InputStream & Reader
-
InputStream(字节流),如下是InputStream的层次结构:
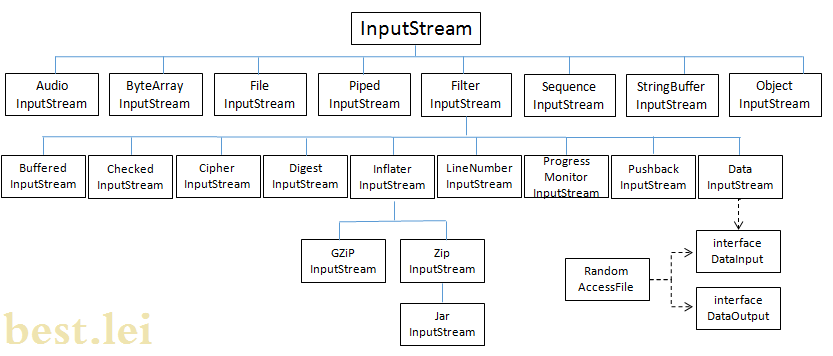
- AudioInputStream:音频输入流类,该方法可以:
- 从外部音频文件、流或 URL 获得音频输入流
- 从音频输入流写入外部文件
- 将音频输入流转换为不同的音频格式
AudioSystem
类包括许多操作 AudioInputStream
对象的方法:
- 播放wav格式的文件代码如下:
public class audioInputStream {
public static void playWAV(){
try {
AudioInputStream stream = AudioSystem.getAudioInputStream(new File("SourceFile/1.wav"));
byte[] samples = getSamples(stream); //将音频转化为字节数组
InputStream in = new ByteArrayInputStream(samples);
play(in,stream.getFormat()); //播放音频文件
} catch (UnsupportedAudioFileException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private static byte[] getSamples(AudioInputStream stream){
int length = (int) (stream.getFrameLength()*stream.getFormat().getFrameSize());
byte[] samples = new byte[length];
DataInputStream in = new DataInputStream(stream);
try {
in.readFully(samples);
System.out.println(length);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return samples;
}
private static void play(InputStream stream, AudioFormat format){
int bufferSize = format.getFrameSize()* Math.round(format.getSampleRate()/10);
byte[] buffer = new byte[bufferSize];
DataLine.Info info = new DataLine.Info(SourceDataLine.class, format);
try {
SourceDataLine line = (SourceDataLine) AudioSystem.getLine(info);
line.open(format, bufferSize);
line.start();
int numBytesRead = 0;
while(numBytesRead != -1){
numBytesRead = stream.read(buffer, 0, buffer.length);
if(numBytesRead != -1){
line.write(buffer, 0, numBytesRead);
//System.out.println(numBytesRead);
}
}
line.drain();
line.close();
} catch (LineUnavailableException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
- ByteArrayInputStream:流的来源并不一定是文件,也可以是内存中的一块空间,例如一个字节数组。ByteArrayInputStream就是将字节数组当作流输入来源的类。
- new ByteArrayInputStream(byte[] buf, int offset, int length)
- new ByteArrayInputStream(byte[] buf)
- FileInputStream:从文件系统或者终端获取输入信息,构造函数如下:
- new FileInputStream(File file)
- new FileInputStream(FileDescriptor fdObj)
- new FileInputStream(String name)
try {
FileInputStream fis = new FileInputStream("SourceFile/employee");
try {
byte[] bytes = new byte[fis.available()];
fis.read(bytes);
fis.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
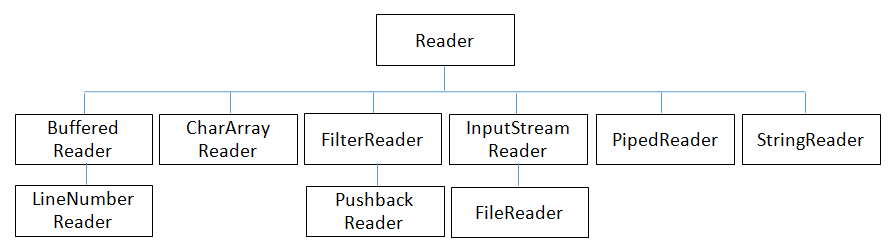
- BufferedReader:字符读入,默认拥有8192字符的缓冲区,当BufferedReader在读取文本文件时,会先尽量从文件中读入字符数据并置入缓冲区,而之后若使用read()方法,会先从缓冲区中进行读取。如果缓冲区数据不足,才会再从文件中读取。
- 构造方法有两个,size表示设置缓冲区大小,默认为8192:
- new BufferedReader(Reader in)
- new BufferedReader(Reader in, int size)
-
//System.in是一个位流,为了转换为字符流,可使用InputStreamReader为其进行字符转换,
//然后再使用BufferedReader为其增加缓冲功能。
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String content = null;
try {
while(!(content = br.readLine()).equals("quit")){
System.out.println(content);
}
br.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
- CharArrayReader:从字符数组中读取信息
- 构造方法有两个:
- new CharArrayReader(char[] buf)
- new CharArrayReader(char[] buf, int offset, int length)
- 相关说明见CharArrayReader类链接。
- InputStreamReader:将字节流转换为字符流。是字节流通向字符流的桥梁。如果不指定字符集编码,该解码过程将使用平台默认的字符编码。
- 构造方法:
- new InputStreamReader(InputStream in)
- new InputStreamReader(InputStream in, Charset cs)
- new InputStreamReader(InputStream in, CharsetDecoder dec)
- new InputStreamReader(InputStream in, String charsetName)
- 相关说明见InputStreamReader类链接。
- StringReader:读入String字符串。
- 构造方法
- new StringReader(String str)
- 相关代码
-
StringReader sr = new StringReader("dsfasdfasdfasd");
char[] chars = new char[5]; //每次读取5个字符
int length = 0;
try {
while((length = sr.read(chars)) != -1){
String strRead = new String(chars, 0, length).toUpperCase();
System.out.println(strRead);
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}