- 功能要求
角色:学校、学员、课程、讲师
要求:
1. 创建学校
2. 创建课程
3. 课程包含,周期,价格,通过学校创建课程
4. 通过学校创建班级, 班级关联课程、讲师
5. 创建学员时,选择学校,关联班级
5. 创建讲师角色时要关联学校,
6. 提供两个角色接口
6.1 学员视图, 可以注册, 交学费, 选择班级,
6.2 讲师视图, 讲师可管理自己的班级, 上课时选择班级, 查看班级学员列表 , 创建学员的成绩
6.3 管理视图,创建讲师, 创建班级,创建课程
7. 上面的操作产生的数据都通过pickle序列化保存到文件里
8. 增加日志文件,能同时输出到控制台
9. 用户认证采用装饰
10.增加try捕捉错误信息
- 开发环境
- python 3.6.1
- PyCharm 2016.2.3
- 数据流图与业务功能图
- 主程序数据流图
2.业务功能图
- 目录结构
- 程序设计

1 #!/usr/bin/env python 2 #coding=utf-8 3 __author__ = 'yinjia' 4 5 6 import os 7 8 BASE_DIR = os.path.dirname(os.path.dirname(__file__)) 9 10 BASE_ADMIN_DIR = os.path.join(BASE_DIR, "db", "admin") 11 12 BASE_STUDENTS_DIR = os.path.join(BASE_DIR, "db", "students") 13 14 BASE_TEACHER_DIR = os.path.join(BASE_DIR,"db","teacher") 15 16 TEACHER_DB_DIR = os.path.join(BASE_DIR, "db", "teacher_list") 17 18 COURSE_DB_DIR = os.path.join(BASE_DIR, "db", "course_list") 19 20 SHOOL_DB_DIR = os.path.join(BASE_DIR,"db", "shool_list") 21 22 STUDENTS_DB_DIR = os.path.join(BASE_DIR,"db", "students_list") 23 24 SCHOOLCLASS_DB_DIR = os.path.join(BASE_DIR,'db','schoolclass_list') 25 26 SCORE_DB_DIR = os.path.join(BASE_DIR,'db','score_list') 27 28 BASE_LOG_DIR = os.path.join(BASE_DIR,'log','AAP_')

1 #!/usr/bin/env python 2 #coding=utf-8 3 __author__ = 'yinjia' 4 5 import os,sys,time,logging,pickle 6 sys.path.append(os.path.dirname(os.path.dirname(__file__))) 7 from lib import models 8 from config import settings 9 from lib.models import * 10 from bin import students 11 from bin import admin 12 from bin import teacher 13 14 15 def Auth(type): 16 """ 17 统一入口用户注册、认证 18 :param type: 判断属于哪认证类型:students、admin、teacher 19 :return: 返回认证结果 20 """ 21 def inner(func): 22 def wrapper(): 23 logger = Logger('auth').getlog() 24 auth_msg = ["注册", "登录"] 25 if type == "students_auth": 26 func() 27 for x, y in enumerate(auth_msg, 1): 28 print("%s%s%s" % (x, ".", y)) 29 while True: 30 inp = str(input("33[35;0m请按序号选择[输入q|Q退出]:33[0m")).strip().lower() 31 if inp == "1": 32 use = str(input("33[36;0m请输入用户名:33[0m")).strip() 33 pwd = str(input("33[36;0m请输入密码:33[0m")).strip() 34 obj = models.Students() 35 if obj.register(use,pwd): 36 logger.error("[用户名]" + use + ":不能注册,当前已存在账户!") 37 else: 38 logger.info("[用户名]" + use + ":注册成功") 39 elif inp == "2": 40 use = str(input("33[36;0m请输入用户名:33[0m")).strip() 41 pwd = str(input("33[36;0m请输入密码:33[0m")).strip() 42 if os.path.exists(os.path.join(settings.BASE_STUDENTS_DIR, use)): 43 try: 44 students_obj = pickle.load(open(os.path.join(settings.BASE_STUDENTS_DIR,use),'rb')) 45 except IOError as e: 46 logger.error(e) 47 if students_obj.login(use,pwd): 48 logger.info("[用户名]" + use + ":登录成功!") 49 students.main(students_obj) 50 else: 51 logger.error("[用户]" + use + ":密码错误!") 52 else: 53 logger.error("[用户名]" + use + "不存在!") 54 elif inp == "q": 55 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 56 sys.exit() 57 else: 58 logger.error("无效选择!") 59 60 elif type == "admin_auth": 61 func() 62 for x, y in enumerate(auth_msg, 1): 63 print("%s%s%s" % (x, ".", y)) 64 while True: 65 inp = str(input("33[35;0m请按序号选择[输入q|Q退出]:33[0m")).strip().lower() 66 if inp == "1": 67 use = str(input("33[36;0m请输入用户名:33[0m")).strip() 68 pwd = str(input("33[36;0m请输入密码:33[0m")).strip() 69 obj = models.Admin() 70 if obj.register(use,pwd): 71 logger.error("[用户名]" + use + ":不能注册,当前已存在账户!") 