感到内疚了;履行做人的原则,有准备了就不至于有什么不顺畅之事了。游戏的设计同样如此,如果游戏开发的中途修改游戏的设计初衷或者理念,将会付出昂贵的代价。孙子曰:"夫未战而庙算胜者,得算多也;未战而庙算不胜者,得算少也。多算胜,少算不胜,而况于无算乎!"讲述的也是这个道理。
游戏设计流程图
创建游戏设计文档有助于减轻潜在的缺陷,帮助开发团队中的成员理解和处理的游戏的设计逻辑。理解我们要设计的游戏逻辑,就从下面的流程图中开始。
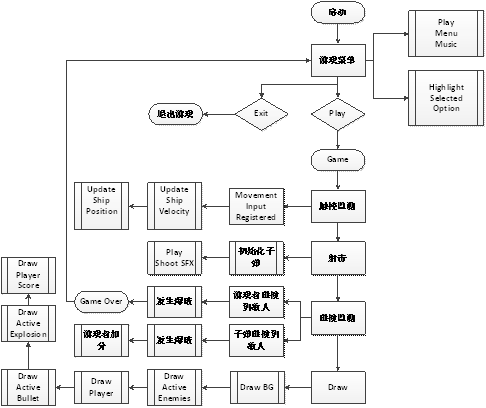
您可以看出,小小的射击游戏的流程图就如此繁杂,大型游戏的设计则更为复杂。详细的文档作为沟通工具可以帮助设计人员、开发人员和测试人员理解期望的目标,完成预期的工作。
游戏的视差背景
飞艇在移动时绘制云图案作为背景,并将其从右向左移动。本例中我们采用视差背景实现真实动感的背景效果。
天文学中采用视差法是确定天体之间距离。视差就是从有一定距离的两个点上观察同一个目标所产生的方向差异。从目标看两个点之间的夹角,叫做这两个点的视差角,两点之间的距离称作基线。只要知道视差角度和基线长度,就可以计算出目标和观测者之间的距离。
游戏开发中利用视觉上的误差,即通常所说的视错觉。造成所谓视差的不仅是人们肉眼所存在的局限和障碍,同时也是由于文化深层的联想和思考所引发。本例中绘制视差背景的方法是:用于绘制多层图像并以不同的速度移动,来达到在飞艇在云端飞行的视错觉。
按 SHIFT + ALT + C创建视差背景类,键入类名ParallaxingBackground.cs。
创建游戏角色类,类名为ParallaxingBackground。同时按下SHIFT + ALT + C,在弹出的窗体中选择[Code]|[Code file]。
在新创建的ParallaxingBackground.cs文件中添加引用。
XNA Project: Shooter File: ParallaxingBackground.cs
using System;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.Graphics;
在ParallaxingBackground类中定义类型为Texture2D的背景图片变量、Vector2数组和移动速度的变量。
XNA Project: Shooter File: ParallaxingBackground.cs
// The image representing the parallaxing background
Texture2D texture;
// An array of positions of the parallaxing background
Vector2[] positions;
// The speed which the background is moving
int speed;
在Initialize()方法中,加载背景图片,设定图片移动速度。
首先使用content.load方法初始化图形,然后计算Vector2数组中对象的数量(screenWidth / texture.Width + 1),其中+1的目的是为保证背景切换平滑。在For循环中,设定背景图片显示的初始位置。
XNA Project: Shooter File: ParallaxingBackground.cs
publicvoid Initialize(ContentManager content, String texturePath, int screenWidth, int speed)
{
// Load the background texture we will be using
texture = content.Load<Texture2D>(texturePath);
// Set the speed of the background
this.speed = speed;
// If we divide the screen with the texture width then we can determine the number of tiles need.
