ylbtech-SilverLight-DataControls-PagedCollectionView:The PagedCollectionView(分页的集合视图) 对象 |
- 1.A, Building a Data Object(创建一个数据对象)
- 1.B, Sorting(排序)
- 1.C, Filtering(过滤)
- 1.D, Grouping(分组)
- 1.E, Paging(分页)
1.A, Building a Data Object(创建一个数据对象)返回顶部 |
/Access/Product.cs

using System; using System.Collections.Generic; using System.Linq; using System.ComponentModel.DataAnnotations; namespace SLYlbtechApp.Access { /// <summary> /// 产品类 /// </summary> public class Product { int productId; /// <summary> /// 编号【PK】 /// </summary> public int ProductId { get { return productId; } set { productId = value; } } string productName; /// <summary> /// 产品名称 /// </summary> public string ProductName { get { return productName; } set { if (value=="") throw new ArgumentException("不能为空"); productName = value; } } string quantityPerUnit; /// <summary> /// 产品规格 /// </summary> public string QuantityPerUnit { get { return quantityPerUnit; } set { quantityPerUnit = value; } } /// <summary> /// 单位价格 /// 注意 不要使用 decimal 类型,在 UserControl.Resounrce 资源绑定是引发类型转换异常 /// </summary> double unitPrice; [Display(Name="价格",Description="价格不能小于0")] public double UnitPrice { get { return unitPrice; } set { if (value < 0) throw new ArgumentException("不能小于0"); unitPrice = value; } } string description; /// <summary> /// 描述 /// </summary> public string Description { get { return description; } set { description = value; } } string productImagePath; /// <summary> /// 产品图片地址 /// </summary> public string ProductImagePath { get { return productImagePath; } set { productImagePath = value; } } string categoryName; /// <summary> /// 类别名称 /// </summary> public string CategoryName { get { return categoryName; } set { categoryName = value; } } DateTime addedDate; /// <summary> /// 添加日期 /// </summary> public DateTime AddedDate { get { return addedDate; } set { addedDate = value; } } /// <summary> /// 空参构造 /// </summary> public Product() { AddedDate = new DateTime(2013, 11, 17); //设置默认日期 } /// <summary> /// 全参构造 /// </summary> /// <param name="productId"></param> /// <param name="productName"></param> /// <param name="quantityPerUnit"></param> /// <param name="unitPrice"></param> /// <param name="description"></param> /// <param name="productImagePath"></param> /// <param name="categoryName"></param> public Product(int productId, string productName, string quantityPerUnit, double unitPrice, string description , string productImagePath, string categoryName) { ProductId = productId; ProductName=productName; QuantityPerUnit=quantityPerUnit; UnitPrice=unitPrice; Description=description; ProductImagePath=productImagePath; CategoryName=categoryName; } /// <summary> /// 获取全部产品 /// </summary> /// <returns></returns> public static IList<Product> GetAll() { IList<Product> dals = new List<Product>(); string categoryName = string.Empty; string productImagePath = string.Empty; string description = "嗟夫!