72 else: 73 logger.info("[用户名]" + use + ":注册成功") 74 elif inp == "2": 75 use = str(input("33[36;0m请输入用户名:33[0m")).strip() 76 pwd = str(input("33[36;0m请输入密码:33[0m")).strip() 77 if os.path.exists(os.path.join(settings.BASE_ADMIN_DIR, use)): 78 try: 79 admin_obj = pickle.load(open(os.path.join(settings.BASE_ADMIN_DIR,use),'rb')) 80 except IOError as e: 81 logger.error(e) 82 if admin_obj.login(use,pwd): 83 logger.info("[用户名]" + use + ":登录成功") 84 admin.main(admin_obj) 85 else: 86 logger.error("[用户名]" + use + ":密码错误!") 87 else: 88 logger.error("[用户]" + use + "不存在!") 89 90 elif inp == "q": 91 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 92 sys.exit() 93 else: 94 logger.error("无效选择!") 95 96 elif type == "teacher_auth": 97 func() 98 for x, y in enumerate(auth_msg, 1): 99 print("%s%s%s" % (x, ".", y)) 100 while True: 101 inp = str(input("33[35;0m请按序号选择[输入q|Q退出]:33[0m")).strip().lower() 102 if inp == "1": 103 use = str(input("33[36;0m请输入用户名:33[0m")).strip() 104 pwd = str(input("33[36;0m请输入密码:33[0m")).strip() 105 obj = models.TeacherAuth() 106 if obj.register(use,pwd): 107 logger.error("[用户名]" + use + ":不能注册,当前已存在账户!") 108 else: 109 logger.info("[用户名]" + use + ":注册成功") 110 elif inp == "2": 111 use = str(input("33[36;0m请输入用户名:33[0m")).strip() 112 pwd = str(input("33[36;0m请输入密码:33[0m")).strip() 113 if os.path.exists(os.path.join(settings.BASE_TEACHER_DIR, use)): 114 try: 115 teacher_obj = pickle.load(open(os.path.join(settings.BASE_TEACHER_DIR,use),'rb')) 116 except IOError as e: 117 logger.error(e) 118 if teacher_obj.login(use,pwd): 119 logger.info("[用户名]" + use + ":登录成功") 120 teacher.main(teacher_obj) 121 else: 122 logger.error("[用户名]" + use + ":密码错误!") 123 else: 124 logger.error("[用户名]" + use + "不存在!") 125 126 elif inp == "q": 127 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 128 sys.exit() 129 else: 130 logger.error("无效选择!") 131 else: 132 pass 133 return wrapper 134 return inner 135 136 137 @Auth(type="students_auth") 138 def students_auth(): 139 print("33[32;0m学生登录认证33[0m".center(40,"*")) 140 return True 141 142 @Auth(type="admin_auth") 143 def admin_auth(): 144 print("33[32;0m管理员登录认证33[0m".center(40,"*")) 145 return True 146 147 @Auth(type="teacher_auth") 148 def teacher_auth(): 149 print("33[32;0m讲师登录认证33[0m".center(40,"*")) 150 return True 151 152 def main(): 153 main_msg = ["学生视图", "讲师视图", "管理视图","退出"] 154 print("33[32;0m学员选课系统33[0m".center(40, "*")) 155 for i, j in enumerate(main_msg, 1): 156 print("%s%s%s" % (i, ".", j)) 157 while True: 158 inp = str(input("33[35;0m请按序号选择:33[0m")).strip() 159 if inp == "1": 160 students_auth() 161 elif inp == "2": 162 teacher_auth() 163 elif inp == "3": 164 admin_auth() 165 elif inp == "4": 166 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 167 sys.exit() 168 else: 169 print("无效选择!")

