题目链接:http://poj.org/problem?id=3321
Apple Tree
Time Limit: 2000MS |
|
Memory Limit: 65536K |
Total Submissions: 34812 |
|
Accepted: 10469 |
Description
There is an apple tree outside of kaka's house. Every autumn, a lot of apples will grow in the tree. Kaka likes apple very much, so he has been carefully nurturing the big apple tree.
The tree has N forks which are connected by branches. Kaka numbers the forks by 1 to N and the root is always numbered by 1. Apples will grow on the forks and two apple won't grow on the same fork. kaka wants to know how many apples are there in a sub-tree, for his study of the produce ability of the apple tree.
The trouble is that a new apple may grow on an empty fork some time and kaka may pick an apple from the tree for his dessert. Can you help kaka?
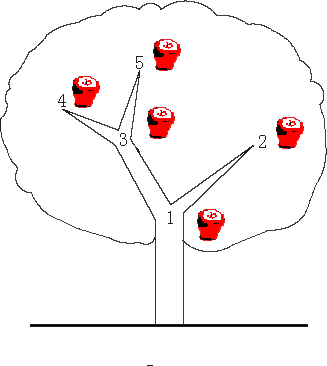
Input
The first line contains an integer N (N ≤ 100,000) , which is the number of the forks in the tree.
The following N - 1 lines each contain two integers u and v, which means fork u and fork v are connected by a branch.
The next line contains an integer M (M ≤ 100,000).
The following M lines each contain a message which is either
"C x" which means the existence of the apple on fork x has been changed. i.e. if there is an apple on the fork, then Kaka pick it; otherwise a new apple has grown on the empty fork.
or
"Q x" which means an inquiry for the number of apples in the sub-tree above the fork x, including the apple (if exists) on the fork x
Note the tree is full of apples at the beginning
Output
Sample Input
3 1 2 1 3 3 Q 1 C 2 Q 1
Sample Output
3 2
Source
题意概括:
给一个 N 阶无向图,要求把这个无向图转换为一颗以 1 为根的树后进行两种操作:
C x :x结点上如果有苹果就摘掉,如果没有苹果就长出一个新的来
Q x: 查询 x 结点与它的所有后代分支一共有几个苹果
解题思路:
因为原本苹果之间的关系我们不好处理,所以就给他们这些结点重新编号。
并且为了方便子树的苹果数求和(即区间查询)我们在给结点增加一个左值一个右值表示当前结点管辖的范围。
如何建树?
根据题目一开始给的边与边的关系我们可以通过 dfs 进行建树。
L[ x ] (X的左值): X结点的新编号就是该结点的左值,表示它管辖范围的下限
R[ x ] (X的右值):而子树的编号最大值为该结点的右值,表示它管辖范围的上限。
至于dfs顺序,如下
举个栗子:
N = 6;
1 - 2
1 - 6
2 - 5
2 - 3
6 - 4
到这里感觉有一点点线段树的味道了。。。
但这里要用树状数组对这些区间进行维护和查询。
例如:我要 Q 2;
由上面建好的新树我们可以知道 结点2这颗子树包含了结点2、结点5、结点3,而结点2所管辖的区间【2,4】刚好把它们包括进去了。
如果我们要求 这可子树的苹果数量,其实就是用树状数组求区间【2,4】的苹果总数
也就是右值的前缀和减去左值的前缀和(但不包括左值,因为结点本身也要算进去)即:ans = sum( R[ 2 ] ) - sum( L[ 2 ] - 1);
而C操作其实就是树状数组的单点更新而已。
注意事项:
一开始用 stl 的 vector 存无向图,虽然过了但效率不咋地。想想好久没用静态邻接表了,换了一下,快了好多。
AC code:
