//异常:感冒,发烧,是可以治
//错误:癌症,艾滋,是不能治的,一旦发生错误,直接修改代码
public class Demo01 {
public static void main(String[] args) {
int[] arr={1,2,3,4,5};
int i=get(arr);
System.out.println(i);
//int[] arr=new int[999999999];
}
public static int get(int[] arr){
int i=arr[5]+1;
return i;
}
}

抛出异常throw
public class Demo02 {
public static void main(String[] args) throws Exception{
int[] arr={};
int i=get(arr);
System.out.println(i);
}
//throw new 异常类名()
//throws 声明异常
public static int get(int[] arr) throws Exception{
if(arr.length==0){
throw new Exception("你传入的数组为空的数组");
}
if(arr==null){
throw new Exception("你传入的数组为null");
}
int i=arr[arr.length-1];
return i;
}
}

public class Demo05 {
public static void main(String[] args) {
}
}
//如果父类方法抛异常,子类继承 如果子类也抛异常,抛的异常必须小于等于父类抛的异常
//如果父类方法没抛异常,子类不许抛异常
//如果父类方法没抛异常 子类继承方法里有抛异常的方法,只能用try catch
class Fu{
public void eat() throws Exception{
}
}
class Zi extends Fu{
}
捕获异常try…catch…finally
/*try{
* 可能会产生异常的语句
* }catch(可能会产生的异常名 变量名){
* 返回该类异常
* }finally{
* 一定会执行的代码
* }*/
public class Demo03 {
public static void main(String[] args){
int[] arr={};
try{
int i=get(arr);
System.out.println(i);
}catch(Exception ex){
System.out.println(ex);
}finally{
System.out.println("一定会执行的代码");
}
System.out.println("Hi");
}
//throw new 异常类名()
//throws 声明异常
public static int get(int[] arr) throws Exception{
if(arr.length==0){
throw new Exception("你传入的数组为空的数组");
}
if(arr==null){
throw new Exception("你传入的数组为null");
}
int i=arr[arr.length-1];
return i;
}
}
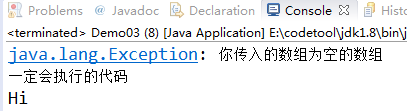
try…catch…finally异常处理的组合方式
/*try{
* 可能会产生异常的语句
* }catch(可能会产生的异常名 变量名){
* 返回该类异常
* }catch(可能会产生的异常名 变量名){
* 返回该类异常
* }finally{
* 一定会执行的代码
* }*/
public class Demo04 {
public static void main(String[] args){
int[] arr={};
try{
int i=get(arr);
System.out.println(i);
}catch(ArrayIndexOutOfBoundsException ex){
System.out.println(ex);
}catch(NullPointerException ex){
System.out.println(ex);
}finally{
System.out.println("一定会执行的代码");
}
System.out.println("Hi");
}
//throw new 异常类名()
//throws 声明异常
public static int get(int[] arr) throws ArrayIndexOutOfBoundsException,NullPointerException{
if(arr.length==0){
throw new ArrayIndexOutOfBoundsException("你传入的数组为空的数组");
}if(arr==null){
throw new NullPointerException("你传入的数组为null");
}
int i=arr[arr.length-1];
return i;
}
}

运行时期异常
运行时期异常的特点:
方法中抛出运行时期异常,方法定义中无需throws声明,调用者也无需处理此异常
运行时期异常一旦发生,需要程序人员修改源代码.
public class FuShu extends RuntimeException{
public FuShu(){
}
public FuShu(String str){
super(str);
}
}
异常中常用方法
public class Demo06 {
public static void main(String[] args) {
int[] arr={};
int i=0;
try{
i=get(arr);
System.out.println(i);
}catch(Exception ex){
//红字打印
ex.printStackTrace();
//异常简短描述
//String e=ex.getMessage();
//详细消息字符串
//String e=ex.toString();
//System.out.println(e);
}
}
public static int get(int[] arr) throws Exception{
if(arr.length==0){
throw new Exception("你传入的数组为空的数组");
}
int i=arr[arr.length-1];
return i;
}
}
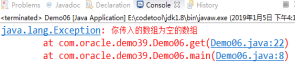
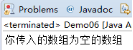
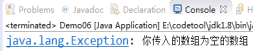
自定义异常
public class Demo07 {
public static void main(String[] args) {
int[] arr={-1,2,3};
double score=avg(arr);
System.out.println(score);
}
public static double avg(int[] arr){
int sum=0;
for(int i:arr){
if(i<0){
throw new FuShu("你传入的数组中有负数"+i);
}
sum+=i;
}
return sum/arr.length;
}
}