1 #!/usr/bin/env python 2 #coding=utf-8 3 __author__ = 'yinjia' 4 5 import os,sys,time,logging,pickle 6 sys.path.append(os.path.dirname(os.path.dirname(__file__))) 7 from lib import models 8 from config import settings 9 from lib.models import * 10 from bin import auth 11 12 logger = Logger('admin').getlog() 13 14 def create_shools(admin_obj): 15 """ 16 创建学校 17 :param admin_obj: 18 :return: 19 """ 20 school_list = [] 21 while True: 22 school_name = str(input("33[36;0m请输入校区名[输入q退出]:33[0m")).strip().lower() 23 if school_name == "q": 24 break 25 school_addr = str(input("33[36;0m请输入校区地址:33[0m")).strip() 26 obj = models.School(school_name,school_addr,admin_obj) 27 school_list.append(obj) 28 if os.path.exists(settings.SHOOL_DB_DIR): 29 try: 30 exists_list = pickle.load(open(settings.SHOOL_DB_DIR,'rb')) 31 school_list.extend(exists_list) 32 except IOError as e: 33 logger.error(e) 34 try: 35 pickle.dump(school_list,open(settings.SHOOL_DB_DIR,'wb')) 36 except IOError as e: 37 logger.error(e) 38 39 def create_schoolclass(admin_obj): 40 """ 41 创建班级 42 :param admin_obj: 43 :return: 44 """ 45 schoolclass_list = [] 46 print(' 序号 校区 校址 创建时间 管理员') 47 print('------------------------------------------------------') 48 school_list = pickle.load(open(settings.SHOOL_DB_DIR, 'rb')) 49 for num, item in enumerate(school_list, 1): 50 print("%3s%5s%5s%25s%10s" % (num, item.school_name, item.school_addr, 51 item.create_time, item.create_admin.username)) 52 while True: 53 schoolclass_name = str(input("33[36;0m请输入班级名[输入q退出]:33[0m")).strip().lower() 54 if schoolclass_name == "q": 55 break 56 school_num = str(input("33[36;0m请序号选择校区:33[0m")).strip() 57 obj = models.SchoolClass(school_list[int(school_num) - 1],schoolclass_name,admin_obj) 58 schoolclass_list.append(obj) 59 if os.path.exists(settings.SCHOOLCLASS_DB_DIR): 60 try: 61 exists_list = pickle.load(open(settings.SCHOOLCLASS_DB_DIR,'rb')) 62 schoolclass_list.extend(exists_list) 63 except IOError as e: 64 logger.error(e) 65 try: 66 pickle.dump(schoolclass_list,open(settings.SCHOOLCLASS_DB_DIR,'wb')) 67 except IOError as e: 68 logger.error(e) 69 70 def create_teacher(admin_obj): 71 """ 72 创建讲师 73 :param admin_obj: 74 :return: 75 """ 76 teacher_list = [] 77 print(' 序号 校区 班级 创建时间 管理员') 78 print('------------------------------------------------------') 79 school_list = pickle.load(open(settings.SCHOOLCLASS_DB_DIR, 