前情提要:
上一章节我们说到的是对页面元素的excel读取以及封装,讲到了操作excel操作,本章节将告诉读者如何让脚本自动执行属于不同系统的浏览器驱动,封装配置文件库,方便数据读取
首先我们要了解一下ini文件:
使用配置文件来灵活的配置一些参数是一件很常见的事情,配置文件的解析并不复杂,在python里更是如此,在官方发布的库中就包含有做这件事情的库,那就是configParser
configParser解析的配置文件的格式比较象ini的配置文件格式,就是文件中由多个section构成,每个section下又有多个配置项
ini文件格式
1 2 [section0] 3 4 key0 = value0 5 key1 = value1 6 7 [section1] 8 9 key2 = value2 10 key3 = value3
注意: section不能重复,里面数据通过section去查找,每个seletion下可以有多个key和vlaue的键值对,注释用英文分号(;)
- 如何读取ini文件
python3里面自带configparser模块来读取ini文件
设置配置文件
1 # 配置文件处理 2 [DEFAULT] 3 # 测试网站主页面 4 URL = http://shop.aircheng.com/ 5 # 默认等待时间 6 TIME_OUT = 5
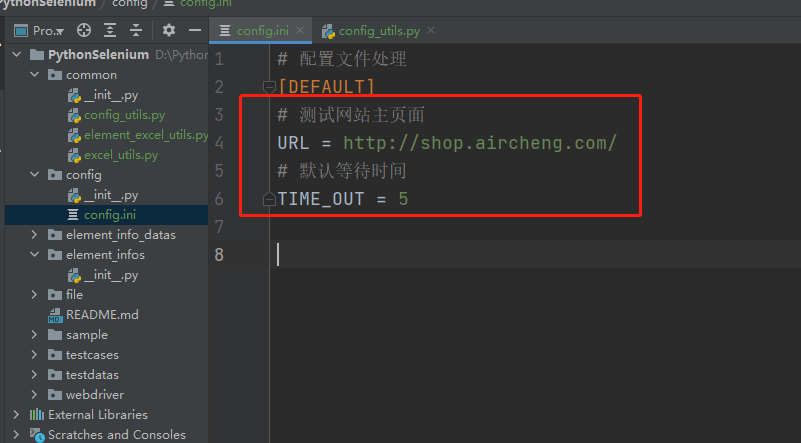
- 新建config_utils.py文件
# -*- coding: utf-8 -*- # @Time : 2022/1/4 19:34 # @Author : Limusen # @File : config_utils import os import configparser current_path = os.path.dirname(os.path.abspath(__file__)) conf_path = os.path.join(current_path, '..', 'config', 'config.ini') class ConfigUtils: def __init__(self): self.cfg = configparser.ConfigParser() self.cfg.read(conf_path, encoding="utf-8") @property def get_host(self): return self.cfg.get("DEFAULT", "url") @property def time_out(self): return self.cfg.get("DEFAULT","time_out") local_config = ConfigUtils() print(local_config.time_out) print(local_config.get_host)
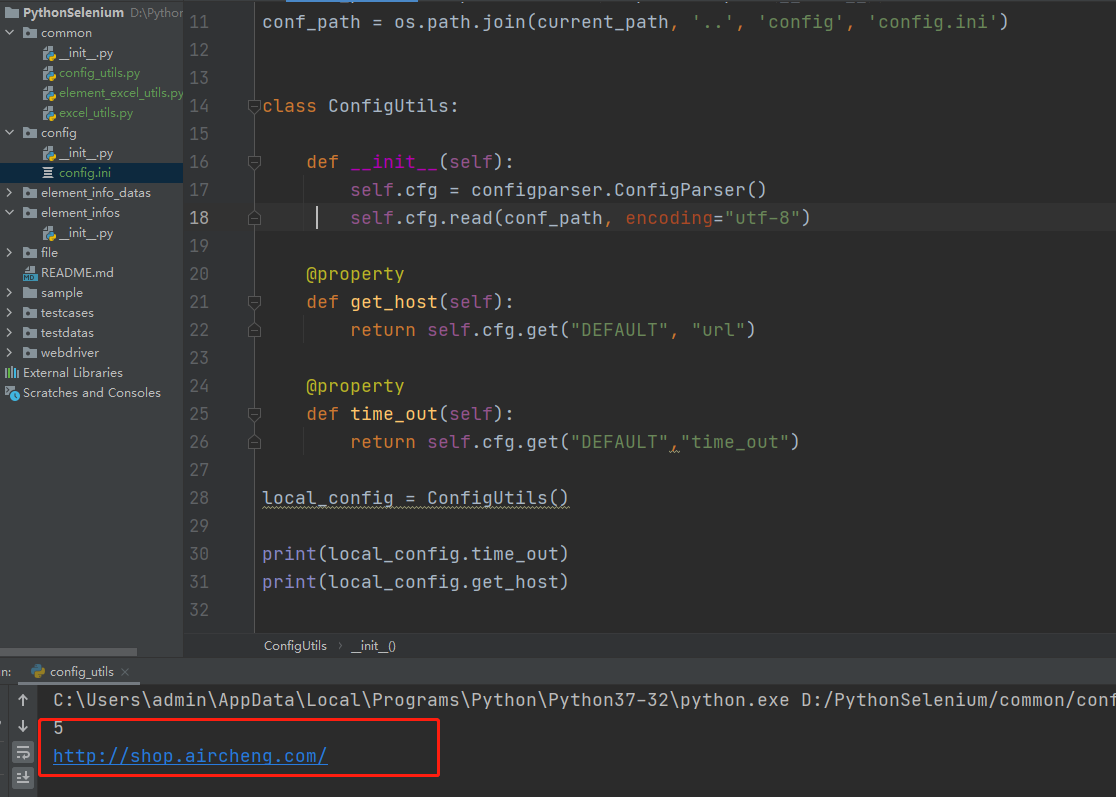
- 优化代码 优化element_excel_utils.py中的超时时间采用配置文件中的5
- 封装驱动类
这里我们需要用到os模块跟系统模块sys
os模块需要获取我们存放驱动的路径. sys模块主要是为了让脚本区分当前是在windows还是mac环境
- demo_browser_22.py
1 # -*- coding: utf-8 -*- 2 # @Time : 2022/1/4 19:46 3 # @Author : Limusen 4 # @File : demo_browser_22 5 6 import os 7 from selenium import webdriver 8 # 引入系统类 9 import sys 10 11 # 获取项目顶层路径 12 current_path = os.path.abspath(os.path.dirname(os.path.dirname(os.path.dirname(__file__)))) 13 # print(current_path) 14 15 # 谷歌驱动地址 16 chrome_path = os.path.join(current_path, 'webdriver', 'chrome', 'chromedriver93.exe') 17 # print(chrome_path) 18 19 # 火狐驱动地址 20 firefox_path = os.path.join(current_path, 'webdriver', 'firefox', 'geckodriver.exe') 21 # print(firefox_path) 22 23 # edge驱动地址 24 edge_path = os.path.join(current_path, 'webdriver', 'edge', 'msedgedriver.exe') 25 # print(edge_path) 26 27 # driver = webdriver.Chrome(executable_path=chrome_path) 28 # driver.get("https://www.baidu.com") 29 # 30 # driver = webdriver.Firefox(executable_path=firefox_path) 31 # driver.get("https://www.baidu.com") 32 # 33 # driver = webdriver.Edge(executable_path=edge_path) 34 # driver.get("https://www.baidu.com") 35 36 # 可以判断当前是mac还是windows 37 system_driver = sys.platform 38 39 if system_driver.lower() == "darwin": 40 print("当前是mac环境") 41 else: 42 print("当前是windows环境")
- 新建browser_utils.py
先封装简易代码
