计算所有客人带来的食物总数
代码如下:
#-*-coding:utf-8-*- #统计所有人带的食物总数 allguests = {'Alice':{'apples':5,'pretzels':12}, 'Bob':{'ham sandwiches':3,'apples':3}, 'Carol':{'cups':3,'apple pies':1} } def totalBrought(guests,items): numbrought = 0 for k,v in guests.items(): numbrought = numbrought + v.get(items,0) return numbrought print('number of things being brought:') print(' - Apples '+ str(totalBrought(allguests,'apples'))) print(' - Pretzels '+ str(totalBrought(allguests,'pretzels'))) print(' - Ham Sandwiches '+ str(totalBrought(allguests,'ham sandwiches'))) print(' - Cups '+ str(totalBrought(allguests,'cups'))) print(' - Apple Pies '+ str(totalBrought(allguests,'apple pies')))
好玩游戏的物品清单
你在创建一个好玩的视频游戏。用于对玩家物品清单建模的数据结构是一个字典。其中键是字符串,描述清单中的物品,值是一个整型值,说明玩家有多少该物品。例如,字典值{‘rope':1,'torch':6,'gold coin':42,'dagger':1,'arrow':12}意味着玩家有1条绳索,6坏人火把,42枚金币等。
写一个名为displayInventory()函数,它接受任何可能的物品清单,并显示如下:
Inventory: 12 arrow 42 gold coin 1 rope 6 torch 1 dagger Total number of items: 62
代码如下:
def displayInventory(inventory): print("Inventory:") item_total=0 for k,v in inventory.items(): print(str(v)+' '+ k) item_total += v print("Total number of items :" ,item_total) stuff={'rope':1,'torch':6,'gold coin':42,'dagger':1,'arrow':12} displayInventory(stuff)
列表到字典的函数,针对好玩游戏物品清单
假设征服一条龙的战利品表示为这样的字符串列表:
dragonLoot=['gold coin','dagger','gold coin','gold coin','ruby']
写一个名为addToInventory(inventory,addedItems)的函数,其中inventory参数是一个字典,表示玩家的物品清单(像前面项目一样),addedItems参数是一个列表,就像dragonLoot。
addToInventory()函数应该返回一个字典,表示更新过的物品清单.
所有代码合起来代码如下:
#游戏的物品清单,一个函数只能有一个返回值 stuff = {'rope':1 ,'torch':6 ,'gold coin':42 ,'dagger':1,'arrow':12} dragonLoot=['gold coin','dagger','gold coin','ruby','gold coin'] def displayInventory(inverntory): print('Inventory:') item_total = 0 for k,v in inverntory.items(): print(str(v) + ' ' + k) item_total = item_total + v print('Total number of items:' +str(item_total)) def addToInventory(inventory1,addedItems): newupdate = {} for i in addedItems: newupdate[i] = addedItems.count(i) for k,v in newupdate.items(): if k in inventory1.keys(): inventory1[k] = inventory1[k] + v else: inventory1[k] = v return inventory1 stuff = addToInventory(stuff,dragonLoot) displayInventory(stuff)
结果输出为:
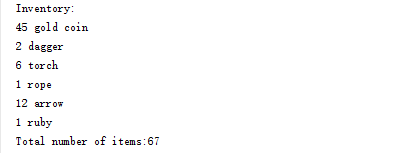
在addToInventory函数中运用了两个基本的思维:将第二个参数列表中重复的元素进行统计,赋给字典中,成为一个新字典。
然后将新字典与第一个参数字典进行相同Key合并成一个字典。