'rb')) 80 for num, item in enumerate(school_list, 1): 81 print("%3s%5s%8s%25s%10s" % (num, item.schoolBJ.school_name, item.schoolclass_name, 82 item.create_time, item.create_admin.username)) 83 while True: 84 teacher_name = str(input("33[36;0m请输入讲师姓名[输入q退出]:33[0m")).strip().lower() 85 if teacher_name == "q": 86 break 87 age = str(input("33[36;0m请输入讲师年龄:33[0m")).strip() 88 salary = str(input("33[36;0m请输入讲师工资:33[0m")).strip() 89 num = str(input("33[36;0m请序号选择班级:33[0m")).strip() 90 obj = models.Teacher(school_list[int(num) - 1],school_list[int(num) - 1],teacher_name,age,salary,admin_obj) 91 teacher_list.append(obj) 92 if os.path.exists(settings.TEACHER_DB_DIR): 93 try: 94 exists_list = pickle.load(open(settings.TEACHER_DB_DIR,'rb')) 95 teacher_list.extend(exists_list) 96 except IOError as e: 97 logger.error(e) 98 try: 99 pickle.dump(teacher_list,open(settings.TEACHER_DB_DIR,'wb')) 100 except IOError as e: 101 logger.error(e) 102 def create_course(admin_obj): 103 """ 104 创建课程 105 :param admin_obj: 106 :return: 107 """ 108 course_list = [] 109 print(' 序号 校区 班级 讲师 年龄 工资 创建时间 管理员') 110 print('---------------------------------------------------------------------------') 111 teacher_list = pickle.load(open(settings.TEACHER_DB_DIR, 'rb')) 112 for num, item in enumerate(teacher_list, 1): 113 print("%3s%5s%8s%5s%4s%11s%23s%10s" % ( 114 num, item.schoolname.schoolBJ.school_name, item.schoolclass.schoolclass_name, 115 item.teacher_name, item.age, item.salary, 116 item.create_time, item.create_admin.username)) 117 while True: 118 course_name = str(input("33[36;0m请输入课程名[输入q退出]:33[0m")).strip().lower() 119 if course_name == "q": 120 break 121 course_cost = str(input("33[36;0m请输入课时费:33[0m")).strip() 122 course_cycle = str(input("33[36;0m请输入课程周期:33[0m")).strip() 123 num = str(input("33[36;0m请序号选择校区:33[0m")).strip() 124 obj = models.Course(teacher_list[int(num) - 1], teacher_list[int(num) - 1], 125 teacher_list[int(num) - 1],course_name,course_cost,course_cycle, admin_obj) 126 course_list.append(obj) 127 if os.path.exists(settings.COURSE_DB_DIR): 128 try: 129 exists_list = pickle.load(open(settings.COURSE_DB_DIR, 'rb')) 130 course_list.extend(exists_list) 131 except IOError as e: 132 logger.error(e) 133 try: 134 pickle.dump(course_list, open(settings.COURSE_DB_DIR, 'wb')) 135 except IOError as e: 136 logger.error(e) 137 138 def main(admin_obj): 139 