open函数理解
open(file, mode='r', buffering=-1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
mode模式
合法mode:r、rb、r+、rb+、w、wb、w+、wb+、a、ab、a+、ab+
========= ===============================================================
Character Meaning
--------- ---------------------------------------------------------------
'r' open for reading (default) 读取: 默认打开文件用于读取
'w' open for writing, truncating the file first 写入:打开文件用于写入,如果文件不存在则创建文件,如果文件存在,则后写入内容覆盖之前内容
'x' create a new file and open it for writing 写入:创建一个新文件,并且打开写入内容(打开文件以进行独占创建。如果文件已经存在,则操作失败。)
'a' open for writing, appending to the end of the file if it exists 写入:append ,在已存在的文件后再添加内容,如果不存在,则创建一个新文件
'b' binary mode 文件格式:二进制文件
't' text mode (default) 文件格式:文本文件(默认格式)
'+' open a disk file for updating (reading and writing) 混合:打开文件进行读写更新
'U' universal newline mode (deprecated)
========= ===============================================================
w用法
#没有文件新建写入文件
In [1]: with open('train1','wt')as f: ...: f.write('today is monday')
#文件存在,再次写入内容,覆盖原来内容写入
In [2]: with open('train1','wt')as f:...: f.write('today is tuesday')
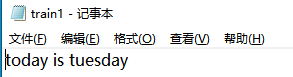
X用法
特点在新,文件存在则不能创建,那么也就没有内容覆盖这一说法
#train1存在;xt写入不成功
In [3]: with open('train1','xt')as f: ...: f.write('today is friday') ...: --------------------------------------------------------------------------- FileExistsError Traceback (most recent call last) <ipython-input-3-26ed44691e4d> in <module> ----> 1 with open('train1','xt')as f: 2 f.write('today is friday') 3 FileExistsError: [Errno 17] File exists: 'train1'
使用os.path.exist('filename')做判断,由此判断‘w’能否写入
a用法
#append:附加在文件末尾
In [4]: with open('train1','at')as f: ...: f.write('这里我们写入a模式')
#文件不存在,则创建文件:
In [5]: with open('train2','at') as f:
...: f.write('文件不存在a模式写入文件')
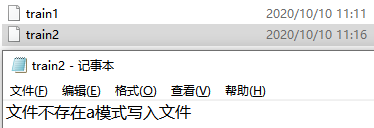
+用法
+的用法 | 文件存在是否存在 | 读取写入内容时 |
r+ | 不存在时报错 | 写入时内容添加在原内容后 |
w+ | 不存在时创建文件 | 写入时内容覆盖原内容 |
a+ | 不存在时创建文件 | 写入时内容添加在原内容后 |
r+用法
1.读取内容,写入时,添加在原内容之后
2.文件不存在报错
In [25]: f=open('train3','r+') In [26]: f.read() Out[26]: '古潼京七星鲁王宫' In [27]: f.write('秦岭神树') Out[27]: 4 In [28]: f.seek(0) Out[28]: 0 In [29]: f.read() Out[29]: '古潼京七星鲁王宫秦岭神树'
In [30]: f=open('train4','r+')
---------------------------------------------------------------------------
FileNotFoundError Traceback (most recent call last)
<ipython-input-30-94bce8ea3946> in <module>
----> 1 f=open('train4','r+')
FileNotFoundError: [Errno 2] No such file or directory: 'train4'
a+用法
1.读取内容,写入时,添加在原内容之后
2.文件不存在,新建文件
#文件不存在会新建内容
In [33]: f=open('train4','a+') In [34]: f.write('云顶天宫') Out[34]: 4 In [35]: f.read() Out[35]: '' In [36]: f.seek(0) Out[36]: 0 In [37]: f.read() Out[37]: '云顶天宫' In [38]: f.close()
#文件存在时,写入文件,a+会把内容append在后
In [43]: f.write('张家古楼')
out[43]: 4
In [44]: f.seek(0)
Out[44]: 0
In [45]: f.read()
Out[45]: '云顶天宫张家古楼'
w+用法
1.读取内容,写入时,覆盖内容
2.文件不存在,新建文件
#文件不存在时新建文件
In [48]: f=open('train5','w+') In [49]: f.write('阴山古楼') Out[49]: 4 In [50]: f.seek(0) Out[50]: 0 In [51]: f.read() Out[51]: '阴山古楼' In [52]: f.close()
#文件存在,写入时覆盖原内容
In [53]: f=open('train5','w+')
In [54]: f.write('塔木坨')
Out[54]: 3
In [55]: f.seek()
In [56]: f.seek(0)
Out[56]: 0
In [57]: f.read()
Out[57]: '塔木坨'
seek的函数
由于+应用中会使用读写操作会涉及指针偏移,为便利读取,故需设定指针位置,需应用seek函数
seek(offset[, whence]) ,offset是相对于某个位置的偏移量。位置由whence决定,默认whence=0,从开头起;whence=1,从当前位置算起;whence=2相对于文件末尾移动,通常offset取负值。
close()关闭
当我们写文件时,操作系统往往不会立刻把数据写入磁盘,而是放到内存缓存起来,空闲的时候再慢慢写入。只有调用close()方法时,操作系统才保证把没有写入的数据全部写入磁盘。忘记调用close()的后果是数据可能只写了一部分到磁盘,剩下的丢失了。
这种方法并不完全安全。如果对文件执行某些操作时发生异常,则代码将退出而不关闭文件。
一种更安全的方法是使用try ... finally块
try: f = open("test.txt", encoding = 'utf-8') # perform file operations finally: f.close()
这样,即使出现引发导致程序流停止的异常,我们也可以保证文件已正确关闭。
关闭文件的最佳方法是使用with
语句。这样可以确保在with
退出语句内的块时关闭文件。
我们不需要显式调用该close()
方法。它是在内部完成的。
with open("test.txt", encoding = 'utf-8') as f: # perform file operations