// We add 1 to it so that we won't have a gap in the tiling
positions = newVector2[screenWidth / texture.Width + 1];
// Set the initial positions of the parallaxing background
for (int i = 0; i < positions.Length; i++)
{
// We need the tiles to be side by side to create a tiling effect
positions[i] = newVector2(i * texture.Width, 0);
}
}
在Update方法中改变背景图片的位置坐标。每个背景图片被认为是一个瓷片Tile,更新瓷片的X轴坐标,以移动速度变量值作为X轴坐标移动的增量。如果移动速度变量小于零,则瓷片从右往左移动。如果移动速度变量大于零,则瓷片从左往右移动。移动时判断瓷片显示位置是否超出屏幕区域,如果是则重置瓷片的X轴坐标,以便背景滚动平滑。
XNA Project: Shooter File: ParallaxingBackground.cs
publicvoid Update()
{
// Update the positions of the background
for (int i = 0; i < positions.Length; i++)
{
// Update the position of the screen by adding the speed
positions[i].X += speed;
// If the speed has the background moving to the left
if (speed <= 0)
{
// Check the texture is out of view then put that texture at the end of the screen
if (positions[i].X <= -texture.Width)
{
positions[i].X = texture.Width * (positions.Length - 1);
}
}
// If the speed has the background moving to the right
else
{
// Check if the texture is out of view then position it to the start of the screen
if (positions[i].X >= texture.Width * (positions.Length - 1))
{
positions[i].X = -texture.Width;
}
}
}
}
位置坐标更新完毕后,使用Draw方法绘制视差背景。
XNA Project: Shooter File: ParallaxingBackground.cs
publicvoid Draw(SpriteBatch spriteBatch)
{
for (int i = 0; i < positions.Length; i++)
{
spriteBatch.Draw(texture, positions[i], Color.White);
}
}
在Game1.cs中声明视差背景的层bgLayer1和bgLayer2。
XNA Project: Shooter File: Game1.cs
// Image used to display the static background Texture2D mainBackground; // Parallaxing Layers ParallaxingBackground bgLayer1; ParallaxingBackground bgLayer2;
在Game1的Initialize方法中实例化视差背景层。
XNA Project: Shooter File: Game1.cs
bgLayer1 = new ParallaxingBackground();
bgLayer2 = new ParallaxingBackground();
在Game1的LoadContent方法中加载背景层。
XNA Project: Shooter File: Game1.cs
// Load the parallaxing background
bgLayer1.Initialize(Content, "bgLayer1", GraphicsDevice.Viewport.Width, -1);
bgLayer2.Initialize(Content, "bgLayer2", GraphicsDevice.Viewport.Width, -2);
mainBackground = Content.Load<Texture2D>("mainbackground");
在Game1的Update方法中,更新游戏角色飞艇之后更新视差背景。
XNA Project: Shooter File: Game1.cs
// Update the parallaxing background
bgLayer1.Update();
bgLayer2.Update();
在Game1的Draw方法中,绘制游戏背景。
XNA Project: Shooter File: Game1.cs
GraphicsDevice.Clear(Color.CornflowerBlue);
// Start drawing
spriteBatch.Begin();
spriteBatch.Draw(mainBackground, Vector2.Zero, Color.White);
// Draw the moving background
bgLayer1.Draw(spriteBatch);
bgLayer2.Draw(spriteBatch);
GraphicsDevice.Clear(Color.CornflowerBlue);
// Start drawing
spriteBatch.Begin();
spriteBatch.Draw(mainBackground, Vector2.Zero, Color.White);
// Draw the moving background
bgLayer1.Draw(spriteBatch);
bgLayer2.Draw(spriteBatch);
在模拟器中运行
按F5运行应用程序,或者点击Start Debugging按钮运行,如图 Start Debugging。

图 Start Debugging
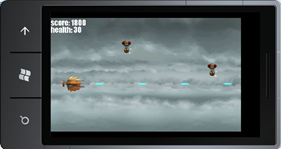
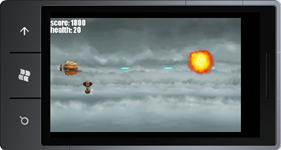
图 飞艇游戏
参考文献
本章参考和引用APP HUB(http://create.msdn.com/en-US)的Game Development Tutorial,以及MSDN Windows Phone开发文档。
作者:雪松
本文版权归作者所有,转载请注明出处。