题目链接:http://poj.org/problem?id=2195
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions:27150 | Accepted: 13536 |
Description
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
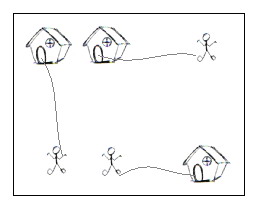
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates there is a little man on that point.
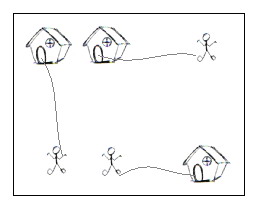
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
题目大意:人与房子的数量相等,人每走一步花费代价为1,求所有人都进入房子的总代价最小是多少。
思路:
1.可以用KM算法,也可以用最小费用最大流。这里用最大流,KM算法在这里:https://www.cnblogs.com/yuanweidao/p/11282994.html
2.设置一个超级源点0和超级汇点 2 * n + 1, 源点向人加容量为1,费用为0的边,房子向汇点加容量为1,费用用0的边(保证费用来自人到房子的代价)。每个人与每间房子都加容量为1,费用为该人到该房子需要移动的距离与人走路代价的乘积。
(容量都为1保证跑MCMF时每条边都跑到,即每个人都到房子里去了。)

1 #include<stdio.h> 2 #include<string.h> 3 #include<queue> 4 #include<math.h> 5 #include<algorithm> 6 #define mem(a, b) memset(a, b, sizeof(a)) 7 using namespace std; 8 const int MAXN = 110 + 10; 9 const int inf = 0x3f3f3f3f; 10 11 char map[MAXN][MAXN]; 12 int cnt1/*人的序号*/, cnt2/*房子的序号*/; 13 int mincost, last[2 * MAXN], pre[2 * MAXN], dis[2 * MAXN], flow[2 * MAXN], vis[2 * MAXN]; 14 queue<int> Q; 15 16 struct Node 17 { 18 int x, y; 19 }no[2 * MAXN]; 20 21 struct Edge 22 { 23 int to, next, flow, cost; 24 }edge[2 * MAXN * MAXN]; 25 int head[2 * MAXN], e_num; 26 void add(int a, int b, int c, int d) 27 { 28 edge[++ e_num].to = b; 29 edge[e_num].next = head[a]; 30 head[a] = e_num; 31 edge[e_num].flow = c; 32 edge[e_num].cost = d; 33 } 34 35 void init() 36 { 37 mincost = 0; 38 cnt1 = 0; 39 e_num = -1; 40 mem(head, -1); 41 } 42 43 bool spfa(int st, int ed) 44 { 45 mem(dis, inf), mem(flow, inf), mem(vis, 0); 46 pre[ed] = -1; 47 dis[st] = 0; 48 vis[st] = 1; 49 Q.push(st); 50 while(!Q.empty()) 51 { 52 int now = Q.front(); 53 Q.pop(); 54 vis[now] = 0; 55 for(int i = head[now]; i != -1; i = edge[i].next) 56 { 57 int to = edge[i].to; 58 if(edge[i].flow > 0 && dis[to] > dis[now] + edge[i].cost) 59 { 60 dis[to] = dis[now] + edge[i].cost; 61 pre[to] = now; 62 last[to] = i; 63 flow[to] = min(flow[now], edge[i].flow); 64 if(!vis[to]) 65 { 66 vis[to] = 1; 67 Q.push(to); 68 } 69 } 70 } 71 } 72 return pre[ed] != -1; 73 } 74 75 void MCMF() 76 { 77 while(spfa(0, cnt2 + 1)) 78 { 79 int ed = cnt2 + 1; 80 int now = ed; 81 // maxflow += flow[ed]; 82 mincost += flow[ed] * dis[ed]; 83 while(now != 0) 84 { 85 edge[last[now]].flow -= flow[ed]; 86 edge[last[now] ^ 1].flow += flow[ed]; 87 now = pre[now]; 88 } 89 } 90 } 91 92 int main() 93 { 94 int n, m; 95 while(scanf("%d%d", &n, &m) != EOF) 96 { 97 getchar(); 98 init(); 99 if(n == 0 && m == 0) 100 break; 101 for(int i = 1; i <= n; i ++) 102 scanf("%s", map[i] + 1); 103 for(int i = 1; i <= n; i ++) 104 for(int j = 1; j <= m; j ++) 105 if(map[i][j] == 'm') 106 no[++ cnt1].x = i, no[cnt1].y = j; //人的编号和坐标 107 cnt2 = cnt1; 108 for(int i = 1; i <= n; i ++) 109 for(int j = 1; j <= m; j ++) 110 if(map[i][j] == 'H') 111 no[++ cnt2].x = i, no[cnt2].y = j;//房子编号和坐标 112 for(int i = 1; i <= cnt1; i ++) //源点 0 到每个人加边 容量为1 花费为0 113 { 114 add(0, i, 1, 0); 115 add(i, 0, 0, 0); 116 } 117 for(int i = cnt1 + 1; i <= cnt2; i ++)//房子到 汇点 cnt2 + 1 加边 容量为1 花费为0 118 { 119 add(i, cnt2 + 1, 1, 0); 120 add(cnt2 + 1, i, 0, 0); 121 } 122 for(int i = 1; i <= cnt1; i ++) 123 { 124 for(int j = cnt1 + 1; j <= cnt2; j ++) 125 { 126 int a, b, c, d; 127 a = i, b = j, c = 1; 128 d = abs(no[i].x - no[j].x) + abs(no[i].y - no[j].y);//花费为两点之间的哈密顿距离 129 add(a, b, c, d); 130 add(b, a, 0, -d); 131 } 132 } 133 MCMF(); 134 printf("%d ", mincost); 135 } 136 return 0; 137 }