logger = Logger('auth').getlog() 140 while True: 141 print("33[32;0m综合管理中心33[0m".center(40, "*")) 142 msg = ["创建校区", "查看校区详情","创建班级", "查看班级详情","创建讲师", '查看讲师详情',"创建课程","查看课程详情"] 143 for x, y in enumerate(msg, 1): 144 print("%s%s%s" % (x, ".", y)) 145 inp = str(input("33[35;0m请按序号选择[输入q|Q退出]:33[0m")).strip().lower() 146 if inp == '1': 147 create_shools(admin_obj) 148 elif inp == '2': 149 print(' 序号 校区 校址 创建时间 管理员') 150 print('------------------------------------------------------') 151 info_list = pickle.load(open(settings.SHOOL_DB_DIR, 'rb')) 152 for num, item in enumerate(info_list, 1): 153 print("%3s%5s%5s%25s%10s" % (num, item.school_name, item.school_addr, 154 item.create_time, item.create_admin.username)) 155 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 156 elif inp == '3': 157 create_schoolclass(admin_obj) 158 elif inp == '4': 159 print(' 序号 校区 班级 创建时间 管理员') 160 print('------------------------------------------------------') 161 school_list = pickle.load(open(settings.SCHOOLCLASS_DB_DIR, 'rb')) 162 for num, item in enumerate(school_list, 1): 163 print("%3s%5s%8s%25s%10s" % (num, item.schoolBJ.school_name, item.schoolclass_name, 164 item.create_time, item.create_admin.username)) 165 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 166 elif inp == '5': 167 create_teacher(admin_obj) 168 elif inp == '6': 169 print(' 序号 校区 班级 讲师 年龄 工资 创建时间 管理员') 170 print('---------------------------------------------------------------------------') 171 teacher_list = pickle.load(open(settings.TEACHER_DB_DIR, 'rb')) 172 for num, item in enumerate(teacher_list, 1): 173 print("%3s%5s%8s%5s%4s%11s%23s%10s" % (num, item.schoolname.schoolBJ.school_name, item.schoolclass.schoolclass_name, 174 item.teacher_name, item.age, item.salary, 175 item.create_time, item.create_admin.username)) 176 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 177 elif inp == '7': 178 create_course(admin_obj) 179 elif inp == '8': 180 print(' 序号 校区 班级 讲师 课程名 课时费 周期 创建时间 管理员') 181 print('--------------------------------------------------------------------------------------') 182 teacher_list = pickle.load(open(settings.COURSE_DB_DIR, 'rb')) 183 for num, item in enumerate(teacher_list, 1): 184 print("%3s%5s%8s%5s%8s%8s%9s%23s%10s" % ( 185 num, item.course_schoolname.schoolname.schoolBJ.school_name, item.course_schoolclass.schoolclass.schoolclass_name, 186 item.course_teacher_name.teacher_name, 187 item.course_name,item.cost,item.cycle,item.create_time, item.create_admin.username)) 188 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 189 elif inp == 'q': 190 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 191 sys.exit() 192 else: 193 logger.error("无效选择!") 194 195 def register(): 196 auth.main()

