Pipe
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 11421 | Accepted: 3567 |
Description
The GX Light Pipeline Company started to prepare bent pipes for the new transgalactic light pipeline. During the design phase of the new pipe shape the company ran into the problem of determining how far the light can reach inside each component of the pipe. Note that the material which the pipe is made from is not transparent and not light reflecting.
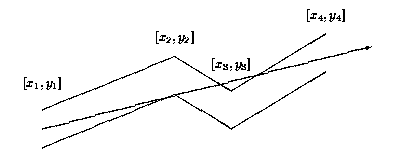
Each pipe component consists of many straight pipes connected tightly together. For the programming purposes, the company developed the description of each component as a sequence of points [x1; y1], [x2; y2], . . ., [xn; yn], where x1 < x2 < . . . xn . These are the upper points of the pipe contour. The bottom points of the pipe contour consist of points with y-coordinate decreased by 1. To each upper point [xi; yi] there is a corresponding bottom point [xi; (yi)-1] (see picture above). The company wants to find, for each pipe component, the point with maximal x-coordinate that the light will reach. The light is emitted by a segment source with endpoints [x1; (y1)-1] and [x1; y1] (endpoints are emitting light too). Assume that the light is not bent at the pipe bent points and the bent points do not stop the light beam.
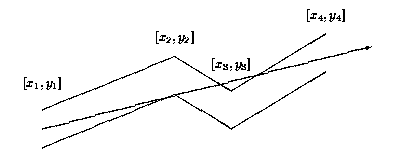
Each pipe component consists of many straight pipes connected tightly together. For the programming purposes, the company developed the description of each component as a sequence of points [x1; y1], [x2; y2], . . ., [xn; yn], where x1 < x2 < . . . xn . These are the upper points of the pipe contour. The bottom points of the pipe contour consist of points with y-coordinate decreased by 1. To each upper point [xi; yi] there is a corresponding bottom point [xi; (yi)-1] (see picture above). The company wants to find, for each pipe component, the point with maximal x-coordinate that the light will reach. The light is emitted by a segment source with endpoints [x1; (y1)-1] and [x1; y1] (endpoints are emitting light too). Assume that the light is not bent at the pipe bent points and the bent points do not stop the light beam.
Input
The input file contains several blocks each describing one pipe component. Each block starts with the number of bent points 2 <= n <= 20 on separate line. Each of the next n lines contains a pair of real values xi, yi separated by space. The last block is denoted with n = 0.
Output
The output file contains lines corresponding to blocks in input file. To each block in the input file there is one line in the output file. Each such line contains either a real value, written with precision of two decimal places, or the message Through all the pipe.. The real value is the desired maximal x-coordinate of the point where the light can reach from the source for corresponding pipe component. If this value equals to xn, then the message Through all the pipe. will appear in the output file.
Sample Input
4 0 1 2 2 4 1 6 4 6 0 1 2 -0.6 5 -4.45 7 -5.57 12 -10.8 17 -16.55 0
Sample Output
4.67 Through all the pipe.
Source
大致题意:在上下高度差为1的管道,射一束光,不能反射,求能传播到的最远距离.
分析:很容易想到这条直线一定要经过一个最低点和一个最高点,这样才能最远.那么枚举最低点和最高点,首先判断是否在入口的范围内,然后逐个逐个管道判断.需要判断直线是和上管道相交还是和下管道相交,根据斜率大小就可以得到.
#include <cstdio> #include <cmath> #include <cstring> #include <iostream> #include <algorithm> using namespace std; const double eps = 1e-8; int n; double pos[30][2],k,b,ans; int cmp(double a) //三出口函数 { if (fabs(a) < eps) return 0; else if (a > eps) return 1; return -1; } double jiao(double x3,double y3,double x4,double y4) //求直线与管道的交点 { double k2 = (y4 - y3) / (x4 - x3); double b2 = y4 - k2 * x4; return (b2 - b) / (k - k2); } double solve(double x3,double y3,double x4,double y4) { double res = pos[n][0]; k = (y4 - y3) / (x4 - x3); b = y4 - k * x4; double temp = k * pos[1][0] + b; if (cmp(temp - pos[1][1]) == 1 || cmp(temp - pos[1][1] + 1) == -1) return pos[1][0]; for (int i = 2; i <= n; i++) { double h = pos[i][0] * k + b; if (cmp(h - pos[i][1] + 1) >= 0 && cmp(h - pos[i][1]) <= 0) //可以通过当前管道 continue; double tk = (pos[i][1] - pos[i-1][1]) / (pos[i][0] - pos[i - 1][0]),tb; if (cmp(k - tk) == 1) //上 当前直线斜率大于管道斜率,则与上边相交 { double temp = jiao(pos[i-1][0],pos[i-1][1],pos[i][0],pos[i][1]); return temp; } else //下 { double temp = jiao(pos[i-1][0],pos[i-1][1] - 1,pos[i][0],pos[i][1] - 1); return temp; } } return res; } int main() { while (scanf("%d",&n) != EOF && n) { for (int i = 1; i <= n; i++) scanf("%lf%lf",&pos[i][0],&pos[i][1]); if (n == 2) { puts("Through all the pipe."); continue; } ans = pos[1][0]; for (int i = 1; i <= n; i++) for (int j = i + 1; j <= n; j++) { double temp = solve(pos[i][0],pos[i][1],pos[j][0],pos[j][1] - 1); if (cmp(temp - ans) == 1) ans = temp; temp = solve(pos[i][0],pos[i][1] - 1,pos[j][0],pos[j][1]); if (cmp(temp - ans) == 1) ans = temp; } if (cmp(ans - pos[n][0]) == 0) puts("Through all the pipe."); else printf("%.2lf ",ans); } return 0; }