A. Search for Pretty Integers
You are given two lists of non-zero digits.
Let's call an integer pretty if its (base 10) representation has at least one digit from the first list and at least one digit from the second list. What is the smallest positive pretty integer?
The first line contains two integers n and m (1 ≤ n, m ≤ 9) — the lengths of the first and the second lists, respectively.
The second line contains n distinct digits a1, a2, ..., an (1 ≤ ai ≤ 9) — the elements of the first list.
The third line contains m distinct digits b1, b2, ..., bm (1 ≤ bi ≤ 9) — the elements of the second list.
Print the smallest pretty integer.
2 3
4 2
5 7 6
25
8 8
1 2 3 4 5 6 7 8
8 7 6 5 4 3 2 1
1
In the first example 25, 46, 24567 are pretty, as well as many other integers. The smallest among them is 25. 42 and 24 are not pretty because they don't have digits from the second list.
In the second example all integers that have at least one digit different from 9 are pretty. It's obvious that the smallest among them is 1, because it's the smallest positive integer.
1 #include<cstdio> 2 #include<cstring> 3 #include<iostream> 4 #include<algorithm> 5 using namespace std; 6 7 const int INF=10; 8 int n,m; 9 10 int main() 11 { int a[10],b[10],visa[10],visb[10]; 12 cin>>n>>m; 13 memset(visa,0,sizeof(visa)); 14 memset(visb,0,sizeof(visb)); 15 for(int i=1;i<=n;i++){ cin>>a[i]; visa[a[i]]=1; } 16 for(int i=1;i<=m;i++){ cin>>b[i]; visb[b[i]]=1; } 17 sort(a+1,a+n+1); 18 sort(b+1,b+m+1); 19 int ans=INF; 20 for(int i=1;i<=9;i++) if(visa[i]&&visb[i]){ ans=i; break; } 21 if(ans!=INF) cout<<ans<<endl; 22 else{ 23 if(a[1]<b[1]) printf("%d%d ",a[1],b[1]); 24 else printf("%d%d ",b[1],a[1]); 25 } 26 }
B. Maximum of Maximums of Minimums
You are given an array a1, a2, ..., an consisting of n integers, and an integer k. You have to split the array into exactly k non-empty subsegments. You'll then compute the minimum integer on each subsegment, and take the maximum integer over the k obtained minimums. What is the maximum possible integer you can get?
Definitions of subsegment and array splitting are given in notes.
The first line contains two integers n and k (1 ≤ k ≤ n ≤ 105) — the size of the array a and the number of subsegments you have to split the array to.
The second line contains n integers a1, a2, ..., an ( - 109 ≤ ai ≤ 109).
Print single integer — the maximum possible integer you can get if you split the array into k non-empty subsegments and take maximum of minimums on the subsegments.
5 2
1 2 3 4 5
5
5 1
-4 -5 -3 -2 -1
-5
A subsegment [l, r] (l ≤ r) of array a is the sequence al, al + 1, ..., ar.
Splitting of array a of n elements into k subsegments [l1, r1], [l2, r2], ..., [lk, rk] (l1 = 1, rk = n, li = ri - 1 + 1 for all i > 1) is k sequences (al1, ..., ar1), ..., (alk, ..., ark).
In the first example you should split the array into subsegments [1, 4] and [5, 5] that results in sequences (1, 2, 3, 4) and (5). The minimums are min(1, 2, 3, 4) = 1 and min(5) = 5. The resulting maximum is max(1, 5) = 5. It is obvious that you can't reach greater result.
In the second example the only option you have is to split the array into one subsegment [1, 5], that results in one sequence ( - 4, - 5, - 3, - 2, - 1). The only minimum is min( - 4, - 5, - 3, - 2, - 1) = - 5. The resulting maximum is - 5.
题解:分类讨论,k>=3的情况,只要把数组中最大值单独作为一个区间,那么就得到了ans.
1 #include<cstdio> 2 #include<cstring> 3 #include<iostream> 4 #include<algorithm> 5 using namespace std; 6 7 const int INF=2e9; 8 const int maxn=2e5; 9 10 int n,k; 11 int a[maxn]; 12 13 int main() 14 { cin>>n>>k; 15 int mi=INF,ma=-INF; //因为数据可能是负的! 16 for(int i=1;i<=n;i++){ 17 scanf("%d",&a[i]); 18 mi=min(mi,a[i]); 19 ma=max(ma,a[i]); 20 } 21 if(k==1) cout<<mi<<endl; 22 else if(k==2){ 23 int b[maxn],c[maxn],x=INF; 24 for(int i=1;i<=n;i++){ 25 x=min(x,a[i]); 26 b[i]=x; 27 } 28 x=INF; 29 for(int i=n;i>=1;i--){ 30 x=min(x,a[i]); 31 c[i]=x; 32 } 33 int ans=-INF; 34 for(int i=1;i<n;i++) ans=max(ans,max(b[i],c[i+1])); 35 cout<<ans<<endl; 36 } 37 else cout<<ma<<endl; 38 }
C. Maximum splitting
You are given several queries. In the i-th query you are given a single positive integer ni. You are to represent ni as a sum of maximum possible number of composite summands and print this maximum number, or print -1, if there are no such splittings.