1 #!/usr/bin/env python 2 #coding=utf-8 3 __author__ = 'yinjia' 4 5 import os,sys,time,logging,pickle 6 sys.path.append(os.path.dirname(os.path.dirname(__file__))) 7 from lib import models 8 from config import settings 9 from lib.models import * 10 from bin import auth,teacher 11 logger = Logger('admin').getlog() 12 13 def select_course(students_obj): 14 """ 15 选择课程 16 :param students_obj: 17 :return: 18 """ 19 print(' 序号 校区 班级 讲师 课程名 课时费 周期 ') 20 print('-------------------------------------------------------') 21 try: 22 course_list = pickle.load(open(settings.COURSE_DB_DIR, 'rb')) 23 except IOError as e: 24 logger.error(e) 25 else: 26 for num, item in enumerate(course_list, 1): 27 print("%3s%5s%8s%5s%8s%8s%9s" % ( 28 num, item.course_schoolname.schoolname.schoolBJ.school_name, 29 item.course_schoolclass.schoolclass.schoolclass_name, 30 item.course_teacher_name.teacher_name, 31 item.course_name, item.cost, item.cycle)) 32 while True: 33 num = str(input("33[36;0m请序号选择课程名[输入q退出]:33[0m")).strip().lower() 34 if num == "q": 35 break 36 select_course_obj = course_list[int(num) - 1] 37 students_obj.course_list.append(select_course_obj) 38 try: 39 pickle.dump(students_obj, open(os.path.join(settings.BASE_STUDENTS_DIR,students_obj.username),'wb')) 40 except IOError as e: 41 logger.error(e) 42 43 def course_info(students_obj): 44 """ 45 查看选课信息 46 :param students_obj: 47 :return: 48 """ 49 print(' 序号 校区 班级 讲师 课程名 课时费 周期 ') 50 print('-------------------------------------------------------') 51 for num, item in enumerate(students_obj.course_list, 1): 52 print("%3s%5s%8s%5s%8s%8s%9s" % ( 53 num, item.course_schoolname.schoolname.schoolBJ.school_name, 54 item.course_schoolclass.schoolclass.schoolclass_name, 55 item.course_teacher_name.teacher_name, 56 item.course_name, item.cost, item.cycle)) 57 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 58 59 def result_info(students_obj): 60 """ 61 查看学习详情 62 :param students_obj: 63 :return: 64 """ 65 print(' 学员 校区 班级 课程 成绩') 66 print('---------------------------------') 