引言
好久没写博客了,因为最近在准备考研,所以博客一直都没有写。之前完成的任务也没有写,今天正好完成一个工作,写写博客。
展示
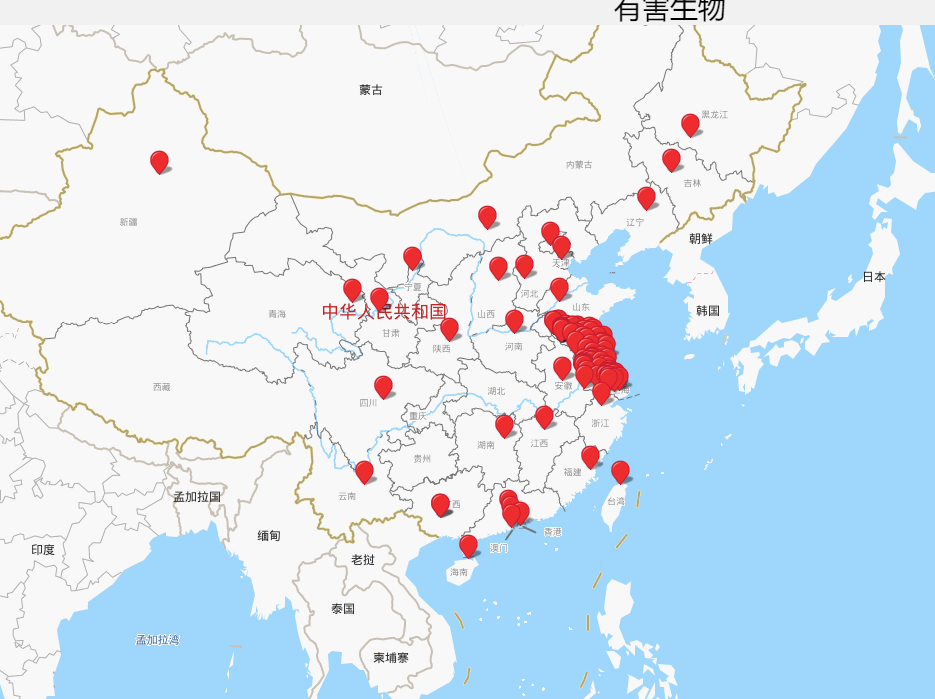
任务
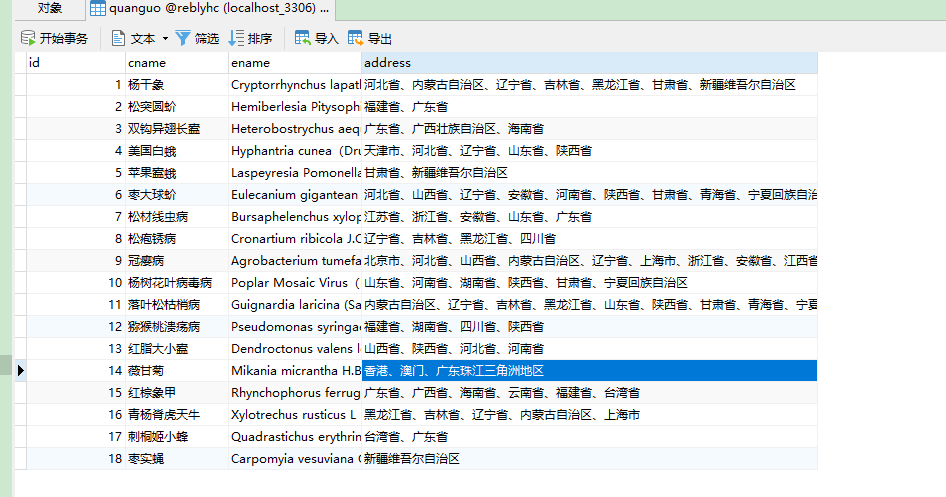
将图片中的信息改成,地名,经度,纬度,相关害虫,然后在中国地图展示
一开始用的是百度地图逆地理编码,不得不吐槽,太辣鸡了。于是选了高德(yyds)。
思路:把所有地区放到一个列表,并去重。然后遍历每个地区,判断该地区在哪个害虫对应的地区,并把害虫名字添加到相关害虫这列中。最后通过后台传值,在前端页面展示。
地区处理:
#-*- codeing = utf-8 -*-
#@Time : 2021/5/26 10:27
#@Author : 杨晓
#@File : test.py
#@Software: PyCharm
import requests
import pymysql
# 返回所有地区和昆虫数据
def get_all_diqu():
db = pymysql.connect(host="localhost", user="root", password="root", database="reblyhc")
# 使用cursor()方法获取操作游标
cursor = db.cursor()
# try:
# 执行SQL语句
cursor.execute("select * from quanguo")
# 获取所有记录列表
results = cursor.fetchall()
data = []
diqu = []
for row in results:
temp = {}
temp["id"] = row[0]
temp["cname"] = row[1]
temp["ename"] = row[2]
temp["address"] = row[3]
data.append(temp)
for one in row[3].split("、"):
diqu.append(one)
db.close()
return list(set(diqu)),data
## 插入信息
def insert_info(name,lng,lat,insects):
# port是整型 mysql是utf8 不是utf-8
con = pymysql.connect(host="localhost", port=3306, user='root', password='root', charset='utf8', db='reblyhc')
# 在pymysql中插入删除修改默认是一个事务
try:
with con.cursor() as cursor: # 获得一个游标
result = cursor.execute('insert into address values(%s,%s,%s,%s,%s)',(0,name,lng,lat,insects))
if result == 1:
print("插入成功")
con.commit()
except pymysql.MySQLError as error:
print(error)
con.rollback()
finally:
con.close()
## 通过地区返回经纬度坐标
def get_all_address(diqu,data):
for one in diqu:
parameters = {
"address": one,
"output": "json",
"key": 你申请的key值
}
response = requests.get("https://restapi.amap.com/v3/geocode/geo?parameters", params=parameters, timeout=4)
try:
answer = response.json()
if answer["status"] == '1':
print("aa")
name = one
temp = answer['geocodes'][0]['location'].split(",")
lng = float(temp[0])
lat = float(temp[1])
insects = []
## 判断害虫是否在这个地区
for row in data:
if name in row["address"]:
insects.append(row["cname"])
str = ",".join(insects)
insert_info(name, lng, lat, str)
except Exception as e:
print(one)
if __name__ == '__main__':
diqu,data = get_all_diqu()
get_all_address(diqu,data)
前端页面
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no"/>
<style type="text/css">
body,
html,
#allmap {
100%;
height: 100%;
overflow: hidden;
margin: 0;
font-family: "微软雅黑";
}
</style>
<script type="text/javascript"
src="http://api.map.baidu.com/getscript?v=2.0&ak=1CP82D4yZqwEXmsuje96jrxfKWTInh0I&services=&t=20170517145936"></script>