An integer greater than 1 is composite, if it is not prime, i.e. if it has positive divisors not equal to 1 and the integer itself.
The first line contains single integer q (1 ≤ q ≤ 105) — the number of queries.
q lines follow. The (i + 1)-th line contains single integer ni (1 ≤ ni ≤ 109) — the i-th query.
For each query print the maximum possible number of summands in a valid splitting to composite summands, or -1, if there are no such splittings.
1
12
3
2
6
8
1
2
3
1
2
3
-1
-1
-1
12 = 4 + 4 + 4 = 4 + 8 = 6 + 6 = 12, but the first splitting has the maximum possible number of summands.
8 = 4 + 4, 6 can't be split into several composite summands.
1, 2, 3 are less than any composite number, so they do not have valid splittings
题意:问一个数最多能用几个合数表示
题解:如果是偶数,那么它能表示成 n=k*2(k个2相乘),答案就是k/2,即只由4和6组成。如果是奇数,那么它能表示成 n=k*2+1,合数由4,6,9组成,所以要分类讨论
1 #include<bits/stdc++.h> 2 using namespace std; 3 4 int kase,n; 5 6 int main() 7 { scanf("%d",&kase); 8 while(kase--){ 9 scanf("%d",&n); 10 if(n==1||n==2||n==3){ cout<<"-1"<<endl; continue; } 11 if(n%2==0) cout<<n/2/2<<endl; 12 else{ 13 int ans=n/2-4; 14 if(ans==0) cout<<"1"<<endl; 15 else if(ans<0||ans==1) cout<<"-1"<<endl; 16 else cout<<ans/2+1<<endl; //别忘了加1(即合数9)!!! 17 } 18 } 19 return 0; 20 }
D. Something with XOR Queries
This is an interactive problem.
Jury has hidden a permutation p of integers from 0 to n - 1. You know only the length n. Remind that in permutation all integers are distinct.
Let b be the inverse permutation for p, i.e. pbi = i for all i. The only thing you can do is to ask xor of elements pi and bj, printing two indices i and j (not necessarily distinct). As a result of the query with indices i and j you'll get the value , where
denotes the xor operation. You can find the description of xor operation in notes.
Note that some permutations can remain indistinguishable from the hidden one, even if you make all possible n2 queries. You have to compute the number of permutations indistinguishable from the hidden one, and print one of such permutations, making no more than 2n queries.
The hidden permutation does not depend on your queries.
The first line contains single integer n (1 ≤ n ≤ 5000) — the length of the hidden permutation. You should read this integer first.
When your program is ready to print the answer, print three lines.
In the first line print "!".
In the second line print single integer answers_cnt — the number of permutations indistinguishable from the hidden one, including the hidden one.
In the third line print n integers p0, p1, ..., pn - 1 (0 ≤ pi < n, all pi should be distinct) — one of the permutations indistinguishable from the hidden one.
Your program should terminate after printing the answer.
To ask about xor of two elements, print a string "? i j", where i and j — are integers from 0 to n - 1 — the index of the permutation element and the index of the inverse permutation element you want to know the xor-sum for. After that print a line break and make flush operation.
After printing the query your program should read single integer — the value of .
For a permutation of length n your program should make no more than 2n queries about xor-sum. Note that printing answer doesn't count as a query. Note that you can't ask more than 2n questions. If you ask more than 2n questions or at least one incorrect question, your solution will get "Wrong answer".
If at some moment your program reads -1 as an answer, it should immediately exit (for example, by calling exit(0)). You will get "Wrong answer" in this case, it means that you asked more than 2n questions, or asked an invalid question. If you ignore this, you can get other verdicts since your program will continue to read from a closed stream.
Your solution will get "Idleness Limit Exceeded", if you don't print anything or forget to flush the output, including for the final answer .
To flush you can use (just after printing line break):
- fflush(stdout) in C++;
- System.out.flush() in Java;
- stdout.flush() in Python;
- flush(output) in Pascal;
- For other languages see the documentation.
Hacking
For hacking use the following format:
n
p0 p1 ... pn - 1
Contestant programs will not be able to see this input.
3
0
0
3
2
3
2
? 0 0
? 1 1
? 1 2
? 0 2
? 2 1
? 2 0
!
