Description
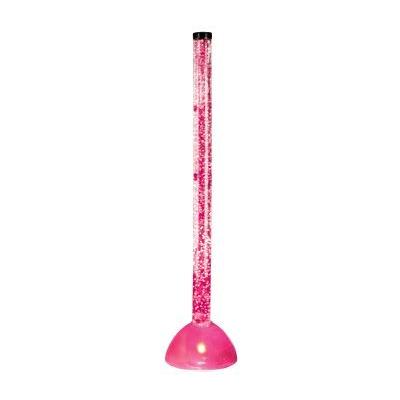
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
大意:求冒泡排序交换的个数,也就是求逆序数,套一下模板

#include<cstring> #include<cstdio> #include<algorithm> const int MAX = 510000; int a[MAX],temp[MAX]; int ans = 0; void mergesort(int *a,int first,int mid,int last,int *temp) { int i = first,j = mid + 1; int k = first; while( i <= mid && j <= last){ if(a[i] <= a[j]) temp[k++] = a[i++]; else { ans += j - k; temp[k++] = a[j++]; } } while( i <= mid) temp[k++] = a[i++]; while( j <= last) temp[k++] = a[j++]; for(int i = first;i <= last;i++) a[i] = temp[i]; } void mergearray(int *a,int first,int last,int *temp) { if(first < last){ int mid = (first + last) >> 1; mergearray(a,first,mid,temp); mergearray(a,mid+1,last,temp); mergesort(a,first,mid,last,temp); } } int main() { int n; scanf("%d",&n); for(int i = 1; i <= n; i++) scanf("%d",&a[i]); mergearray(a,1,n,temp); printf("%d",ans); return 0; }
树状数组:
/************************************************ * Author :Powatr * Created Time :2015-8-13 19:02:01 * File Name :poj2299Ultra-QuickSort.cpp ************************************************/ #include <cstdio> #include <algorithm> #include <iostream> #include <sstream> #include <cstring> #include <cmath> #include <string> #include <vector> #include <queue> #include <deque> #include <stack> #include <list> #include <map> #include <set> #include <bitset> #include <cstdlib> #include <ctime> using namespace std; #define lson l, mid, rt << 1 #define rson mid + 1, r, rt << 1 | 1 typedef long long ll; const int MAXN = 5e5 + 10; const int INF = 0x3f3f3f3f; const int MOD = 1e9 + 7; struct edge{ long long m; int id; bool operator <(const edge &a) const{ return m < a.m; } }a[MAXN]; int C[MAXN]; int b[MAXN]; int query(int x) { int ret = 0; while(x > 0){ ret += C[x]; x -= x&-x; } return ret; } void update(int x) { while(x < MAXN){ C[x]++; x += x&-x; } } int main(){ int n; int m; while(~scanf("%d", &n)&&n){ int cnt = 0; for(int i = 1; i <= n; i++){ scanf("%I64d", &a[i].m); a[i].id = i; } sort(a+1, a+n+1); for(int i = 1; i <= n; i++) b[a[i].id] = i; ll ans = 0; memset(C, 0, sizeof(C)); for(int i = 1; i <= n; i++){ ans += i - query(b[i]) - 1; update(b[i]); } printf("%I64d ", ans); } return 0; }