因为SpringBoot就是为了实现没有配置文件,因此之前手动在Mybatis中配置的PageHelper现在需要重新配置,而且配置方式与之前的SSM框架中还是有点点区别。
首先需要在pom文件中加入
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>0.1.0</version>
</dependency>
然后在SpringBoot的配置文件application.yml中加入如下配置:
pagehelper:
helperDialect: sqlserver
reasonable: true
supportMethodsArguments: true
pageSizeZero: true
params: count=countSql
目前Pagehelper插件支持Oracle,Mysql,MariaDB,SQLite,Hsqldb,PostgreSQL六种数据库分页,不同数据库只需要修改helperDialect就行。
java代码中的使用如下:
PageHelper.startPage(page, rows);
List<Map> list = testService.find();
PageInfo<Map> pageInfo = new PageInfo<>(list);
第一行是设置页数和每页显示几条,插件会自动对接下来的sql语句加上分页方式。PageInfo中是分页的一些信息,包括总页数,当前页,总数据等。
访问数据库采用mybatis框架
1.添加pom文件依赖
<!-- spring mvc支持 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- springboot整合mybatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.1</version>
</dependency>
<!-- springboot分页插件 -->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.2</version>
</dependency>
<!-- 阿里巴巴druid数据库连接池 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.3</version>
</dependency>
<!-- mysql驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
2.配置application.yml
# 与mybatis整合
mybatis:
config-location: classpath:mybatis.xml
mapper-locations:
- classpath:mapper/*.xml
# 分页配置
pagehelper:
helper-dialect: mysql
reasonable: true
support-methods-arguments: true
params: count=countSql
3.service层中使用插件
package com.ahut.serviceImpl;
import java.util.List;
import javax.servlet.ServletContext;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.web.context.ContextLoader;
import com.ahut.entity.GoodsType;
import com.ahut.mapper.GoodsTypeMapper;
import com.ahut.service.GoodsTypeService;
import com.github.pagehelper.PageHelper;
/**
*
* @ClassName: GoodsTypeServiceImpl
* @Description: 商品类型业务逻辑处理
* @author cheng
* @date 2017年7月17日 上午10:04:31
*/
@Service
@Transactional(rollbackFor = { RuntimeException.class, Exception.class })
public class GoodsTypeServiceImpl implements GoodsTypeService {
// 数据访问
@Autowired
private GoodsTypeMapper typeDao;
/**
*
* @Title: getList
* @Description: 从数据库中获取所有商品类型列表
* @param pageNum 当前页
* @param pageSize 当前页面展示数目
* @return
* @throws Exception
*/
public List<GoodsType> getList(int pageNum, int pageSize) throws Exception {
//使用分页插件,核心代码就这一行
PageHelper.startPage(pageNum, pageSize);
// 获取
List<GoodsType> typeList = typeDao.getList();
return typeList;
}
}
4.controller层代码
package com.ahut.action;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.ahut.entity.GoodsType;
import com.ahut.service.GoodsTypeService;
/**
*
* @ClassName: GoodsTypeAction
* @Description: 商品类型控制层
* @author cheng
* @date 2017年7月17日 上午11:09:47
*/
@RestController // 等价于@Controller+@ResponseBody
public class GoodsTypeAction {
// 业务逻辑
@Autowired
private GoodsTypeService typeService;
/**
*
* @Title: getGoodsTypeList
* @Description: 获取商品类型列表
* @return