# -*- coding: utf-8 -*- # @Time : 2022/1/4 19:44 # @Author : Limusen # @File : browser_utils # 封装驱动类 import os import sys from selenium import webdriver from common.config_utils import local_config current_path = os.path.dirname(os.path.abspath(__file__)) system_driver = sys.platform class BrowserUtils: def __init__(self): self.driver_name = local_config.driver_name def get_chrome_driver(self): # 先封装简易代码 chrome_path = os.path.join(current_path, '..', 'webdriver', 'chrome', 'chromedriver93.exe') driver = webdriver.Chrome(executable_path=chrome_path) return driver def get_firefox_driver(self): firefox_path = os.path.join(current_path, '..', 'webdriver', 'firefox', 'geckodriver.exe') driver = webdriver.Firefox(executable_path=firefox_path) return driver def get_edge_driver(self): edge_path = os.path.join(current_path, '..', 'webdriver', 'edge', 'msedgedriver.exe') driver = webdriver.Edge(executable_path=edge_path) return driver if __name__ == '__main__': driver = BrowserUtils().get_edge_driver() driver.get("https://www.baidu.com")
继续封装,通过一个方法调用不同的驱动,做成私有化
在config.ini文件当中新增驱动chrome
继续优化代码
# -*- coding: utf-8 -*- # @Time : 2022/1/4 19:44 # @Author : Limusen # @File : browser_utils # 封装驱动类 import os import sys from selenium import webdriver from common.config_utils import local_config current_path = os.path.dirname(os.path.abspath(__file__)) system_driver = sys.platform class BrowserUtils: def __init__(self): self.driver_name = local_config.driver_name def get_driver(self): if self.driver_name.lower() == 'chrome': return self.__get_chrome_driver() elif self.driver_name.lower() == 'firefox': return self.__get_firefox_driver() elif self.driver_name.lower() == 'edge': return self.__get_edge_driver() def __get_chrome_driver(self): # 先封装简易代码 chrome_path = os.path.join(current_path, '..', 'webdriver', 'chrome', 'chromedriver93.exe') driver = webdriver.Chrome(executable_path=chrome_path) return driver def __get_firefox_driver(self): firefox_path = os.path.join(current_path, '..', 'webdriver', 'firefox', 'geckodriver.exe') driver = webdriver.Firefox(executable_path=firefox_path) return driver def __get_edge_driver(self): edge_path = os.path.join(current_path, '..', 'webdriver', 'edge', 'msedgedriver.exe') driver = webdriver.Edge(executable_path=edge_path) return driver if __name__ == '__main__': driver = BrowserUtils().get_driver() driver.get("https://www.baidu.com")
加入系统判断
# -*- coding: utf-8 -*- # @Time : 2022/1/4 19:44 # @Author : Limusen # @File : browser_utils # 封装驱动类 import os import sys from selenium import webdriver from common.config_utils import local_config current_path = os.path.dirname(os.path.abspath(__file__)) system_driver = sys.platform class BrowserUtils: def __init__(self): self.driver_name = local_config.driver_name def get_driver(self): if self.driver_name.lower() == 'chrome': return self.__get_chrome_driver() elif self.driver_name.lower() == 'firefox': return self.__get_firefox_driver() elif self.driver_name.lower() == 'edge': return self.__get_edge_driver() def __get_chrome_driver(self): # 先封装简易代码 # 加入系统环境判断 if system_driver.lower() == "darwin": """如果是mac系统执行这个驱动""" chrome_path = os.path.join(current_path, '..', 'webdriver', 'chrome', 'chromedriver.exe') driver = webdriver.Chrome(executable_path=chrome_path) return driver else: chrome_path = os.path.join(current_path, '..', 'webdriver', 'chrome', 'chromedriver93.exe') driver = webdriver.Chrome(executable_path=chrome_path) return driver def __get_firefox_driver(self): if system_driver.lower() == "darwin": firefox_path = os.path.join(current_path, '..', 'webdriver', 'firefox', 'geckodriver') driver = webdriver.Firefox(executable_path=firefox_path) return driver else: firefox_path = os.path.join(current_path, '..', 'webdriver', 'firefox', 'geckodriver.exe') driver = webdriver.Firefox(executable_path=firefox_path) return driver def __get_edge_driver(self): """ 驱动下载地址:https://developer.microsoft.com/zh-cn/microsoft-edge/tools/webdriver/ :return: """ edge_path = os.path.join(current_path, '..', 'webdriver', 'edge', 'msedgedriver.exe') driver = webdriver.Edge(executable_path=edge_path) return driver if __name__ == '__main__': driver = BrowserUtils().get_driver() driver.get("https://www.baidu.com")
去除浏览器提示白条
# -*- coding: utf-8 -*- # @Time : 2022/1/4 19:44 # @Author : Limusen # @File : browser_utils # 封装驱动类 import os import sys import warnings from selenium import webdriver from common.config_utils import local_config from selenium.webdriver.chrome.options import Options current_path = os.path.dirname(os.path.abspath(__file__)) system_driver = sys.platform class BrowserUtils: def __init__(self): # 去除控制台警告 warnings.filterwarnings("ignore", category=DeprecationWarning) self.driver_name = local_config.driver_name def get_driver(self): if self.driver_name.lower() == 'chrome': return self.__get_chrome_driver() elif self.driver_name.lower() == 'firefox': return self.__get_firefox_driver() elif self.driver_name.lower() == 'edge': return self.__get_edge_driver() def __get_chrome_driver(self): # 先封装简易代码 # 加入系统环境判断 chrome_options = Options() chrome_options.add_argument('--disable-gpu') # 谷歌文档提到需要加上这个属性来规避bug chrome_options.add_argument('lang=zh_CN.UTF-8') # 设置默认编码为utf-8 chrome_options.add_experimental_option('useAutomationExtension', False) # 取消chrome受自动控制提示 chrome_options.add_experimental_option("excludeSwitches", ['enable-automation']) # 取消chrome受自动控制提示 if system_driver.lower() == "darwin": """如果是mac系统执行这个驱动""" chrome_path = os.path.join(current_path, '..', 'webdriver', 'chrome', 'chromedriver.exe') driver = webdriver.Chrome(executable_path=chrome_path, options=chrome_options) return driver else: chrome_path = os.path.join(current_path, '..', 'webdriver', 'chrome', 'chromedriver93.exe') driver = webdriver.Chrome(executable_path=chrome_path, options=chrome_options) return driver def __get_firefox_driver(self): if system_driver.lower() == "darwin": firefox_path = os.path.join(current_path, '..', 'webdriver', 'firefox', 'geckodriver') driver = webdriver.Firefox(executable_path=firefox_path) return driver else: firefox_path = os.path.join(current_path, '..', 'webdriver', 'firefox', 'geckodriver.exe') driver = webdriver.Firefox(executable_path=firefox_path) return driver def __get_edge_driver(self): """ 驱动下载地址:https://developer.microsoft.com/zh-cn/microsoft-edge/tools/webdriver/ :return: """ edge_path = os.path.join(current_path, '..', 'webdriver', 'edge', 'msedgedriver.exe') driver = webdriver.Edge(executable_path=edge_path) return driver if __name__ == '__main__': driver = BrowserUtils().get_driver() driver.get("https://www.baidu.com")
到此 封装驱动类就结束了 小伙伴可以在不同的系统尝试一下
下一章节内容敬请期待