public class SoundPlayerGUI extends JFrame
implements ChangeListener, ActionListener
{
private static final String VERSION = "Version 1.0";
private static final String AUDIO_DIR = "audio";
private JList fileList;
private JSlider slider;
private JLabel infoLabel;
public static void main(String[] args)
{
SoundPlayerGUI gui = new SoundPlayerGUI();
}
public SoundPlayerGUI()
{
super("SoundPlayer");
String[] audioFileNames = findFiles(AUDIO_DIR, null);
makeFrame(audioFileNames);
}
private void play()
{
String filename = (String)fileList.getSelectedValue();
if(filename == null) { // nothing selected
return;
}
slider.setValue(0);
boolean successful = player.play(new File(AUDIO_DIR, filename));
}
private void showInfo(String message)
{
infoLabel.setText(message);
}
private void showAbout()
{
JOptionPane.showMessageDialog(this,
"SoundPlayer
" + VERSION,
"About SoundPlayer",
JOptionPane.INFORMATION_MESSAGE);
}
private String[] findFiles(String dirName, String suffix)
{
File dir = new File(dirName);
if(dir.isDirectory()) {
String[] allFiles = dir.list();
if(suffix == null) {
return allFiles;
}
else {
List<String> selected = new ArrayList<String>();
for(String filename : allFiles) {
if(filename.endsWith(suffix)) {
selected.add(filename);
}
}
return selected.toArray(new String[selected.size()]);
}
}
else {
System.out.println("");
return null;
}
}
public void stateChanged(ChangeEvent evt)
{
player.seek(slider.getValue());
}
public void actionPerformed(ActionEvent evt)
{
JComboBox cb = (JComboBox)evt.getSource();
String format = (String)cb.getSelectedItem();
if(format.equals("all formats")) {
format = null;
}
fileList.setListData(findFiles(AUDIO_DIR, format));
}
private void makeFrame(String[] audioFiles)
{
JPanel contentPane = (JPanel)getContentPane();
contentPane.setBorder(new EmptyBorder(0, 0, 0, 0));
makeMenuBar();
contentPane.setLayout(new BorderLayout(0, 0));
contentPane.setBackground(new Color(0,0,0));
JPanel leftPane = new JPanel();
{
leftPane.setLayout(new BorderLayout(188, 1));
String[] formats = { "all formats", ".wav", ".au", ".aif",".mp3" };
// Create the combo box.
JComboBox formatList = new JComboBox(formats);
formatList.addActionListener(this);
leftPane.add(formatList, BorderLayout.NORTH);
// Create the scrolled list for file names
fileList = new JList(audioFiles);
fileList.setForeground(new Color(140,171,226));
fileList.setBackground(new Color(0,0,0));
fileList.setSelectionBackground(new Color(87,49,134));