草木无情,有时飘零。人为动物,惟物之灵。百忧感其心,万事劳其形,有动于中,必摇其精。而况思其力之所不及,忧其智之所不能,宜其渥然丹者为槁木,黟然黑者为星星。奈何以非金石之质,欲与草木而争荣?念谁为之戕贼,亦何恨乎秋声!"; #region Add Product #region 饮料 categoryName = "饮料"; productImagePath = "../Images/pencil.jpg"; dals.Add(new Product() { ProductId = 100, ProductName = "啤酒", QuantityPerUnit = "1箱*6听", UnitPrice = 10d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); dals.Add(new Product() { ProductId = 120, ProductName = "绿茶", QuantityPerUnit = "500ml", UnitPrice = 3d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); dals.Add(new Product() { ProductId = 190, ProductName = "红茶", QuantityPerUnit = "500ml", UnitPrice = 3d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); dals.Add(new Product() { ProductId = 102, ProductName = "纯净水", QuantityPerUnit = "500ml", UnitPrice = 1.5d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); #endregion #region 零食 categoryName = "零食"; productImagePath = "../Images/pencil2.jpg"; dals.Add(new Product() { ProductId = 200, ProductName = "奥利奥巧克力饼干", QuantityPerUnit = "10 boxes x 20 bags", UnitPrice = 30d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); dals.Add(new Product() { ProductId = 220, ProductName = "玻璃 海苔", QuantityPerUnit = "20g", UnitPrice = 13d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); dals.Add(new Product() { ProductId = 210, ProductName = "好丽友 薯片", QuantityPerUnit = "100g", UnitPrice = 3d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); dals.Add(new Product() { ProductId = 201, ProductName = "统一 老坛酸菜面", QuantityPerUnit = "1 盒", UnitPrice = 8.5d, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }); #endregion #endregion return dals; } /// <summary> /// GetModel /// </summary> /// <returns></returns> public static Product GetModel() { Product dal = null; string categoryName = string.Empty; string productImagePath = string.Empty; string description = "嗟夫!草木无情,有时飘零。人为动物,惟物之灵。百忧感其心,万事劳其形,有动于中,必摇其精。而况思其力之所不及,忧其智之所不能,宜其渥然丹者为槁木,黟然黑者为星星。奈何以非金石之质,欲与草木而争荣?念谁为之戕贼,亦何恨乎秋声!"; categoryName = "饮料"; productImagePath = "Images/pencil.jpg"; dal = new Product() { ProductId = 100, ProductName = "啤酒", QuantityPerUnit = "1箱*6听", UnitPrice=3.5, CategoryName = categoryName , ProductImagePath = productImagePath, Description = description }; //dal._unitPrice = 10d; return dal; } /// <summary> /// GetModel /// </summary> /// <param name="id">产品编号</param> /// <returns></returns> public static Product GetModel(int id) { Product dal = null; IList<Product> dals = GetAll(); try { dal = dals.Single(p => p.ProductId == id); } catch { } finally { dals = null; } return dal; } } }
4,
1.B, Sorting(排序)返回顶部 |
1,
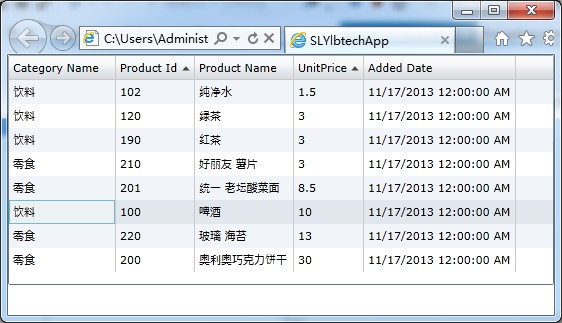
