springSecurity搭建
- 创建springsecurity配置文件
@Configuration
@EnableWebSecurity //开启springSecurity安全配置
public class SpringSecurityConfiguration extends WebSecurityConfigurerAdapter {
}
- 重写
protected void configure(HttpSecurity http)
方法 下面是该方法的默认配置:
- 自定义重写
protected void configure(HttpSecurity http)
方法
@Configuration
@EnableWebSecurity
public class SpringSecurityConfiguration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests() //请求权限认证
.antMatchers("/index.jsp") //使用ANT风格匹配路径
.permitAll() //允许访问所有
.anyRequest() //其他请求
.authenticated(); //都不允许访问
}
}
当访问出来index.jsp的其他页面时会出现如下错误 403
指定登录页 过滤请求
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests() //请求权限认证
.antMatchers("/index.jsp") //匹配路径
.permitAll() //允许访问所有
.anyRequest() //其他请求
.authenticated()//都不允许访问
.and()
.formLogin() //启动表单登录
// .loginPage("admin-login.jsp") // 指定登录页面
//loginProcessingUrl指定登录时的请求路径 不设置默认为 admin-login.jsp 设置后为 "admin/do/login.html"
.loginProcessingUrl("admin/do/login.html") //指定登录请求的路径
.permitAll(); //允许所有用户访问
}
配置基于内存存储的用户
- 前端页面 必须添加 ——csrf防止跨站请求伪造
如果不加会产生错误
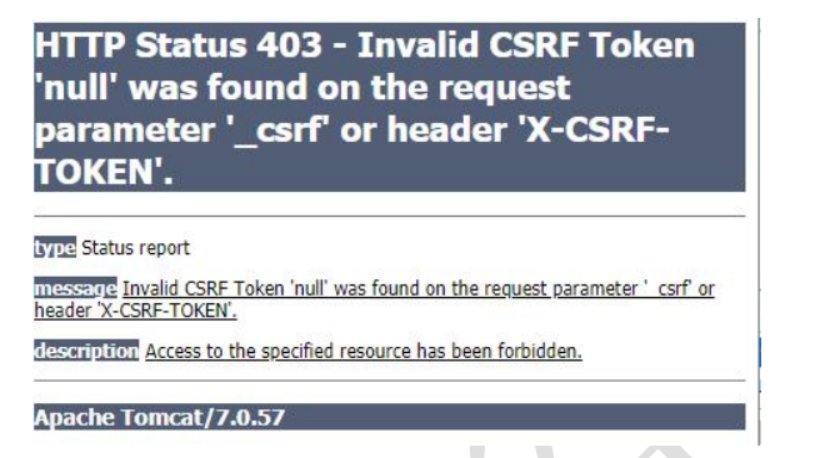
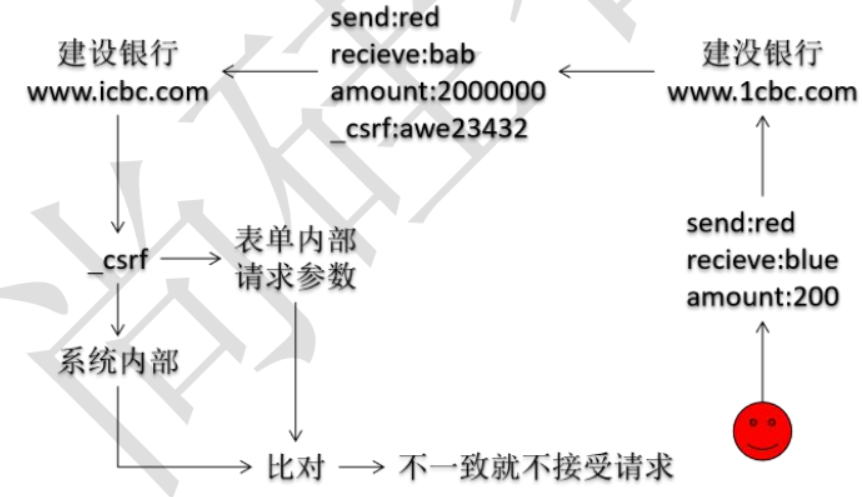
@Override
protected void configure(AuthenticationManagerBuilder builder) throws Exception {
builder
.inMemoryAuthentication() // 在内存中完成账号、密码的检查
.withUser("tom") // 指定账号
.password("123123") // 指定密码
.roles("ADMIN") // 指定当前用户的角色
.and()
.withUser("jerry") // 指定账号
.password("123123") // 指定密码
.authorities("UPDATE") // 指定当前用户的权限
;
}
@Override