1
0 1 2
4
2
3
2
0
2
3
2
0
? 0 1
? 1 2
? 2 3
? 3 3
? 3 2
? 2 1
? 1 0
? 0 0
!
2
3 1 2 0
xor operation, or bitwise exclusive OR, is an operation performed over two integers, in which the i-th digit in binary representation of the result is equal to 1 if and only if exactly one of the two integers has the i-th digit in binary representation equal to 1. For more information, see here.
In the first example p = [0, 1, 2], thus b = [0, 1, 2], the values are correct for the given i, j. There are no other permutations that give the same answers for the given queries.
The answers for the queries are:
,
,
,
,
,
.
In the second example p = [3, 1, 2, 0], and b = [3, 1, 2, 0], the values match for all pairs i, j. But there is one more suitable permutation p = [0, 2, 1, 3], b = [0, 2, 1, 3] that matches all n2 possible queries as well. All other permutations do not match even the shown queries.
官方题解:
The statement: those and only those permutations whose answers to the queries (0, i) and (i, 0) for all i coincide with the answers given to the program, are suitable for all possible queries.
The proof: , which means that with the answers to the queries (0, i) and (i, 0) you can restore the answers to all other queries.
If we fix the value b0, then we can restore the whole permutation, since we know the answers to the queries (i, 0), and .
You can iterate through the value b0, restore the whole permutation, and if there were no contradictions in it (that is, every number from 0 to n - 1 occurs 1 time) and for all i values and
coincide with the answers given to the program, then this permutation is indistinguishable from the hidden permutation.
The answer is the number of such permutations, and one of such permutations.
E. Points, Lines and Ready-made Titles
You are given n distinct points on a plane with integral coordinates. For each point you can either draw a vertical line through it, draw a horizontal line through it, or do nothing.
You consider several coinciding straight lines as a single one. How many distinct pictures you can get? Print the answer modulo 109 + 7.
The first line contains single integer n (1 ≤ n ≤ 105) — the number of points.
n lines follow. The (i + 1)-th of these lines contains two integers xi, yi ( - 109 ≤ xi, yi ≤ 109) — coordinates of the i-th point.
It is guaranteed that all points are distinct.
Print the number of possible distinct pictures modulo 109 + 7.
4
1 1
1 2
2 1
2 2
16
2
-1 -1
0 1
9
In the first example there are two vertical and two horizontal lines passing through the points. You can get pictures with any subset of these lines. For example, you can get the picture containing all four lines in two ways (each segment represents a line containing it).
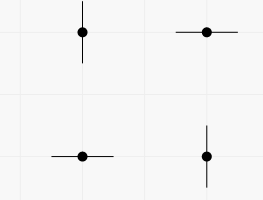
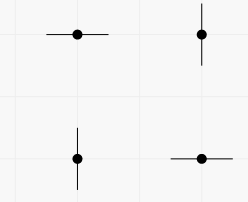
In the second example you can work with two points independently. The number of pictures is 32 = 9.
官方题解:
Let's build graph on points. Add edge from point to left, right, top and bottom neighbours (if such neigbour exist). Note that we can solve problem independently for each connected component and then print product of answer for components. So we can consider only connected graphs without loss of generality.
Let's define X as number of different x-coords, Y as number of different y-coords.
What if graph contains some cycle? Let's consider this cycle without immediate vertices (vertices that lie on the same line with previous and next vertices of cycle). Draw a line from each such vertex to the next vertex of cycle (and from last to the first). We got all lines that are corresponding to x-coords and y-coords of vertices of cycle. Let's prove by induction that we can got all such lines from the whole graph
Run depth-first search from vertices of cycle. Let we enter in some vertex that is not from cycle. It mush have at least one visited neighbour. By induction for graph consisting of visited vertices we can get all lines. So there is line from visited neighbour. Draw line in another direction and continue depth-first search. Sooner or later we will get all lines for the whole graph. Please note that intermediate vertices of cycle will be processed correctly too.
If we can get all lines the we can get all subsets of lines. Answer for cyclic graph is 2X + Y.
Now look at another case — acyclic graph or tree. We can prove that we can get any incomplete subset of lines.
Let's fix subset and some line not from this subset. Just draw this line without restriction. By similar induction as in cyclic graph case we can prove that we can get all lines (but fixed line doesn't exist really).
Now let's prove that it is impossible to take all lines. For graph consisting of only one vertex it is obvious. Else fix some leaf. We must draw a line which are not directed to any neigbour because it is the only way to draw this line. But now we have tree with less number of vertices. So our statement is correct by induction.
Answer for tree is 2X + Y - 1.
So the problem now is just about building graph on points and checking each component on having cycles.