<script src="../static/js/jquery-1.11.3.min.js" charset="utf-8"></script>
<link rel="stylesheet" href="../static/layui/css/layui.css" media="all">
<script src="../static/layui/layui.js" charset="utf-8"></script>
<title>地图展示</title>
</head>
<body>
<blockquote class="layui-elem-quote">在本页面查看结构物的所有有害生物。</blockquote>
<h1 align="center">有害生物</h1>
<div id="allmap"></div>
</body>
</html>
<script type="text/javascript">
// 百度地图API功能
var map = new BMap.Map("allmap"); // 创建Map实例
map.addControl(new BMap.NavigationControl());
map.addControl(new BMap.ScaleControl());
map.addControl(new BMap.OverviewMapControl());
map.centerAndZoom(new BMap.Point(parseFloat("115.797429"), parseFloat("40.398826")), 6); // 初始化地图,设置中心点坐标和地图级别
//添加地图类型控件
map.addControl(new BMap.MapTypeControl({
mapTypes: [
BMAP_NORMAL_MAP,
BMAP_HYBRID_MAP
]
}));
map.setCurrentCity("江苏"); // 设置地图显示的城市 此项是必须设置的
map.enableScrollWheelZoom(true); //开启鼠标滚轮缩放
// 编写自定义函数,创建标注
function addMarker(name,point,insect_names) {
var marker = new BMap.Marker(point);
marker.addEventListener('click', function (e) {
// 通过点击的坐标创建一个点对象,添加标签
var point = new BMap.Point(e.point.lng, e.point.lat);
var opts = {
title: '<h2>'+"有害生物分布"+'</h2>',
enableMessage: false,
};
var infowindow = new BMap.InfoWindow("城市名称:"+name+"<br/>" + "经度:" + e.point.lng + "<br/>纬度:" + e.point.lat+"<br>害虫种类:"+insect_names, opts);
map.openInfoWindow(infowindow, point);
});
map.addOverlay(marker);
}
{% for one in zuobiao %}
var point = new BMap.Point({{ one['lng'] }}, {{ one['lat'] }});
addMarker('{{ one['name'] }}',point,'{{ one['insect_names'] }}');
{% endfor %}
</script>
后台代码
@app.route("/fbdt")
def fbdt():
# all_address = get_all_insect_address()
# get_all_address()
address_data = get_address_by_sort()
db = pymysql.connect(host="localhost", user="root", password="root", database="reblyhc")
# 使用cursor()方法获取操作游标
cursor = db.cursor()
# try:
# 执行SQL语句
cursor.execute("select * from address")
# 获取所有记录列表
results = cursor.fetchall()
zuobiao = []
for row in results:
temp = {}
temp["name"] = row[1]
temp["lng"] = row[2]
temp["lat"] = row[3]
if row[4] == None:
insect_names = []
for one in address_data:
for i in one["fbdq"]:
if (i in row[1]) or (row[1] in i):
insect_names.append(one["name"])
break
temp["insect_names"] = ','.join(insect_names)
else:
temp["insect_names"] = row[4]
zuobiao.append(temp)
db.close()
return render_template("dt.html",zuobiao=zuobiao)