2,
2.1/3,
xmlns:data="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data"
2.2/3,

<Grid x:Name="LayoutRoot" Background="White"> <Grid.RowDefinitions> <RowDefinition Height="*"></RowDefinition> <RowDefinition Height="30"></RowDefinition> </Grid.RowDefinitions> <data:DataGrid Grid.Row="0" x:Name="gridList" AutoGenerateColumns="False"> <data:DataGrid.Columns> <data:DataGridTextColumn Header="Category Name" Binding="{Binding CategoryName}"></data:DataGridTextColumn> <data:DataGridTextColumn Header="Product Id" Binding="{Binding ProductId}"></data:DataGridTextColumn> <data:DataGridTextColumn Header="Product Name" Binding="{Binding ProductName}"></data:DataGridTextColumn> <data:DataGridTextColumn Header="UnitPrice" Binding="{Binding UnitPrice}" CanUserReorder="True"></data:DataGridTextColumn> <data:DataGridTextColumn Header="Added Date" Binding="{Binding AddedDate}"></data:DataGridTextColumn> </data:DataGrid.Columns> </data:DataGrid> </Grid>
2.3/3,
using System.Windows.Controls; using SLYlbtechApp.Access; using System.Windows.Data; namespace SLYlbtechApp.ThePagedCollectionView { public partial class Sorting : UserControl { public Sorting() { InitializeComponent(); PagedCollectionView view = new PagedCollectionView(Product.GetAll()); //排序 view.SortDescriptions.Add(new System.ComponentModel.SortDescription("UnitPrice" , System.ComponentModel.ListSortDirection.Ascending)); view.SortDescriptions.Add(new System.ComponentModel.SortDescription("ProductId" , System.ComponentModel.ListSortDirection.Ascending)); //二次排序 this.gridList.ItemsSource = view; } } }
3,
4,
1.C, Filtering(过滤)返回顶部 |
1,
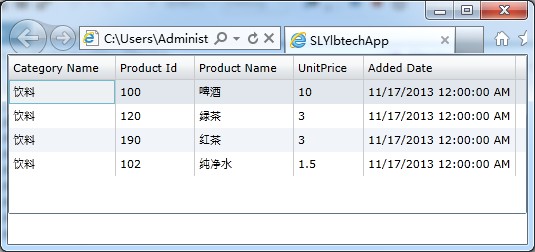
2,
2.1/3, 同上文 B.2.1/3
2.2/3, 同上文 B.2.2/3
2.3/3,
using System.Windows.Controls; using SLYlbtechApp.Access; using System.Windows.Data; namespace SLYlbtechApp.ThePagedCollectionView { public partial class Filtering : UserControl { public Filtering() { InitializeComponent(); PagedCollectionView view = new PagedCollectionView(Product.GetAll()); //过滤集合 view.Filter = delegate(object filterObject) { Product product = (Product)filterObject; return (product.CategoryName == "饮料"); //只显示 类别名称等于“饮料”的商品 }; this.gridList.ItemsSource = view; } } }
3,
4,
1.D Grouping(分组)返回顶部 |
1,
1.1/2, 一次分组
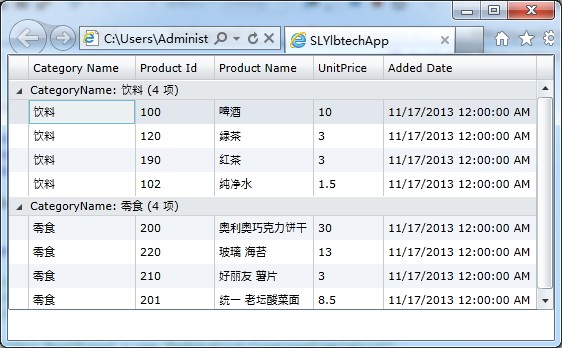
1.2/2,二次分组
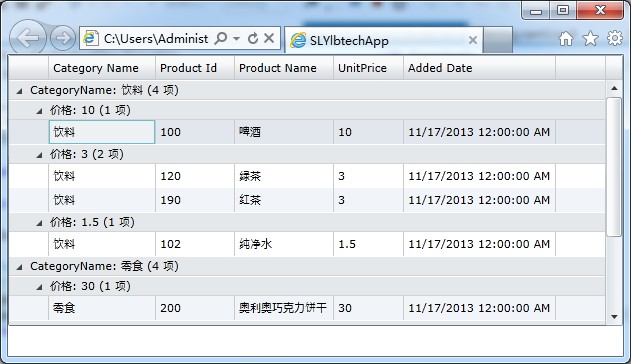
2,
2.1/3, 同上文 B.2.1/3
2.2/3, 同上文 B.2.2/3
2.3/3,
using System.Windows.Controls; using SLYlbtechApp.Access; using System.Windows.Data; namespace SLYlbtechApp.ThePagedCollectionView { public partial class Grouping : UserControl { public Grouping() { InitializeComponent(); PagedCollectionView paged = new PagedCollectionView(Product.GetAll()); //分组 paged.GroupDescriptions.Add(new PropertyGroupDescription("CategoryName")); //paged.GroupDescriptions.Add(new System.Windows.Data.PropertyGroupDescription("UnitPrice")); //二次分组 this.gridList.ItemsSource = paged; } } }