67 try: 68 students_dic = pickle.load(open(settings.SCORE_DB_DIR, 'rb')) 69 except IOError as e: 70 logger.error(e) 71 else: 72 for i, item in students_dic.items(): 73 for x, y in item.score_schoolname.items(): 74 print("%3s%6s%9s%5s%5s" % (x.username, y.course_schoolname.schoolname.schoolBJ.school_name, 75 y.course_schoolclass.schoolclass.schoolclass_name, y.course_name, item.score)) 76 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 77 78 def study(students_obj): 79 """ 80 学员上课 81 :param students_obj: 82 :return: 83 """ 84 print(' 序号 校区 班级 讲师 课程名 课时费 周期 ') 85 print('-------------------------------------------------------') 86 for num, item in enumerate(students_obj.course_list, 1): 87 print("%3s%5s%8s%5s%8s%8s%9s" % ( 88 num, item.course_schoolname.schoolname.schoolBJ.school_name, 89 item.course_schoolclass.schoolclass.schoolclass_name, 90 item.course_teacher_name.teacher_name, 91 item.course_name, item.cost, item.cycle)) 92 while True: 93 num = str(input("33[36;0m请按序号选择课程上课[输入q退出]:33[0m")).strip().lower() 94 if num == "q": 95 break 96 obj = students_obj.course_list[int(num) - 1] 97 if students_obj in students_obj.study_dict.keys(): 98 students_obj.study_dict[students_obj].append(obj) 99 else: 100 students_obj.study_dict[students_obj] = obj 101 try: 102 pickle.dump(students_obj.study_dict, open(settings.STUDENTS_DB_DIR, 'wb')) 103 except IOError as e: 104 logger.error(e) 105 106 def main(students_obj): 107 while True: 108 print("33[32;0m学员学习中心33[0m".center(40, "*")) 109 msg = ['选课','上课','查看选课信息','查看学习成绩'] 110 for x, y in enumerate(msg, 1): 111 print("%s%s%s" % (x, ".", y)) 112 inp = str(input("33[35;0m请按序号选择[输入q|Q退出]:33[0m")).strip().lower() 113 if inp == '1': 114 select_course(students_obj) 115 elif inp == '2': 116 study(students_obj) 117 elif inp == '3': 118 course_info(students_obj) 119 elif inp == '4': 120 result_info(students_obj) 121 elif inp == 'q': 122 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 123 sys.exit() 124 else: 125 logger.error("无效选择!") 126 127 def register(): 128 auth.main()

