使用配置maven插件的方式配置逆向工程
一、新建maven项目
本次测试:新建一个空的maven项目,并且指定打包方式为war
二、pom文件配置
- 导入相关依赖:mybatis、mybatis逆向工程、mysql、lombok
- 配置逆向工程插件
这里为什么需要Lombok?
因为mybaitis逆向工程只能为pojo类生成setter/getter,不会生成toString等其他方法,所以用lombok为我们生成其他方法
<dependencies>
<!--mybatis核心-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.5</version>
</dependency>
<!-- mybatis逆向工程依赖 -->
<dependency>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-core</artifactId>
<version>1.3.6</version>
</dependency>
<!--mysql相关-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.6</version>
</dependency>
<!--lombok-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.2</version>
</dependency>
</dependencies>
<build>
<!--逆向工程插件-->
<plugins>
<plugin>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-maven-plugin</artifactId>
<version>1.3.6</version>
<configuration>
<configurationFile>
${basedir}/src/main/resources/generatorConfig.xml(其实是绝对位置)
</configurationFile>
<overwrite>true</overwrite>
<verbose>true</verbose>
</configuration>
<dependencies>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.6</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.4.5</version>
</dependency>
</dependencies>
</plugin>
</plugins>
</build>
配置完mybatis逆向工程插件,图示
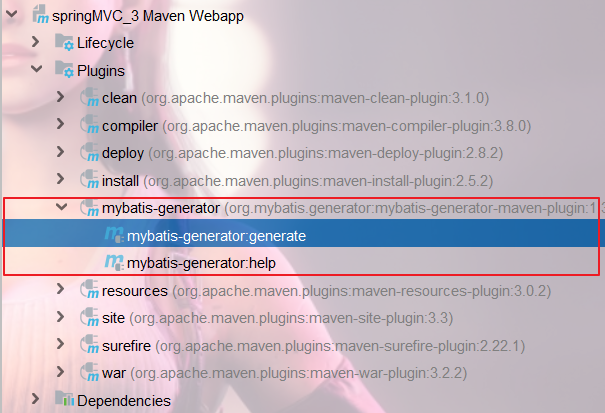
三、逆向工程xml文件
注意点:
- 如果项目中没有导入数据库的依赖包,那么在逆向工程xml文件中需要指定该驱动包的位置
<classPathEntry location=""/>
- 逆向工程生成pojo类的时候默认会生成相应的example类,可以关闭
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE generatorConfiguration
PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN"
"http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd">
<generatorConfiguration>
<!--导入properties文件属性-->
<properties resource="config/dbconfig.properties" />
<!--指定数据库mysql-connector-java-5.1.6.jar包位置-->
<!--<classPathEntry location=""/>-->
<!--id:自定义-->
<context id="mybatisConfig" targetRuntime="MyBatis3">
<!--禁用注释-->
<commentGenerator>
<property name="suppressAllComments" value="true"/>
<property name="suppressDate" value="true"/>
</commentGenerator>
<!--连接数据库,useSSL=false解决jdbc与mysql版本兼容问题-->
<jdbcConnection driverClass="${driverClass}" connectionURL="${connectionURL}"
userId="${userId}" password="${password}"/>
<!--生成entity类-->
<javaModelGenerator targetPackage="mybatis.pojo" targetProject="src/main/java">
<!-- enableSubPackages:是否让schema作为包的后缀 -->
<property name="enableSubPackages" value="false" />
<!-- 从数据库返回的值被清理前后的空格 -->
<property name="trimStrings" value="true" />
</javaModelGenerator>
<!--xml映射文件-->
<sqlMapGenerator targetPackage="mapper" targetProject="src/main/resources">
<!-- enableSubPackages:是否让schema作为包的后缀 -->
<property name="enableSubPackages" value="false" />
</sqlMapGenerator>
<!--mapper接口-->
<javaClientGenerator type="XMLMAPPER" targetPackage="mybatis.dao" targetProject="src/main/java">
<!-- enableSubPackages:是否让schema作为包的后缀 -->
<property name="enableSubPackages" value="false" />
</javaClientGenerator>
<!--
配置需要逆向工程生成实体类的对应的数据库表
schema:配置访问的数据库名
tableName:配置逆向工程生成的数据库表
domainObject:配置逆向工程生成实体类的名称
-->
<!--table配置,指定数据库中t_user表生成对应的User实体类-->
<!--
<table tableName="crm_admin" domainObjectName="Admin"
enableCountByExample="false"
enableUpdateByExample="false" enableDeleteByExample="false"
enableSelectByExample="false" selectByExampleQueryId="false"
/>
<table tableName="book" domainObjectName="Book"
enableCountByExample="false"
enableUpdateByExample="false" enableDeleteByExample="false"
enableSelectByExample="false" selectByExampleQueryId="false"
/>
-->
<!--全部表参与逆向工程-->
<!--以下example为false,表示不会生成example类,否则将自动生成example类-->
<table schema="" tableName="%"
enableCountByExample="false"
enableUpdateByExample="false"
enableDeleteByExample="false"
enableSelectByExample="false"
selectByExampleQueryId="false">
</table>
</context>
</generatorConfiguration>
四、dbconfig.properties文件
创建在src/main/resources/config/dbconfig.properties(位置不固定)
注意:不知道为什么逆向工程xml文件只读取到数据库连接信息,其他的2、3、4、5、6的配置信息读取不到
# 1.数据库连接信息
driverClass=com.mysql.jdbc.Driver
connectionURL=jdbc:mysql:///test?serverTimezone=UTC&useSSL=false
userId=root
password=root
# 2.生成entity类
targetPackageEntity="mybatis.pojo"
# 3.MapperXml映射文件
targetPackageMapperXml="mapper"
# 4.mapper接口
targetPackageDAO="mybatis.dao"
# 5.生成2、4文件的目标根目录位置
targetJavaProject="src/main/java"
# 6.生成3文件的目标根目录位置
targetResourcesProject=src/main/resources