* @throws Exception
*/
@RequestMapping(value = "/getGoodsTypeList")
public List<GoodsType> getGoodsTypeList(int pageNum, int pageSize) throws Exception {
// 调用业务逻辑,返回数据
return typeService.getList(pageNum,pageSize);
}
}
5.测试
已知我数据库中有九条数据:
正常情况:
1.显示第一页或者第二页数据
请求url:
http://localhost:8080/getGoodsTypeList?pageNum=1&pageSize=4
- 1
返回数据:
[
{
"typeId": "708cc61c6a9811e796dee09467355fab",
"typeName": "全部",
"createTime": 1500258859000,
"updateTime": 1500621762000
},
{
"typeId": "98f8a04e6a9811e796dee09467355fab",
"typeName": "考研资料",
"createTime": 1500258927000,
"updateTime": null
},
{
"typeId": "b720c87f6a9811e796dee09467355fab",
"typeName": "交通工具",
"createTime": 1500258978000,
"updateTime": null
},
{
"typeId": "cbe3c2326a9811e796dee09467355fab",
"typeName": "生活用品",
"createTime": 1500259013000,
"updateTime": 1500626046000
}
]
2.显示最后一页
请求url:
http://localhost:8080/getGoodsTypeList?pageNum=3&pageSize=4
- 1
返回数据:
[
{
"typeId": "d992195f6df111e7bab4e09467355fab",
"typeName": "测试2改变了",
"createTime": 1501145516000,
"updateTime": 1500716178000
}
]
不正常情况:
1.显示的页数小于第一页(显示第一页数据)
pageNumber <= 0
请求url:
http://localhost:8080/getGoodsTypeList?pageNum=0&pageSize=4
- 1
返回数据:
[
{
"typeId": "708cc61c6a9811e796dee09467355fab",
"typeName": "全部",
"createTime": 1500258859000,
"updateTime": 1500621762000
},
{
"typeId": "98f8a04e6a9811e796dee09467355fab",
"typeName": "考研资料",
"createTime": 1500258927000,
"updateTime": null
},
{
"typeId": "b720c87f6a9811e796dee09467355fab",
"typeName": "交通工具",
"createTime": 1500258978000,
"updateTime": null
},
{
"typeId": "cbe3c2326a9811e796dee09467355fab",
"typeName": "生活用品",
"createTime": 1500259013000,
"updateTime": 1500626046000
}
]
结论:当请求页数小于第一页时,显示第一页数据
2.显示的页数大于最后一页(显示最后一页数据)
pageNum > 最后一页
请求url:
http://localhost:8080/getGoodsTypeList?pageNum=4&pageSize=4
- 1
返回数据:
[
{
"typeId": "d992195f6df111e7bab4e09467355fab",
"typeName": "测试2改变了",
"createTime": 1501145516000,
"updateTime": 1500716178000
}
Springboot整合pagehelper分页
一、添加依赖
在pom中添加依赖
<dependency> <groupId>com.github.pagehelper</groupId> <artifactId>pagehelper-spring-boot-starter</artifactId> <version>1.2.2</version> </dependency>
二、使用
网络上很多文章都会说需要在application.properties进行配置
其实完全不需要,默认的设置就已经满足大部分需要了
直接使用即可
@RequestMapping(value = "getApps.do") public String getApps(Apps apps) { PageHelper.startPage(apps.getPageNum(), apps.getPageSize()); ArrayList<Apps> appsList = appsService.getApps(apps); PageInfo<Apps> appsPageInfo = new PageInfo<>(appsList); return JSON.toJSONString(appsPageInfo); }
PageHelper.startPage(需要显示的第几个页面,每个页面显示的数量);
下一行紧跟查询语句,不可以写其他的,否则没有效果。
PageHelper.startPage(apps.getPageNum(), apps.getPageSize()); ArrayList<Apps> appsList = appsService.getApps(apps);
这样只起到了分页效果,对总页面数之类的没有详细信息
如果对页面数量等有需求,则需要加上下面这行
PageInfo<T> appsPageInfo = new PageInfo<>(appsList);
这样就满足了全部的分页要求
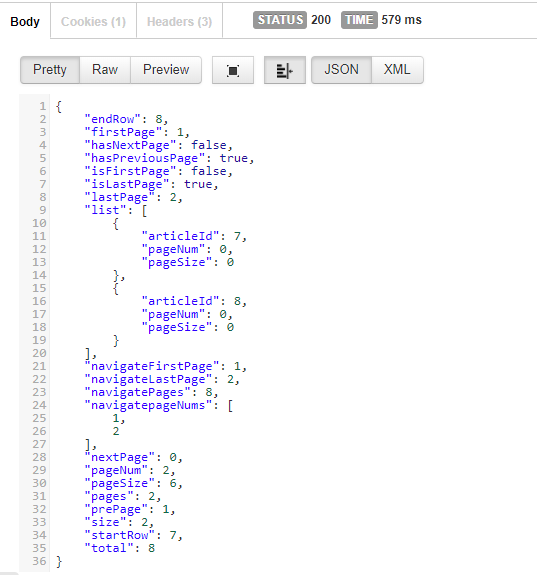