3,
4,
1.E, Paging(分页)返回顶部 |
1,
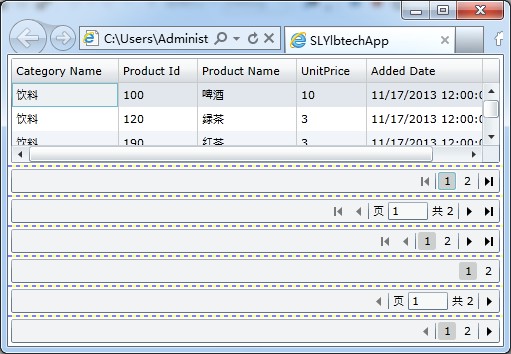
2,
2.1/3, 同上文 B.2.1/3
2.2/3,

<Grid x:Name="LayoutRoot" ShowGridLines="True" Background="White"> <Grid.RowDefinitions> <RowDefinition/> <RowDefinition Height="30"/> <RowDefinition Height="30"/> <RowDefinition Height="30"/> <RowDefinition Height="30"/> <RowDefinition Height="30"/> <RowDefinition Height="30"/> </Grid.RowDefinitions> <data:DataGrid x:Name="gridList" AutoGenerateColumns="False" Margin="3"> <data:DataGrid.Columns> <data:DataGridTextColumn Header="Category Name" Binding="{Binding CategoryName}"></data:DataGridTextColumn> <data:DataGridTextColumn Header="Product Id" Binding="{Binding ProductId}"></data:DataGridTextColumn> <data:DataGridTextColumn Header="Product Name" Binding="{Binding ProductName}"></data:DataGridTextColumn> <data:DataGridTextColumn Header="UnitPrice" Binding="{Binding UnitPrice}" CanUserReorder="True"></data:DataGridTextColumn> <data:DataGridTextColumn Header="Added Date" Binding="{Binding AddedDate}"></data:DataGridTextColumn> </data:DataGrid.Columns> </data:DataGrid> <data:DataPager x:Name="pager" Grid.Row="1" Margin="3" IsTotalItemCountFixed="True" PageSize="5" DisplayMode="FirstLastNumeric" Source="{Binding ItemsSource, ElementName=gridList}"/> <data:DataPager x:Name="pager2" Grid.Row="2" Margin="3" IsTotalItemCountFixed="True" PageSize="5" Source="{Binding ItemsSource, ElementName=gridList}"/> <data:DataPager x:Name="pager3" Grid.Row="3" Margin="3" IsTotalItemCountFixed="True" PageSize="5" DisplayMode="FirstLastPreviousNextNumeric" Source="{Binding ItemsSource, ElementName=gridList}"/> <data:DataPager x:Name="pager4" Grid.Row="4" Margin="3" IsTotalItemCountFixed="True" PageSize="5" DisplayMode="Numeric" Source="{Binding ItemsSource, ElementName=gridList}"/> <data:DataPager x:Name="pager5" Grid.Row="5" Margin="3" IsTotalItemCountFixed="True" PageSize="5" DisplayMode="PreviousNext" Source="{Binding ItemsSource, ElementName=gridList}"/> <data:DataPager x:Name="pager6" Grid.Row="6" Margin="3" IsTotalItemCountFixed="True" PageSize="5" DisplayMode="PreviousNextNumeric" Source="{Binding ItemsSource, ElementName=gridList}"/> </Grid>
2.3/3,
using System.Windows.Controls; using SLYlbtechApp.Access; using System.Windows.Data; namespace SLYlbtechApp.ThePagedCollectionView { public partial class Paging : UserControl { public Paging() { InitializeComponent(); //分页 PagedCollectionView view = new PagedCollectionView(Product.GetAll()); this.gridList.ItemsSource = view; } } }
3,
4,
1.F,返回顶部 |
![]() |
作者:ylbtech 出处:http://ylbtech.cnblogs.com/ 本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。 |