1 ///建树+树状数组(stl)8080k 1047ms 2 /* 3 #include <cstdio> 4 #include <iostream> 5 #include <cstring> 6 #include <vector> 7 #include <algorithm> 8 #define INF 0x3f3f3f3f 9 using namespace std; 10 typedef vector<int>ve; 11 12 const int MAXN = 1e5+10; 13 int t[MAXN], l[MAXN], r[MAXN]; 14 bool vis[MAXN]; 15 int N, M, cnt; 16 17 vector<ve>node(MAXN); 18 19 int lowbit(int x) 20 { 21 return x&(-x); 22 } 23 void add(int x, int value) 24 { 25 for(int i = x; i <= N; i+=lowbit(i)) 26 t[i]+=value; 27 } 28 int sum(int x) 29 { 30 int res = 0; 31 for(int i = x; i > 0; i-=lowbit(i)) 32 res+=t[i]; 33 return res; 34 } 35 void dfs(int k) 36 { 37 l[k] = cnt; 38 for(int i = 0; i < node[k].size(); i++) 39 { 40 cnt++; 41 dfs(node[k][i]); 42 } 43 r[k] = cnt; 44 return; 45 } 46 int main() 47 { 48 scanf("%d", &N); 49 int a, b; 50 for(int i = 1; i <= N; i++) 51 { 52 vis[i] = 1; 53 add(i, 1); 54 } 55 for(int i = 1; i < N; i++) 56 { 57 scanf("%d%d", &a, &b); 58 node[a].push_back(b); 59 } 60 cnt = 1; 61 dfs(1); 62 scanf("%d", &M); 63 while(M--) 64 { 65 char com[3]; 66 int px; 67 scanf("%s", &com); 68 if(com[0] == 'C') 69 { 70 scanf("%d", &px); 71 if(vis[px]) add(l[px], -1); 72 else add(l[px], 1); 73 vis[px] = !vis[px]; 74 } 75 else 76 { 77 scanf("%d", &px); 78 int ans = sum(r[px]) - sum(l[px]-1); 79 printf("%d ", ans); 80 } 81 } 82 return 0; 83 } 84 */ 85 86 ///建树+树状数组(静态连接表) 4940k 454ms 87 #include <cstdio> 88 #include <iostream> 89 #include <cstring> 90 #include <vector> 91 #include <algorithm> 92 #define INF 0x3f3f3f3f 93 using namespace std; 94 95 const int MAXN = 1e5+10; 96 const int MAXM = 1e5+10; 97 98 struct node 99 { 100 int to, next; 101 }edge[MAXM<<1]; 102 103 int head[MAXN]; 104 bool vis[MAXN]; 105 int t[MAXN], l[MAXN], r[MAXN]; 106 int N, M, cnt, key; 107 108 int lowbit(int x) 109 { 110 return x&(-x); 111 } 112 void add(int x, int value) 113 { 114 for(int i = x; i <= N; i+=lowbit(i)) 115 t[i]+=value; 116 } 117 int sum(int x) 118 { 119 int res = 0; 120 for(int i = x; i > 0; i-=lowbit(i)) 121 res+=t[i]; 122 return res; 123 } 124 125 void dfs(int k) 126 { 127 l[k] = key; 128 for(int i = head[k]; i != -1; i = edge[i].next) 129 { 130 if(l[edge[i].to]) continue; 131 key++; 132 dfs(edge[i].to); 133 } 134 r[k] = key; 135 } 136 137 void init() 138 { 139 memset(head, -1, sizeof(head)); 140 cnt = 1; 141 } 142 143 void add_e(int u, int v) 144 { 145 edge[cnt].to = v; 146 edge[cnt].next = head[u]; 147 head[u] = cnt++; 148 } 149 150 int main() 151 { 152 scanf("%d", &N); 153 init(); 154 int a, b; 155 for(int i = 1; i <= N; i++) 156 { 157 vis[i] = 1; //每个节点一开始都有苹果 158 add(i, 1); //初始化树状数组 159 } 160 for(int i = 1; i < N; i++) 161 { 162 scanf("%d%d", &a, &b); ///建无向图 163 add_e(a, b); 164 add_e(b, a); 165 } 166 key = 1; dfs(1); //建树 167 scanf("%d", &M); 168 int px; 169 char com[3]; 170 while(M--) 171 { 172 scanf("%s", &com); 173 if(com[0] == 'C') 174 { 175 scanf("%d", &px); 176 if(vis[px]) add(l[px], -1); 177 else add(l[px], 1); 178 vis[px] = !vis[px]; 179 } 180 else 181 { 182 scanf("%d", &px); 183 printf("%d ", sum(r[px])-sum(l[px]-1)); 184 } 185 } 186 return 0; 187 }