protected void configure(HttpSecurity security) throws Exception {
security
.authorizeRequests() // 对请求进行授权
.antMatchers("/index.jsp") // 针对/index.jsp路径进行授权
.permitAll() // 可以无条件访问
.antMatchers("/layui/**") // 针对/layui目录下所有资源进行授权
.permitAll() // 可以无条件访问
.and()
.authorizeRequests() // 对请求进行授权
.anyRequest() // 任意请求
.authenticated() // 需要登录以后才可以访问
.and()
.formLogin() // 使用表单形式登录
// 关于loginPage()方法的特殊说明
// 指定登录页的同时会影响到:“提交登录表单的地址”、“退出登录地址”、“登录失败地址”
// /index.jsp GET - the login form 去登录页面
// /index.jsp POST - process the credentials and if valid authenticate the user 提交登录表单
// /index.jsp?error GET - redirect here for failed authentication attempts 登录失败
// /index.jsp?logout GET - redirect here after successfully logging out 退出登录
.loginPage("/index.jsp") // 指定登录页面(如果没有指定会访问SpringSecurity自带的登录页)
// loginProcessingUrl()方法指定了登录地址,就会覆盖loginPage()方法中设置的默认值/index.jsp POST
.loginProcessingUrl("/do/login.html") // 指定提交登录表单的地址
.usernameParameter("loginAcct") // 定制登录账号的请求参数名
.passwordParameter("userPswd") // 定制登录密码的请求参数名
.defaultSuccessUrl("/main.html") // 登录成功后前往的地址
;
}
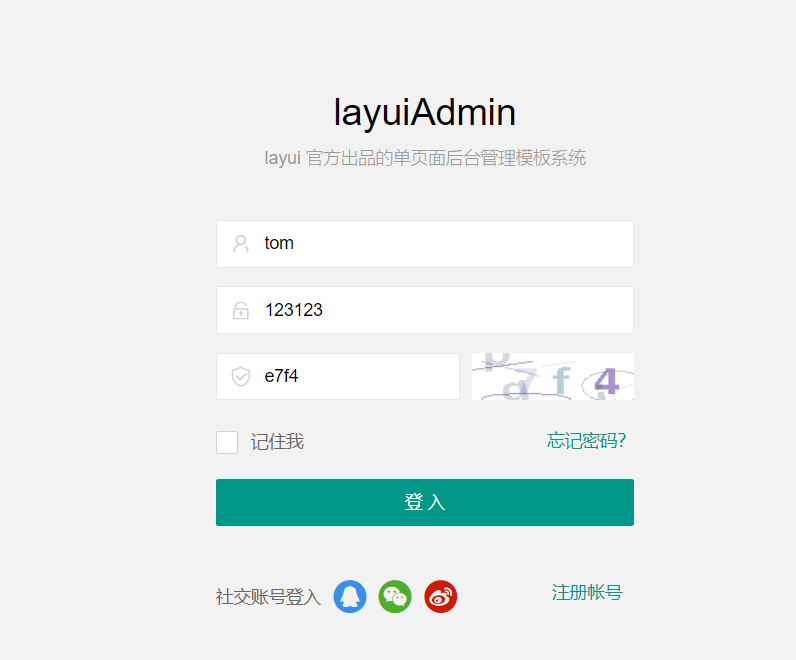
- 添加退出功能
.and()
.logout() //添加推出的登录功能
.logoutUrl("/logout") //推出登录请求路径
.logoutSuccessUrl("/index.jsp") //添加推出成功后跳转的路径
如果没有关闭csrf功能而要退出用户的话 必须要用post请求 可以进行如下转换
- 关闭csrf功能
.and()
.csrf()
.disable()
基于角色和权限的认证
@Override
protected void configure(AuthenticationManagerBuilder builder) throws Exception {
builder
.inMemoryAuthentication() // 在内存中完成账号、密码的检查
.withUser("tom") // 指定账号