1 #!/usr/bin/env python 2 #coding=utf-8 3 __author__ = 'yinjia' 4 5 6 import os,sys,time,logging,pickle 7 sys.path.append(os.path.dirname(os.path.dirname(__file__))) 8 from lib import models 9 from config import settings 10 from lib.models import * 11 from bin import auth 12 logger = Logger('admin').getlog() 13 14 def Create_Score(teacher_obj): 15 score_dic = {} 16 print(' 学员 校区 班级 课程 ') 17 print('------------------------------') 18 try: 19 students_dic = pickle.load(open(settings.STUDENTS_DB_DIR, 'rb')) 20 except IOError as e: 21 logger.error(e) 22 else: 23 for i, item in students_dic.items(): 24 print(i.username,item.course_schoolname.schoolname.schoolBJ.school_name, 25 item.course_schoolclass.schoolclass.schoolclass_name, 26 item.course_name) 27 while True: 28 students_name = str(input("33[36;0m请输入学员[输入q退出]:33[0m")).strip() 29 if students_name == "q": 30 break 31 score = str(input("33[36;0m请输入学员成绩:33[0m")).strip() 32 obj = models.Score(students_name,students_dic,students_dic,students_dic,score) 33 34 if teacher_obj in score_dic.keys(): 35 score_dic[teacher_obj].append(obj) 36 else: 37 score_dic[teacher_obj] = obj 38 if os.path.exists(settings.SCORE_DB_DIR): 39 try: 40 exists_dic = pickle.load(open(settings.SCORE_DB_DIR, 'rb')) 41 except IOError as e: 42 logger.error(e) 43 else: 44 if teacher_obj in exists_dic.keys(): 45 score_dic[teacher_obj].append(exists_dic) 46 try: 47 pickle.dump(score_dic, open(settings.SCORE_DB_DIR, 'wb')) 48 except IOError as e: 49 logger.error(e) 50 51 52 def show_info(teacher_obj): 53 print(' 学员 校区 班级 课程 成绩') 54 print('---------------------------------') 55 try: 56 students_dic = pickle.load(open(settings.SCORE_DB_DIR, 'rb')) 57 except IOError as e: 58 logger.error(e) 59 else: 60 for i, item in students_dic.items(): 61 for x,y in item.score_schoolname.items(): 62 print("%3s%6s%9s%5s%5s" % (x.username,y.course_schoolname.schoolname.schoolBJ.school_name, 63 y.course_schoolclass.schoolclass.schoolclass_name,y.course_name,item.score)) 64 input("33[35;0m>>>>>>>>>按任意键退出...33[0m") 65 66 def main(teacher_obj): 67 while True: 68 print("33[32;0m学员学习中心33[0m".center(40, "*")) 69 msg = ['创建学员成绩','查看学员信息'] 70 for x, y in enumerate(msg, 1): 71 print("%s%s%s" % (x, ".", y)) 72 inp = str(input("33[35;0m请按序号选择[输入q|Q退出]:33[0m")).strip().lower() 73 if inp == '1': 74 Create_Score(teacher_obj) 75 elif inp == '2': 76 show_info(teacher_obj) 77 elif inp == 'q': 78 print("33[32;0m谢谢您的光临,再见!33[0m".center(40, "*")) 79 sys.exit() 80 else: 81 logger.error("无效选择!") 82 83 def register(): 84 auth.main()
- 类模板定义
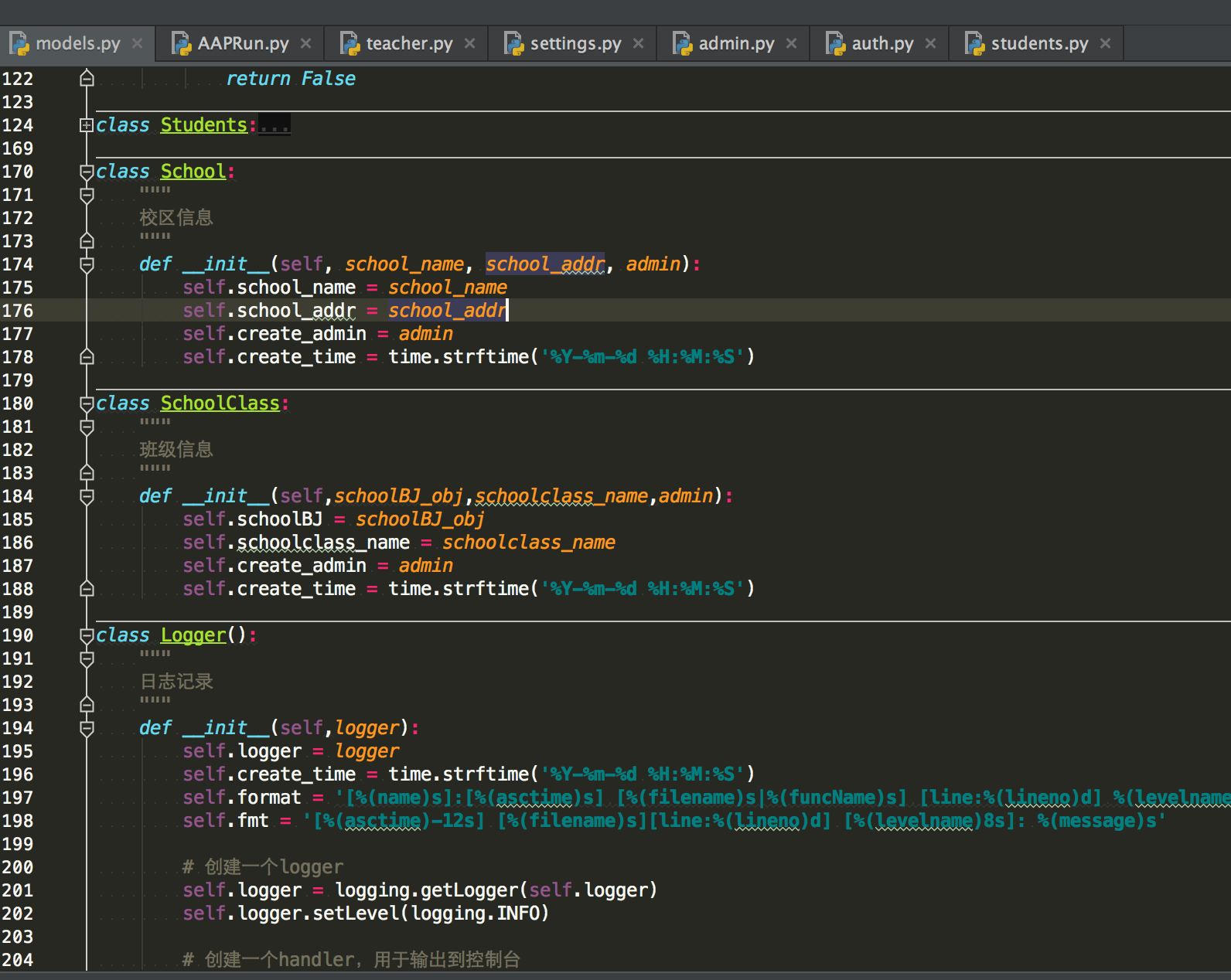
- 效果演示
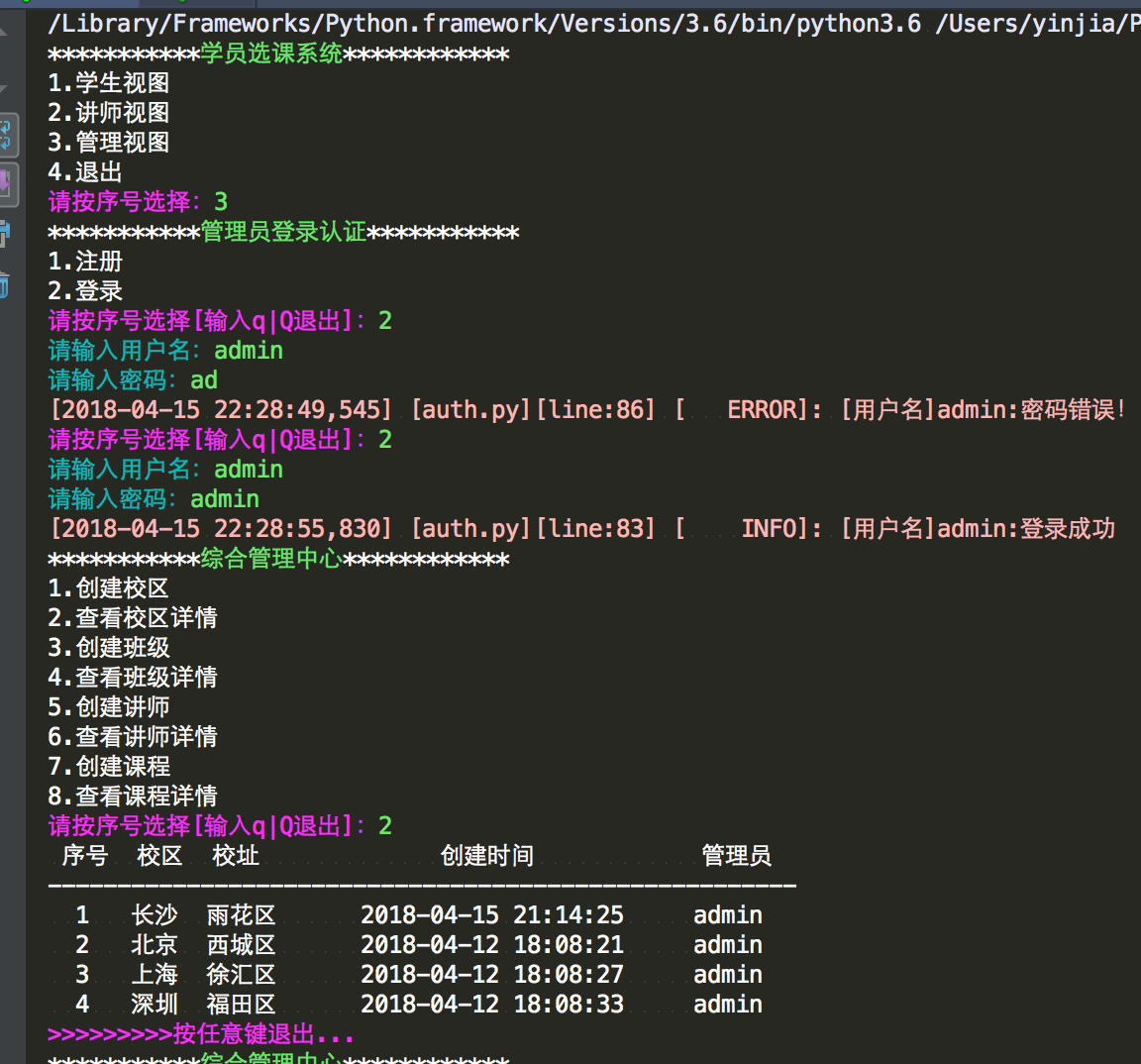
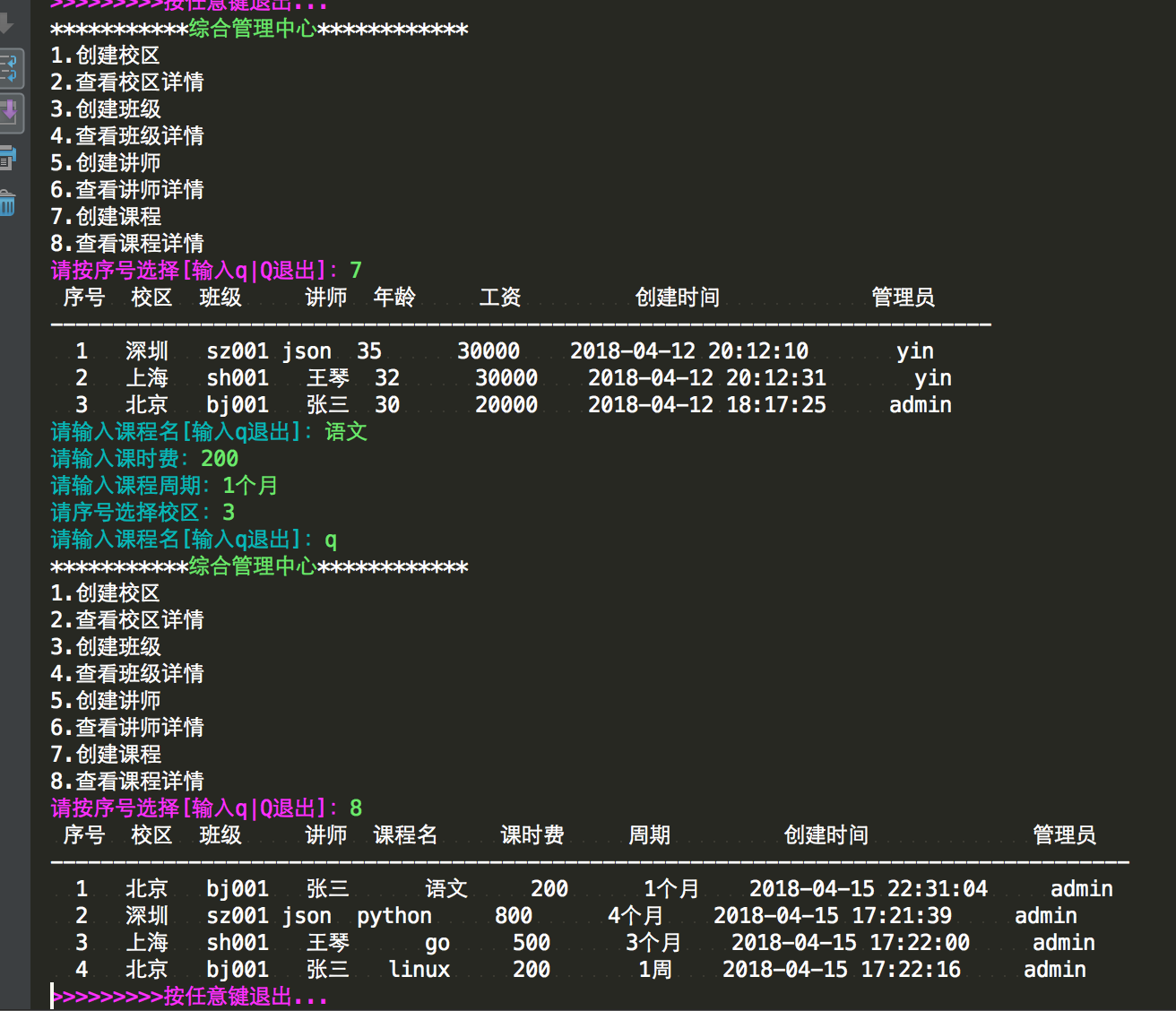