fileList.setSelectionForeground(new Color(140,171,226));
JScrollPane scrollPane = new JScrollPane(fileList);
scrollPane.setColumnHeaderView(new JLabel("本地歌曲"));
leftPane.add(scrollPane, BorderLayout.CENTER);
JButton button = new JButton(new ImageIcon("play.jpg"));
button.setPressedIcon(new ImageIcon ("play1.jpg"));
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) { play(); }
});
slider = new JSlider(0, 100, 0);
TitledBorder border = new TitledBorder("Seek");
border.setTitleColor(Color.white);
slider.setBorder(new CompoundBorder(new EmptyBorder(6, 10, 10, 10), border));
slider.addChangeListener(this);
slider.setBackground(Color.white);
slider.setMajorTickSpacing(25);
slider.setPaintTicks(true);
JPanel toolbar = new JPanel();
toolbar.setLayout(new GridLayout(2, 1));
toolbar.add(button);
toolbar.add(slider);
leftPane.add(toolbar,BorderLayout.NORTH);
}
contentPane.add(leftPane, BorderLayout.WEST);
JPanel picPane=new JPanel();
{
JLabel pic=new JLabel(new ImageIcon("zhuomian.jpg"));
picPane.add(pic, BorderLayout.CENTER);
contentPane.add(picPane,BorderLayout.CENTER);
}
JPanel centerPane = new JPanel();
{
centerPane.setLayout(new BorderLayout(188, 8));
JLabel image = new JLabel(new ImageIcon("u=2786397887,625919022&fm=21&gp=0.jpg"));
centerPane.add(image, BorderLayout.NORTH);
centerPane.setBackground(Color.BLUE);
infoLabel = new JLabel(new ImageIcon("zhi.jpg"));
centerPane.add(infoLabel, BorderLayout.CENTER);
}
contentPane.add(centerPane, BorderLayout.EAST);
// Create the toolbar with the buttons
pack();
// place this frame at the center of the screen and show
Dimension d = Toolkit.getDefaultToolkit().getScreenSize();
setLocation(d.width/2 - getWidth()/2, d.height/2 - getHeight()/2);
setVisible(true);}
private void makeMenuBar()
{
final int SHORTCUT_MASK =
Toolkit.getDefaultToolkit().getMenuShortcutKeyMask();
JMenuBar menubar = new JMenuBar();
setJMenuBar(menubar);
JMenu menu;
JMenuItem item;
menu = new JMenu("File");
menubar.add(menu);
item = new JMenuItem("Quit");
item.setAccelerator(KeyStroke.getKeyStroke(KeyEvent.VK_Q, SHORTCUT_MASK));
item.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) { quit(); }
});
menu.add(item);
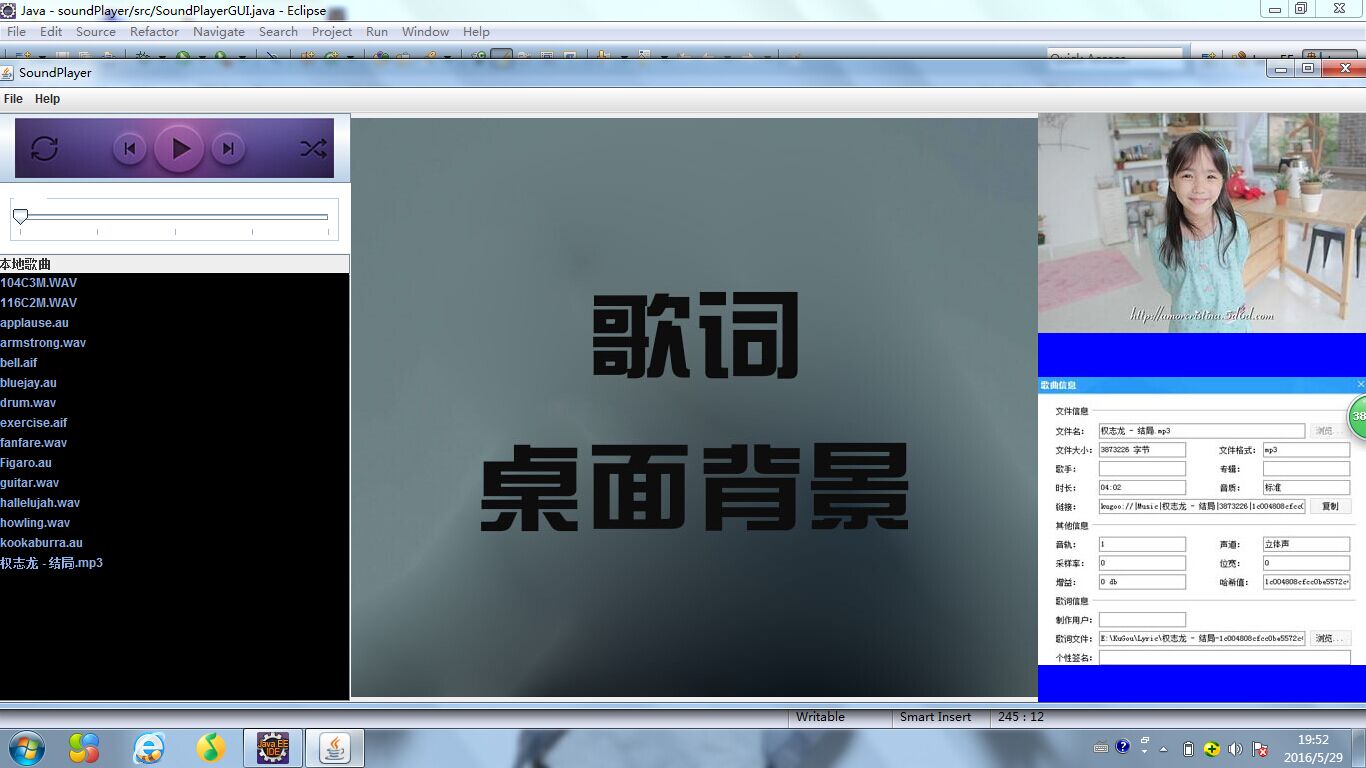
menu = new JMenu("Help");
menubar.add(menu);
item = new JMenuItem("About SoundPlayer...");
item.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) { showAbout(); }
});
menu.add(item);
}
}