.password("123123") // 指定密码
.roles("ADMIN") // 指定当前用户的角色
.and()
.withUser("jerry") // 指定账号
.password("123123") // 指定密码
.authorities("UPDATE") // 指定当前用户的权限
;
}
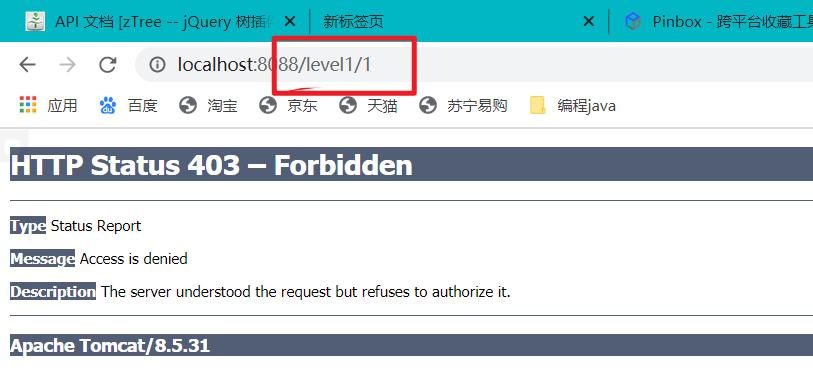
添加太极角色后进行测试
访问成功
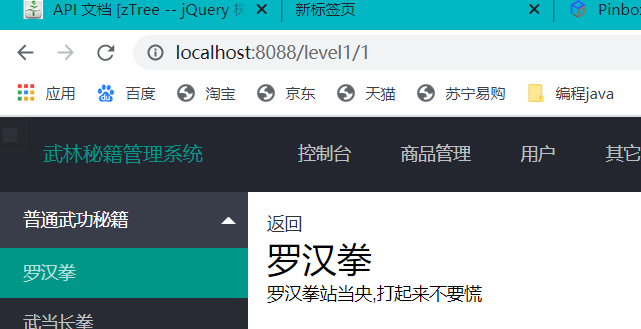
角色和权限区别
角色自动带有前缀ROLE_
权限没有前缀
springSecurity记住我
基于本地存储的cookie
- 前端
基于数据库存储的cookie
- 在xml配置文件中添加数据源
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="username" value="root"/>
<property name="password" value="root"/>
<property name="url" value="jdbc:mysql://localhost:3306/security"/>
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
</bean>
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"/>
</bean>
- 重写继承
JdbcTokenRepositoryImpl
的类JdbcTokenRepositoryService
public class JdbcTokenRepositoryService extends JdbcTokenRepositoryImpl {
public static final String CREATE_TABLE_SQL = "create table if not exists persistent_logins (username varchar(64) not null, series varchar(64) primary key, token varchar(64) not null, last_used timestamp not null)";
private boolean createTableOnStartup;
@Override
public void initDao() {
if (createTableOnStartup) {
getJdbcTemplate().execute(CREATE_TABLE_SQL);
}
}
@Override
public void setCreateTableOnStartup(boolean createTableOnStartup) {
this.createTableOnStartup=createTableOnStartup;
}
}
-
-
这里可以查看
JdbcTokenRepositoryImpl
类的源码 发现他可以自动创建出具库
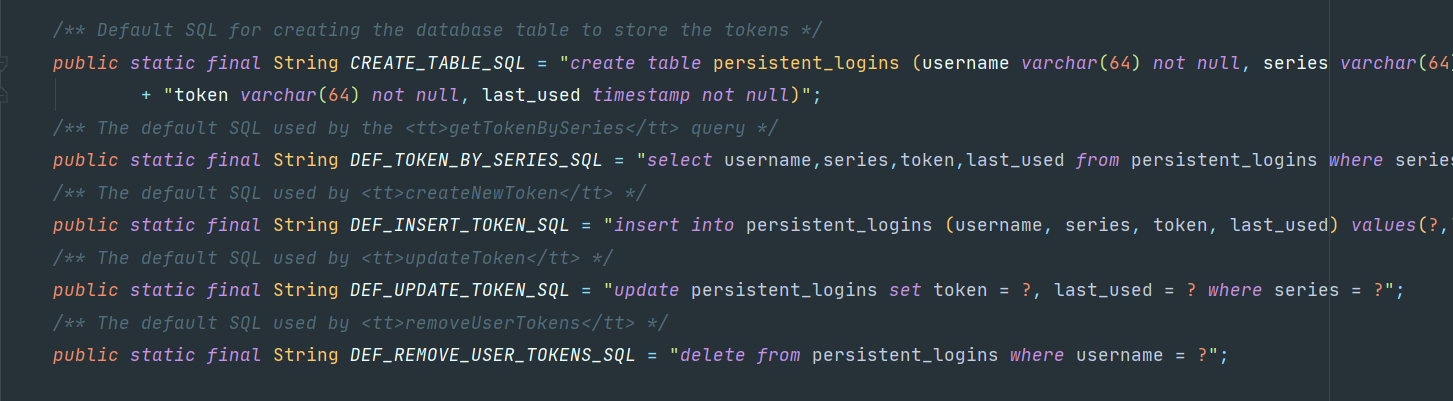
因为该类的初始化创建方法是protected标记的 所以必须继承他 或者重写为public才可以使用
- 重新访问 查看数据库
成功!!!