Crashing Robots
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 11432 | Accepted: 4831 |
Description
In a modernized warehouse, robots are used to fetch the goods. Careful planning is needed to ensure that the robots reach their destinations without crashing into each other. Of course, all warehouses are rectangular, and all robots occupy a circular floor space with a diameter of 1 meter. Assume there are N robots, numbered from 1 through N. You will get to know the position and orientation of each robot, and all the instructions, which are carefully (and mindlessly) followed by the robots. Instructions are processed in the order they come. No two robots move simultaneously; a robot always completes its move before the next one starts moving.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
Input
The first line of input is K, the number of test cases. Each test case starts with one line consisting of two integers, 1 <= A, B <= 100, giving the size of the warehouse in meters. A is the length in the EW-direction, and B in the NS-direction.
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
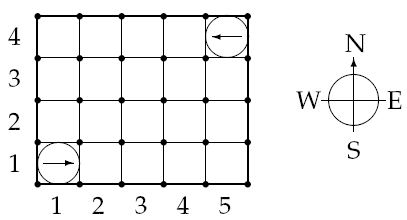
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
- L: turn left 90 degrees,
- R: turn right 90 degrees, or
- F: move forward one meter,
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
Output
Output one line for each test case:
Only the first crash is to be reported.
- Robot i crashes into the wall, if robot i crashes into a wall. (A robot crashes into a wall if Xi = 0, Xi = A + 1, Yi = 0 or Yi = B + 1.)
- Robot i crashes into robot j, if robots i and j crash, and i is the moving robot.
- OK, if no crashing occurs.
Only the first crash is to be reported.
Sample Input
4
5 4
2 2
1 1 E
5 4 W
1 F 7
2 F 7
5 4
2 4
1 1 E
5 4 W
1 F 3
2 F 1
1 L 1
1 F 3
5 4
2 2
1 1 E
5 4 W
1 L 96
1 F 2
5 4
2 3
1 1 E
5 4 W
1 F 4
1 L 1
1 F 20
Sample Output
Robot 1 crashes into the wall
Robot 1 crashes into robot 2
OK
Robot 1 crashes into robot 2
Source
题目大意:
给定一个A*B的棋盘,N个机器人,每个机器人都有起始位置,M个指令(x,C,r)代表第x个机器人执行指令C重复r次。
F->向前走一步
L->向左转
R->向右转
若i号机器人撞墙,输出:Robot i crashes into the wall
若i号机器人撞到j号机器人,输出:Robot i crashes into robot j
若M个指令执行完仍无事故发生 输出:OK
解题思路:
模拟题,看不懂英文是硬伤(┬_┬)
1 #include<iostream> 2 #include<cstdio> 3 #include<map> 4 using namespace std; 5 int OK = 1; 6 struct robot{ 7 int x; 8 int y; 9 char dir; 10 }rb[102]; 11 int m[5][2] = {{1,0},//东 12 {0,-1},//南 13 {-1,0},//西 14 {0,1},//北 15 {0,0}}; 16 bool Move(int rID,char Direction,int repeat,int N,int A,int B) 17 { 18 19 for(int i = 0;i<repeat;i++) 20 { 21 int mi; 22 if(rb[rID].dir == 'E') mi = 0; 23 else if(rb[rID].dir == 'S') mi = 1; 24 else if(rb[rID].dir == 'W') mi = 2; 25 else if(rb[rID].dir == 'N') mi = 3; 26 27 ///变换方向 28 if(Direction == 'L') 29 { 30 mi = (mi+4-1)%4; 31 if(mi == 0) rb[rID].dir = 'E'; 32 else if(mi == 1) rb[rID].dir = 'S'; 33 else if(mi == 2) rb[rID].dir = 'W'; 34 else if(mi == 3) rb[rID].dir = 'N'; 35 mi = 4;} 36 else if(Direction == 'R') { 37 mi = (mi+1)%4; 38 if(mi == 0) rb[rID].dir = 'E'; 39 else if(mi == 1) rb[rID].dir = 'S'; 40 else if(mi == 2) rb[rID].dir = 'W'; 41 else if(mi == 3) rb[rID].dir = 'N'; 42 mi = 4; 43 } 44 if(mi != 4) 45 { 46 ///判断是否和墙相撞 47 if(((rb[rID].x-1)%A==0&&m[mi][0]==-1) 48 ||((rb[rID].x-1)%A==(A-1)&&m[mi][0]==1) 49 ||((rb[rID].y-1)%B==0&&m[mi][1]==-1) 50 ||((rb[rID].y-1)%B==(B-1)&&m[mi][1]==1)) 51 { 52 cout<<"Robot "<<rID<<" crashes into the wall"<<endl; 53 return 0; 54 } 55 56 ///进行移动 57 rb[rID].x += m[mi][0]; 58 rb[rID].y += m[mi][1]; 59 60 for(int j = 1;j<=N;j++) 61 {///判断是否和机器人相撞 62 if(j != rID) 63 { 64 if(rb[j].x == rb[rID].x && rb[j].y == rb[rID].y) 65 { 66 cout<<"Robot "<<rID<<" crashes into robot "<<j<<endl; 67 return 0; 68 } 69 } 70 } 71 } 72 73 } 74 return 1; 75 } 76 int main() 77 { 78 int turn; 79 cin>>turn; 80 while(turn--) 81 { 82 OK = 1; 83 int A,B; 84 cin>>A>>B; 85 int N,M; 86 cin>>N>>M; 87 for(int i=1;i<=N;i++) 88 { 89 cin>>rb[i].x>>rb[i].y; 90 while((rb[i].dir = getchar()) == ' '); 91 } 92 93 while(M--) 94 { 95 char Direction; 96 int rID,repeat; 97 cin>>rID; 98 while((Direction = getchar()) == ' '); 99 cin>>repeat; 100 if(OK == 1) 101 { 102 if(!Move(rID,Direction,repeat,N,A,B)) 103 OK = 0; 104 } 105 } 106 if(OK == 1) cout<<"OK"<<endl; 107 108 } 109 return 0; 110 }