package Test5_4;
/**
3.(编程题)
要求使用线程同步与等待机制实现如下打印
*Thread-0#Thread-1@Thread-2
*Thread-0#Thread-1@Thread-2*Thread-0#Thread-1@Thread-2
*Thread-0#Thread-1@Thread-2*Thread-0#Thread-1@Thread-2*Thread-0#Thread-1@Thread-2
...
循环1000次
*/
class PrintThread{
private static int flag=0;
private int count=0;
public PrintThread(int count) {
this.count=count;
}
public void print() {
Object lock = new Object();
Thread aThread = new Thread(new Runnable() {
@Override
public void run() {
for(int i=0;i<count;) {
synchronized (lock) {
if(flag%3==0&&"Thread-0".equals(Thread.currentThread().getName())) {
System.out.print("*Thread-0");
flag++;
lock.notifyAll();
i++;
}
else {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
});
Thread bThread = new Thread(new Runnable() {
@Override
public void run() {
for(int i=0;i<count;) {
synchronized (lock) {
if(flag%3==1&&"Thread-1".equals(Thread.currentThread().getName())) {
System.out.print("#Thread-1");
flag++;
lock.notifyAll();
i++;
}
else {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
});
Thread cThread = new Thread(new Runnable() {
@Override
public void run() {
for(int i=0;i<count;) {
synchronized (lock) {
if(flag%3==2&&"Thread-2".equals(Thread.currentThread().getName())) {
System.out.print("@Thread-2
");
flag++;
lock.notifyAll();
i++;
}
else {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
});
aThread.start();
bThread.start();
cThread.start();
}
}
public class Test {
public static void main(String[] args) throws InterruptedException {
PrintThread mt = new PrintThread(3);
mt.print();
}
}
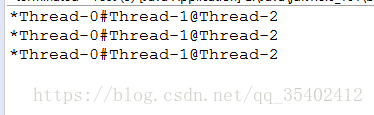
package Test5_4;
/**
题目:输入数组,最大的与第一个元素交换,最小的与最后一个元素交换,输出数组。
*/
public class Test2 {
public static void main(String[] args) {
int[] array= {0,1,2,3,4,5,6,7,8,9};
for (int i : array) {
System.out.print(i+" ");
}
System.out.println();
changeMaxMin(array);
for (int i : array) {
System.out.print(i+" ");
}
}
public static void swap(int[] array, int x,int y) {
int tmp = array[x];
array[x] = array[y];
array[y] = tmp;
}
public static void changeMaxMin(int[] array) {
int max=0, min=0;
for(int i=0;i<array.length;i++) {
if(array[max]<array[i]) {
max=i;
}
if(array[min]>array[i]) {
min=i;
}
}
swap(array, 0, max);
if(min==0) { //若min的下标就是0,则0的元素已被max交换,则min的值应变为max
min=max;
}
swap(array, array.length-1, min);
}
}